Created the origin to follow the mouse by holding the middle mouse button.
editor.py
import pygame, sys
from settings import *
from pygame.math import Vector2 as vector
from pygame.mouse import get_pressed as mouse_buttons
from pygame.mouse import get_pos as mouse_pos
class Editor:
def __init__(self):
# main setup
self.display_surface = pygame.display.get_surface()
# navigation
self.origin = vector()
self.pan_active = False
def event_loop(self):
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
self.pan_input(event)
----------------------------------------- look between these
def pan_input(self, event):
'''checks if middle button is pressed'''
if event.type == pygame.MOUSEBUTTONDOWN and mouse_buttons()[1]:
self.pan_active = True
if not mouse_buttons()[1]:
self.pan_active = False
#panning update
if self.pan_active:
self.origin = mouse_pos()
------------------------------------- look between these
def run(self, dt):
self.display_surface.fill('white')
self.event_loop()
pygame.draw.circle(self.display_surface, 'blue', self.origin, 10)
The code between the dotted lines is what makes the origin move by holding down the mouse button.
Result:
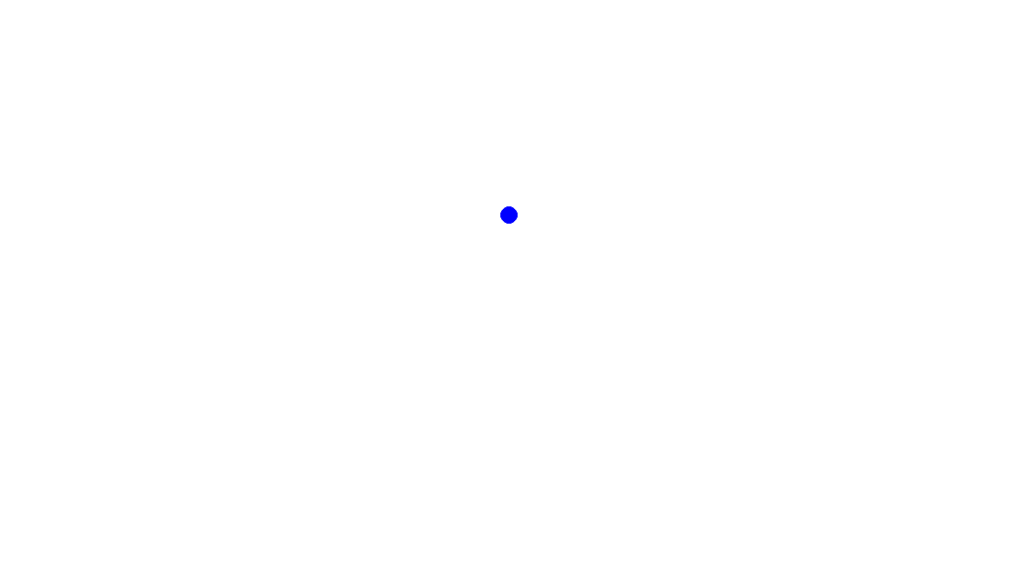
Origin in the middle.
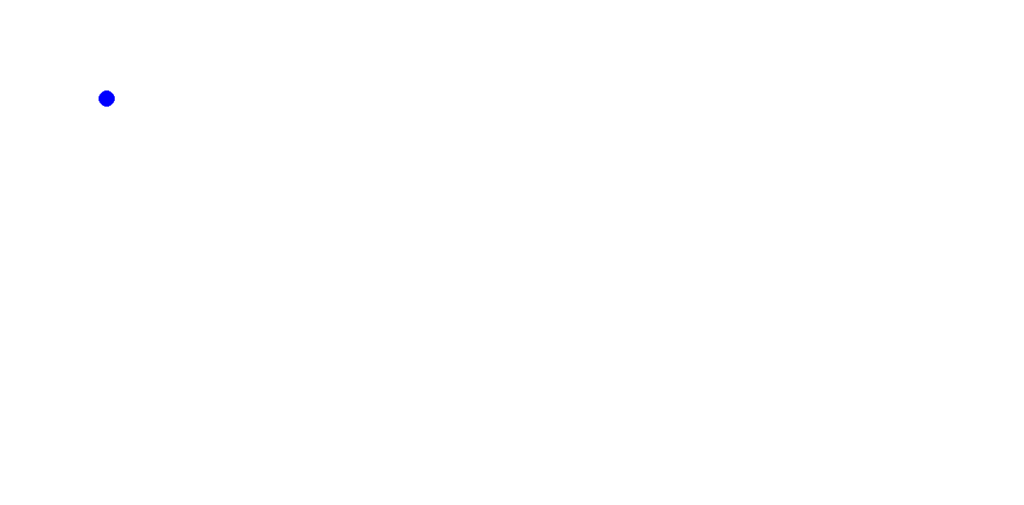
Origin on the left.
