This is a combination of what I did yesterday AND today. This blog post is gonna be big…
Made an offset to the camera!!!
class YSortCameraGroup(pygame.sprite.Group):
def __init__(self):
# general setup
super().__init__()
self.display_surface = pygame.display.get_surface()
self.half_width = self.display_surface.get_size()[0] // 2
self.half_height = self.display_surface.get_size()[1] // 2
self.offset = pygame.math.Vector2()
def custom_draw(self, player):
# getting the offset
self.offset.x = player.rect.centerx - self.half_width
self.offset.y = player.rect.centery - self.half_height
for sprite in self.sprites():
offset_pos = sprite.rect.topleft - self.offset
self.display_surface.blit(sprite.image, offset_pos)
The code creates where the camera will be “placed” on the screen. It then puts an offset – what kind of angle or area the camera – will be “placed”.
RESULT:
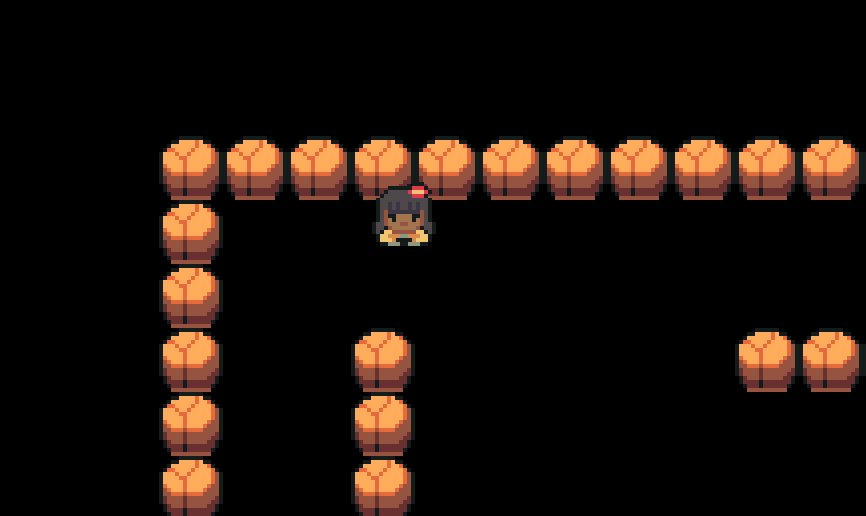
This only offsets the camera, but doesn’t make the camera move with the player… yet.
Added an overlap for collisions (and also made the camera move with the player).

changes the size of the rectangle (the collision) and makes it smaller or larger. The parameters “x” and “y” are the numbers that will increase or decrease the size of the hitbox.
“x” will be zero and “y” will be a certain number (-26 to be specific)
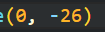
Also replaced (almost) every rect with hitbox.
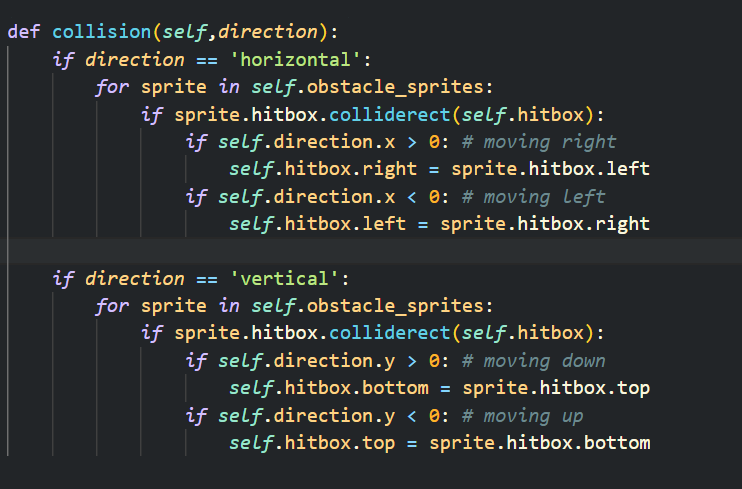
Also changed the for loop in YSortCameraGroup class so that we have overlap on ALL rocks.
BEFORE:
for sprite in self.sprites():
offset_pos = sprite.rect.topleft - self.offset
self.display_surface.blit(sprite.image, offset_pos)
AFTER:
for sprite in sorted(self.sprites(),
key = lambda sprite: sprite.rect.centery):
offset_pos = sprite.rect.topleft - self.offset
self.display_surface.blit(sprite.image, offset_pos
RESULT:
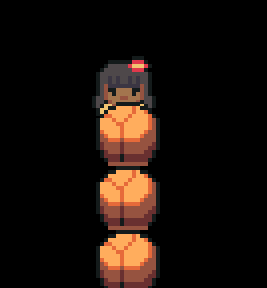
Character overlaping behind the rock.
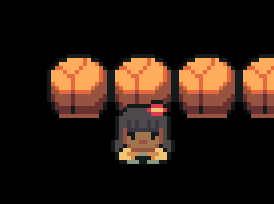
Character overlaping in front of the rock.
NOTE: I will add a short clip of the camera following the player, just not now (can’t screen record if the settings are blocked ˙◠˙).
PUTTING THE FLOOR MAP ON THE SCREEN (and putting the player in the middle of the map)!!!
Created the floor map of the game :
self.floor_surf = pygame.image.load('graphics//tilemap//ground.png')
self.floor_rect = self.floor_surf.get_rect(topleft = (0,0))
And drew the floor map.
floor_offset_pos = self.floor_rect.topleft - self.offset
self.display_surface.blit(self.floor_surf, floor_offset_pos)
Put the player in the middle of the map (idk if the numbers are temporary or not :/)
self.player = Player((2000,1430),
[self.visible_sprites],self.obstacle_sprites)
RESULT:
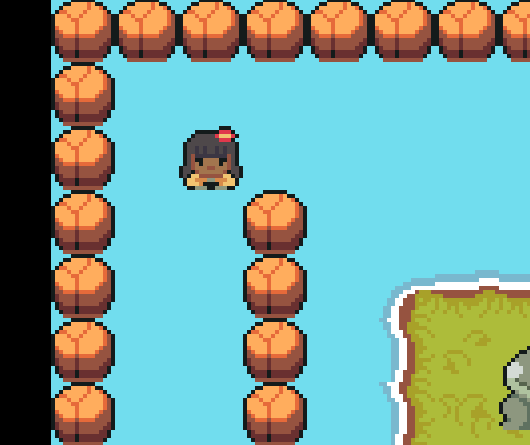
This was before I added that code to center the player.
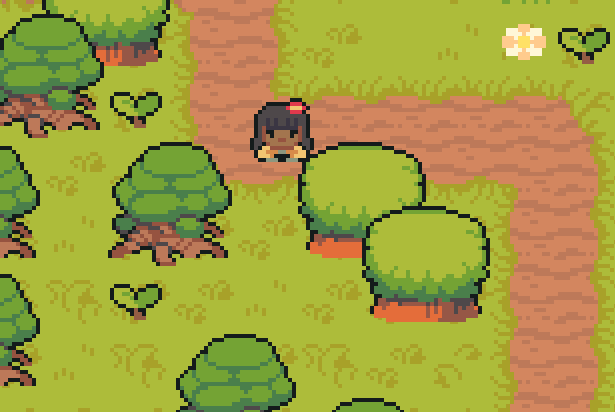
ANNND this is after!!! There are no collisions on the plants or anything yet.
Sweet! Keep up the good work!