**This continues from the 1/19/25 blog post**
KEYBOARD INPUTS
- Now that we know how to speed the object up and down, let’s add some keyboard inputs.
- in “def_update()”, we’re gonna add a module called “held_keys”. This will let you use any key you want on your keyboard (or your mouse keys) to control objects and entities on the screen.
def update():
if held_keys['a']:
test_square.x -= 1 * time.dt
- You’ll also need to indent “test_square” for it to work (as shown above).
- Now if we run it, we’ll be able to move the square by pressing “A” on the keyboard.
ADDING IMAGES IN URSINA
- We now have our object and a way to move it. Now let’s add a picture. The picture will also need to be and entity (and will also be a square, or “quad” in this case).
- We’re gonna call this entity “sans” (the short skeleton guy from Undertale that is probably the hardest character to go against in a Genocide run.)
sans = Entity(model = 'quad', texture = sans_texture)
- Before we can see sans on the screen, we must make a texture. This will be simply called ‘sans_texture”, which will need an image (this will just be called “Sans.png”. You can store this in a folder if you want.)
sans_texture = load_texture('assets/Sans.png')
- NOW we can run the program and see sans staring into your soul.
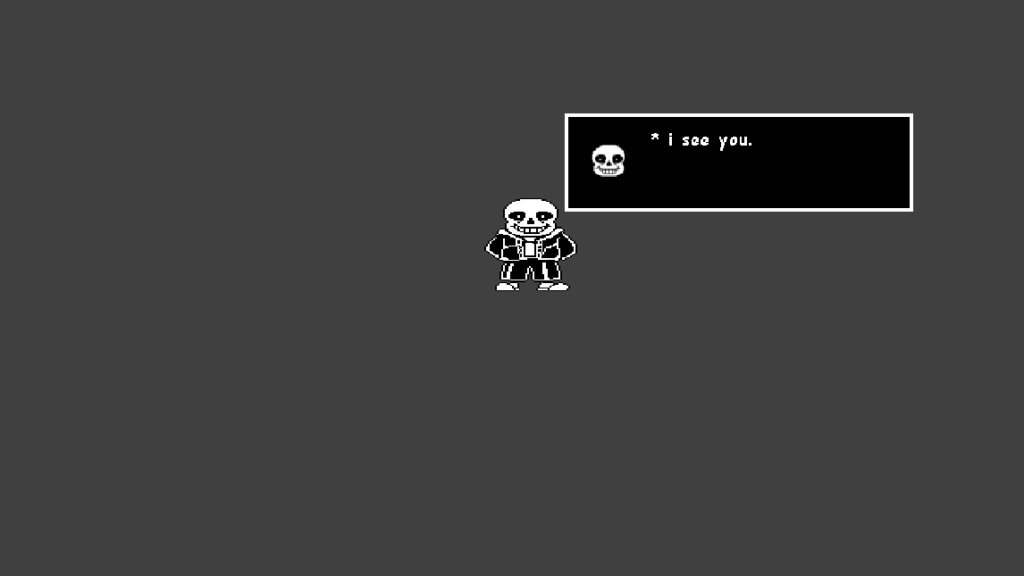
*You also could just pass the “assets/Sans.png” instead of making a variable for it, and it’ll generate the same thing (unless you’re an overachiever and like doing things the difficult way).
sans = Entity(model = 'quad', texture = 'assets/Sans.png') #<- like this
CREATING 3D OBJECTS IN URSINA
- Now, in an actual game, you’d want more control over all these things, so the code above is usually made to create simple images. To create more complex images, you’ll need to create a class for it, which we will do now.
- Let’s make a cube. For this, we’ll make a class called “Test_cube” (I know, again, so creative). In it, we need it to inherit from something, which well be called “Entity”.
class Test_cube(Entity):
- Inside the class, we need and “def __init__()” method so we can call our variables (there’s a reason why we need this, but I forgot why and don’t feel like looking it up). We’ll also need “super().__init__()” in order to call some attributes for the cube (model, color, texture, etc…).
class Test_cube(Entity):
def __init__(self):
super().__init__(
model = 'cube',
color = color.white,
texture = 'white_cube'
)
*Put this towards the top of your program btw (or don’t. I’m not your boss).
- Now, we just need to call it, and it’ll show up on the screen when we run the program.