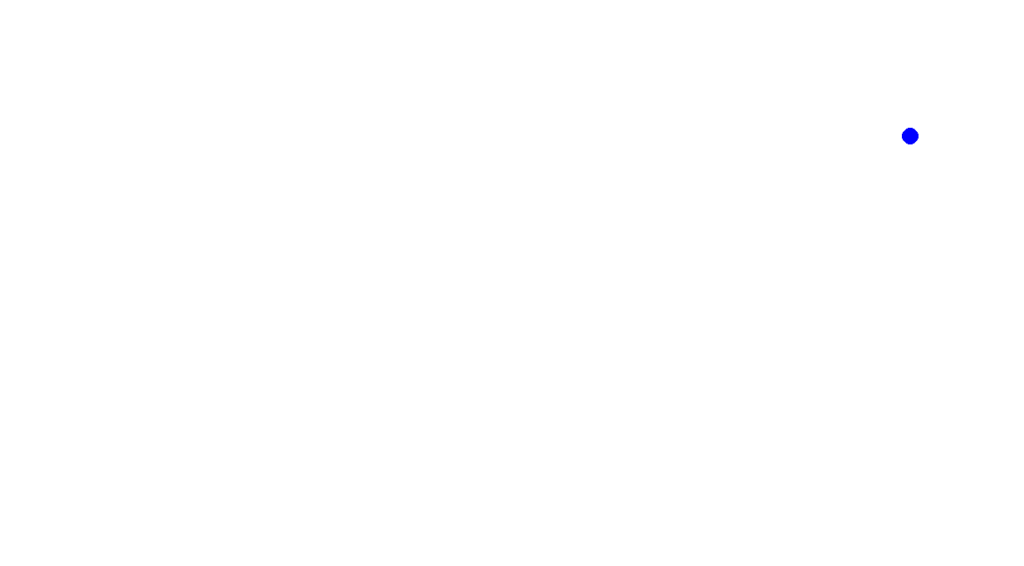
And the origin on the right.
There is a slight problem with this. The origin follows where the mouse’s position is. For example. If the mouse is at the bottom right-hand corner of the screen and the origin is on the top left-hand corner of the screen and the user presses the middle mouse button, the origin will go from its original position to the mouse’s position.
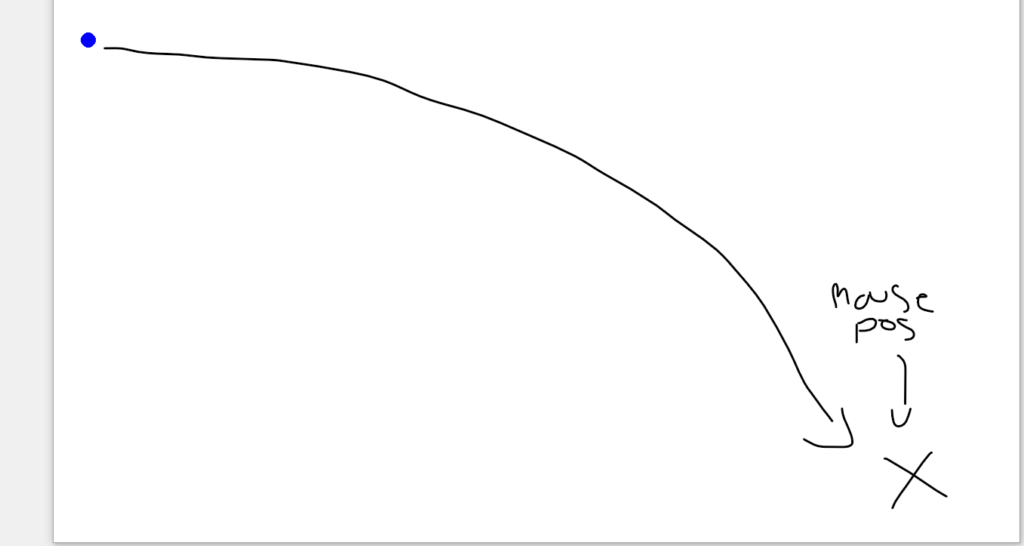
If we keep this, it will get pretty confusing later when we progress making the game, especially having different tiles and objects all around the screen.
