Today I did testing with something I’ve been working on over this past week. I have been working with Python Turtle Graphics and have been trying to make the Sierpisnki triangle with rainbow colors and I finally, finally, finally have found the proper algorithm, so here it is, the code for it alongside an image of the successful small-scale test
from turtle import *
import time
# Set the color mode to RGB
colormode(255)
# Set the background color to black
bgcolor(0, 0, 0)
# Function to generate rainbow colors
def rainbow_color_interpolation(num_colors):
colors = []
for i in range(num_colors):
# Calculate the hue value
hue = i / num_colors * 360
# Convert HSV to RGB
rgb = hsv_to_rgb(hue / 360.0, 1.0, 1.0)
colors.append((int(rgb[0] * 255), int(rgb[1] * 255), int(rgb[2] * 255)))
return colors
def hsv_to_rgb(h, s, v):
if s == 0.0: # Achromatic (grey)
return (v, v, v)
i = int(h * 6.0) # Assume h is [0, 1)
f = (h * 6.0) - i
p = v * (1.0 - s)
q = v * (1.0 - f * s)
t = v * (1.0 - (1.0 - f) * s)
i = i % 6
if i == 0:
return (v, t, p)
elif i == 1:
return (q, v, p)
elif i == 2:
return (p, v, t)
elif i == 3:
return (p, q, v)
elif i == 4:
return (t, p, v)
elif i == 5:
return (v, p, q)
# Setup turtle
speed(0)
width(1)
count = 0
def draw_triangle(length):
global count
# Use interpolated colors for the triangle
color(rainbow_colors[count % len(rainbow_colors)])
count += 1
forward(length)
right(120)
color(rainbow_colors[count % len(rainbow_colors)])
count += 1
forward(length)
right(120)
def draw_sierpinski(length):
draw_triangle(length)
if length > 10:
draw_sierpinski(length // 2)
draw_triangle(length)
if length > 10:
draw_sierpinski(length // 2)
draw_triangle(length)
if length > 10:
draw_sierpinski(length // 2)
draw_triangle(length)
if length > 10:
draw_sierpinski(length // 2)
draw_triangle(length)
if length >= 10:
draw_sierpinski(length // 2)
draw_triangle(length)
# Generate rainbow colors
num_colors = 6 # You can adjust this number for more or fewer colors
rainbow_colors = rainbow_color_interpolation(num_colors)
# Main drawing loop
while True:
for color_value in rainbow_colors:
color(color_value) # Set the turtle color
draw_sierpinski(120)
# Pause for a moment before the next drawing
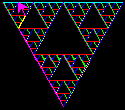
And an image from Pennwest/Edinboro’s Chaos Game Website (This Website is super cool check it out at prodweb2.cis.pennwest.edu/sierpinski/):
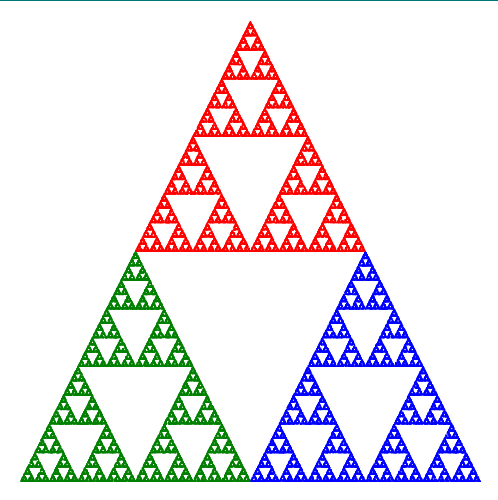
As can be seen, the program successfully makes a Sierpinski’s Triangle despite taking a very long time even for just this small one, I have tried to tweak the numbers to fix this issue, but I haven’t yet found any values that would make it work properly and make a true Sierpinski triangle as I have found can be made. I will now show a larger scale test with a length increase of 100:
As you can see, it takes a long time for the turtle to do anything because it keeps tracing back over itself because of the way that the function recursion is working, and I can’t lower the amount of function recursions, or it does not make a proper Sierpinski triangle. Here is the picture of the completed product of the test with 220 length, which took me over an hour to complete.
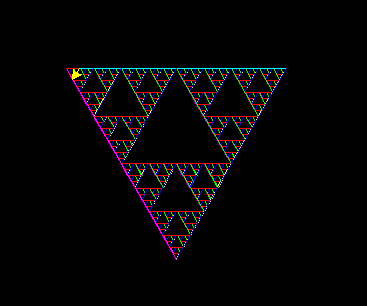
And it completed it, it took from 10:35-11:37 to complete. Honestly, I tumbled across the number of recursions to make it work but, I am glad it did, because now I have a way to make any computer crash just by drawing too big of a triangle which did actually happen to me, I bluescreened off a triangle.
Leave a Reply