test_cube = Test_cube()
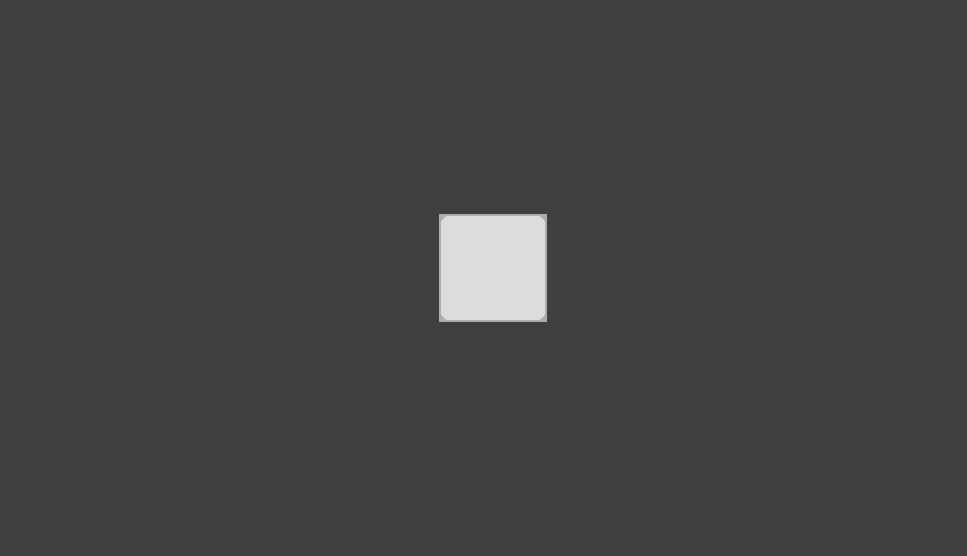
- It doesn’t look 3D, but that’s because we haven’t added any rotation yet. In order to do that, we’ll add a rotation argument.
class Test_cube(Entity):
def __init__(self):
super().__init__(
model = 'cube',
color = color.white,
texture = 'white_cube',
rotation = Vec3(45, 45, 45)
)
- If we run the code, the cube should be rotated 45°.
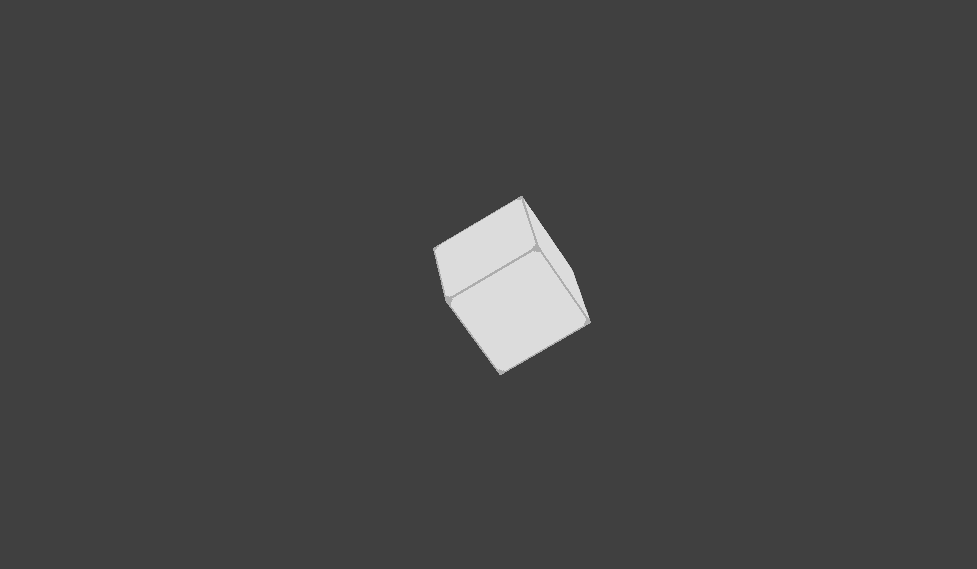
*SO flipping cool.
- You can also add images as a texture to a 3D object, like you can with a 2D object.
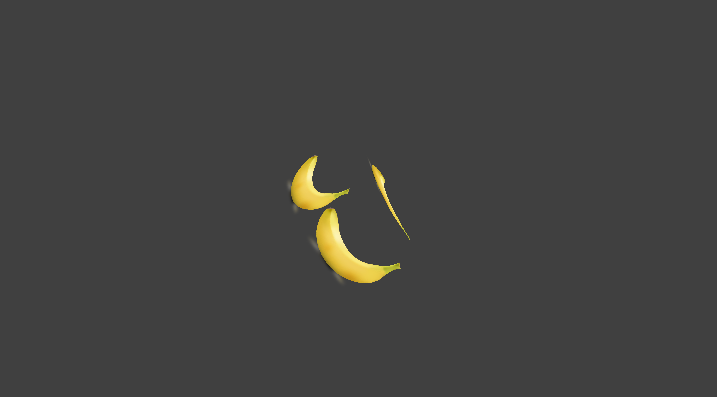
*Only terminally online people will get this reference
CREATING BUTTONS IN URSINA
- We’ve covered a lot of stuff in this, but before we make an actual game (yes, this is just the basics of Ursina), we need to create a button. Buttons work slightly differently (not really).
- To create a button, you have to create a class. This’ll be called “Test_button”. This will inherit from the class “Button” itself (which is already in Ursina, we don’t have to make a class called “Button”, same with “Test_cube”).