The BETTER way to move the origin.
editor.py
def pan_input(self, event):
'''checks if middle button is pressed'''
if event.type == pygame.MOUSEBUTTONDOWN and mouse_buttons()[1]:
self.pan_active = True
self.pan_offset = vector(mouse_pos()) - self.origin
if not mouse_buttons()[1]:
self.pan_active = False
#panning update
if self.pan_active:
self.origin = vector(mouse_pos()) - self.pan_offset
The bolded lines of code helps with making it better to move the origin.
What you get from the first bolded line of code is the distance between the origin and the mouse position.
To use it you subtract the mouse_pos() with the pan_offset to get the distance IF the pan_active is true.
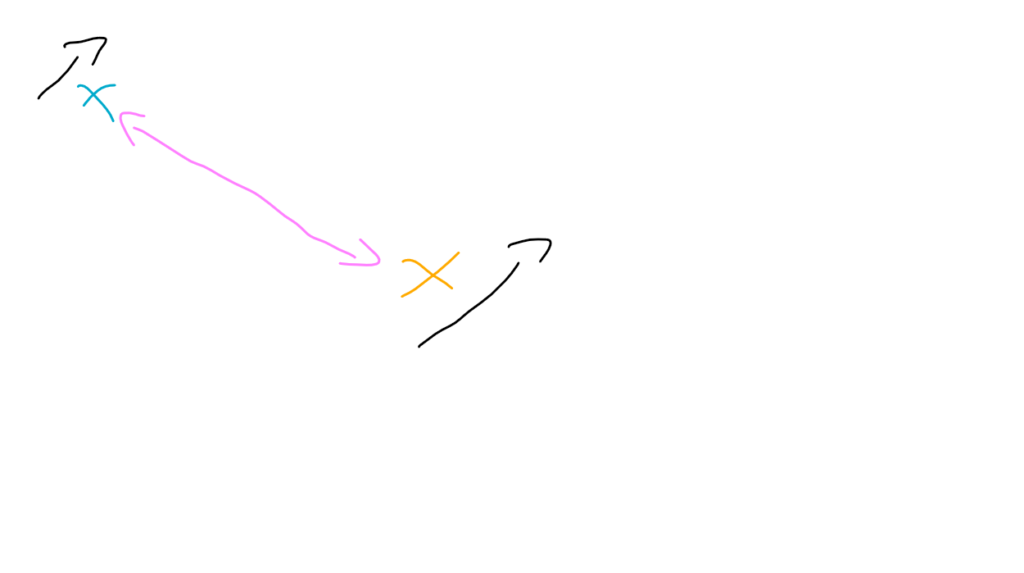
The teal x is the origin. The yellow one is the mouse. vector(mouse_pos()) – self.origin calculates the distance (in pink) between the mouse_pos() and the origin.
self.origin = vector(mouse_pos()) – self.pan_offset creates an offset of the pan when holding down the middle mouse button. This will move the origin point WITHOUT moving the origin point with the mouse (aka. clicking on it).
Result:
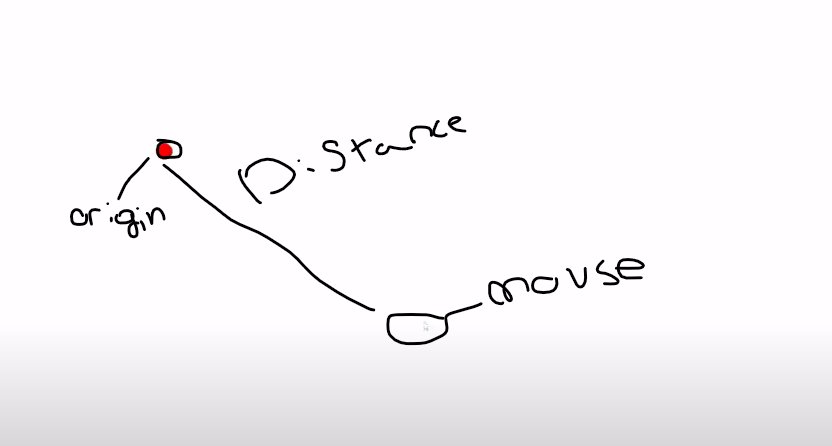
When moving the mouse, the origin will move with it, but not follow its exact position.
Ignore my chicken scratch. It’s hard writing with a right-handed mouse when you’re left-handed :/