*This is a somewhat short program. I’ll resume with making minecraft in Python next time).
First, create the definitions for the calculator.
import tkinter as tk
calculation = ""
def add_to_calculation(symbol):
global calculation
calculation += str(symbol)
text_result.delete(1.0, "end")
text_result.insert(1.0, calculation)
def add_to_calculation will add a number (1,2,3…) or a symbol (*, +, -…) to calculation.
def evaluate_calculation():
global calculation
try:
result = str(eval(calculation))
calculation = ""
text_result.delete(1.0, "end")
text_result.insert( 1.0, calculation)
except:
clear_field()
text_result.insert(1.0, "error")
def evaluate_calculation will solve the calculation the user has inputted (EX. will return 42 if the user inputted 6*7).
def clear_field():
global calculation
calculation = ""
text_result.delete(1.0, "end")
def clear_field will clear any numbers or symobls inputted into calculation, giving it a clean slate, and allowing another calculation to be inputted.
Next, create the window, and its geometry.
window = tk.Tk()
window.geometry("300x275")
Then, create a text area for numbers and symbols to be inputted.
text_result = tk.Text(window, height= 2, width= 16, font=("Arial", 24))
text_result.grid(columnspan=5)
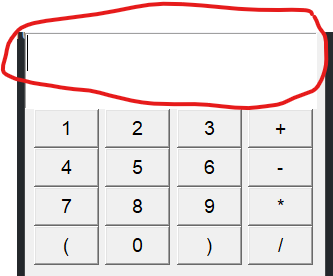
Now, create the buttons and add them onto the GUI.
btn_1 = tk.Button(window, text='1', command=lambda: add_to_calculation(1), width=5, font =("Arial", 14))
btn_1.grid(row=2, column=1)
btn_2 = tk.Button(window, text='2', command=lambda: add_to_calculation(2), width=5, font =("Arial", 14))
btn_2.grid(row=2, column=2)
btn_3 = tk.Button(window, text='3', command=lambda: add_to_calculation(3), width=5, font =("Arial", 14))
btn_3.grid(row=2, column=3)
btn_4 = tk.Button(window, text='4', command=lambda: add_to_calculation(4), width=5, font =("Arial", 14))
btn_4.grid(row=3, column=1)
btn_5 = tk.Button(window, text='5', command=lambda: add_to_calculation(5), width=5, font =("Arial", 14))
btn_5.grid(row=3, column=2)
btn_6 = tk.Button(window, text='6', command=lambda: add_to_calculation(6), width=5, font =("Arial", 14))
btn_6.grid(row=3, column=3)
btn_7 = tk.Button(window, text='7', command=lambda: add_to_calculation(7), width=5, font =("Arial", 14))
btn_7.grid(row=4, column=1)
btn_8 = tk.Button(window, text='8', command=lambda: add_to_calculation(8), width=5, font =("Arial", 14))
btn_8.grid(row=4, column=2)
btn_9 = tk.Button(window, text='9', command=lambda: add_to_calculation(9), width=5, font =("Arial", 14))
btn_9.grid(row=4, column=3)
btn_0 = tk.Button(window, text='0', command=lambda: add_to_calculation(0), width=5, font =("Arial", 14))
btn_0.grid(row=5, column=2)
btn_plus = tk.Button(window, text='+', command=lambda: add_to_calculation("+"), width=5, font =("Arial", 14))
btn_plus.grid(row=2, column=4)
btn_minus = tk.Button(window, text='-', command=lambda: add_to_calculation("-"), width=5, font =("Arial", 14))
btn_minus.grid(row=3, column=4)
btn_mult = tk.Button(window, text='*', command=lambda: add_to_calculation("*"), width=5, font =("Arial", 14))
btn_mult.grid(row=4, column=4)
btn_div = tk.Button(window, text='/', command=lambda: add_to_calculation("/"), width=5, font =("Arial", 14))
btn_div.grid(row=5, column=4)
btn_open_peren = tk.Button(window, text='(', command=lambda: add_to_calculation("("), width=5, font =("Arial", 14))
btn_open_peren.grid(row=5, column=1)
btn_close_peren = tk.Button(window, text=')', command=lambda: add_to_calculation(")"), width=5, font =("Arial", 14))
btn_close_peren.grid(row=5, column=3)
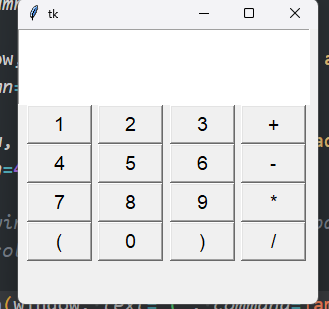
*I haven’t added the equals sign yet because it may need its own function.