- We will also add a “def__init__” method and a “super().__init__()” method in the class (where we’ll add some attributes in it too).
class Test_button(Button):
def __init__(self):
super().__init__(
model = 'cube',
texture = 'brick',
color = color.orange
)
NOTE: MAKE SURE TO COMMENT OUT “test_cube = Test_cube” OR ELSE IT’LL BE IN THE WAY!!!
If we run it, we’ll have a GIANT orange brick button created at the center of the screen.
test_button = Test_button()
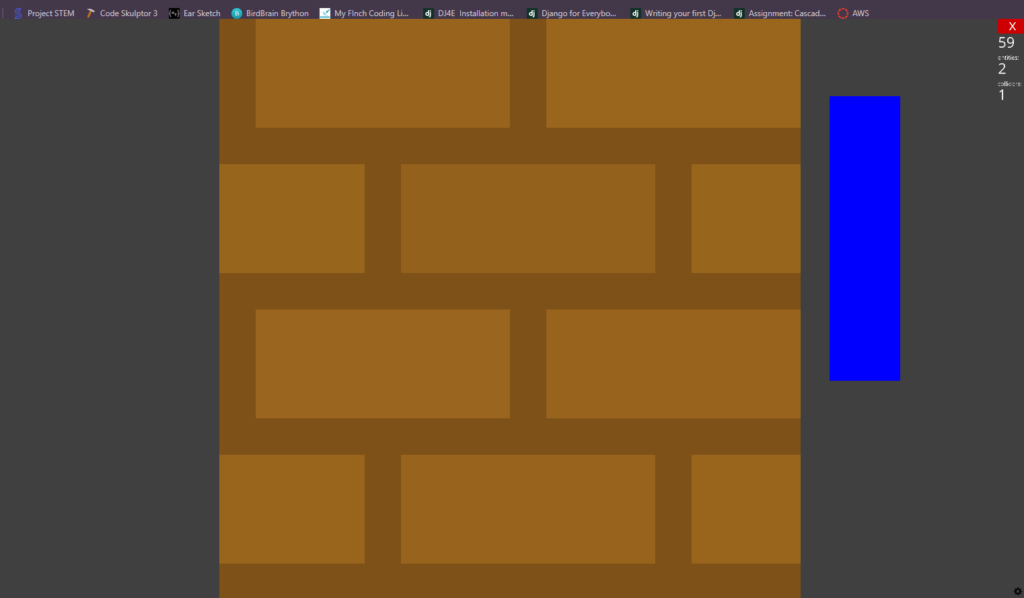
*You’re able to press it, but it doesn’t do anything except light up and stuff.
- Now, the problem with this is that the button is significantly larger than “test_cube”. In order to fix this, we need the “parent” argument for the button. In the parent argument, we need the “scene”, which is the game scene itself (I tried to find some information on what the parent argument doe and what a scene is, but I was left empty-handed…)
class Test_button(Button):
def __init__(self):
super().__init__(
parent= scene,
model = 'cube',
texture = 'brick',
color = color.orange
)
- If we run it, the button won’t absolutely massive anymore (massive?).
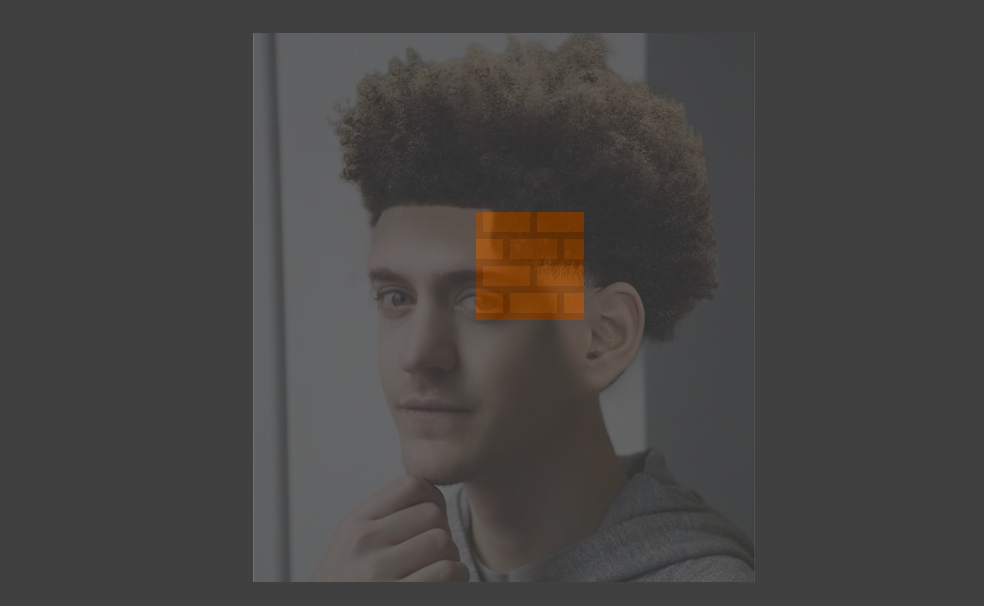
*This joke is so stupid.