Made the scroll wheel move the origin up, down, left and right
editor.py
if event.type == pygame.MOUSEWHEEL:
if pygame.key.get_pressed()[pygame.K_LCTRL]:
self.origin.y -= event.y * 50
else:
self.origin.x -= event.y * 50
Allows the origin to move or right WITHOUT holding down left CTRL and up or down when holding down left CTRL.
Without holding down left CTRL:
Holding down left CTRL:
That’s really it for the pan input. Nothing extremely complex. Later on we’ll have to add more stuff, but for now this is good.
Drawing the tile lines to find what location we are in the grid
Making the tile lines should be infinite and relative to the origin point.
All of the lines should start at the position 0 (start of the window) and end at the height (the bottom) of the window. The x position for the top and bottom of the line should be the same.
Very rough sketch of what I’m trying to explain:
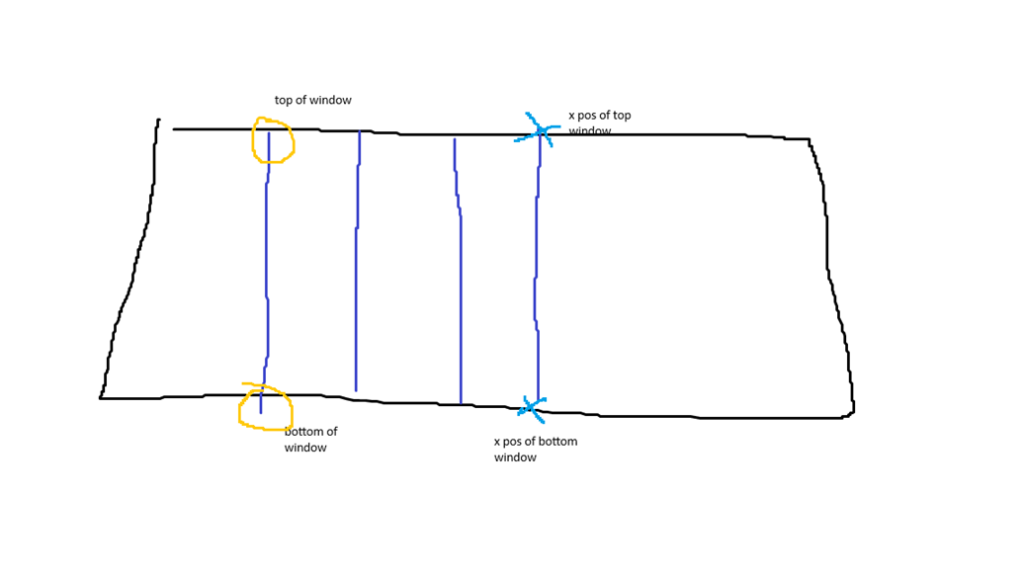
We would find this out by this code:
editor.py
for col in range(cols):
x = self.origin.x + col * TILE_SIZE
pygame.draw.line(self.display_surface, LINE_COLOR, (x,0), (x,WINDOW_HEIGHT))
Let’s say that the position of the origin point is (0,0). We would be getting that from self.origin.x. x = 0. We then add that to the column (which is 0) and then multiply by the TILE_SIZE (which will always be 64). The answer to that equation will be 0, which will be the line at the left side of the window.
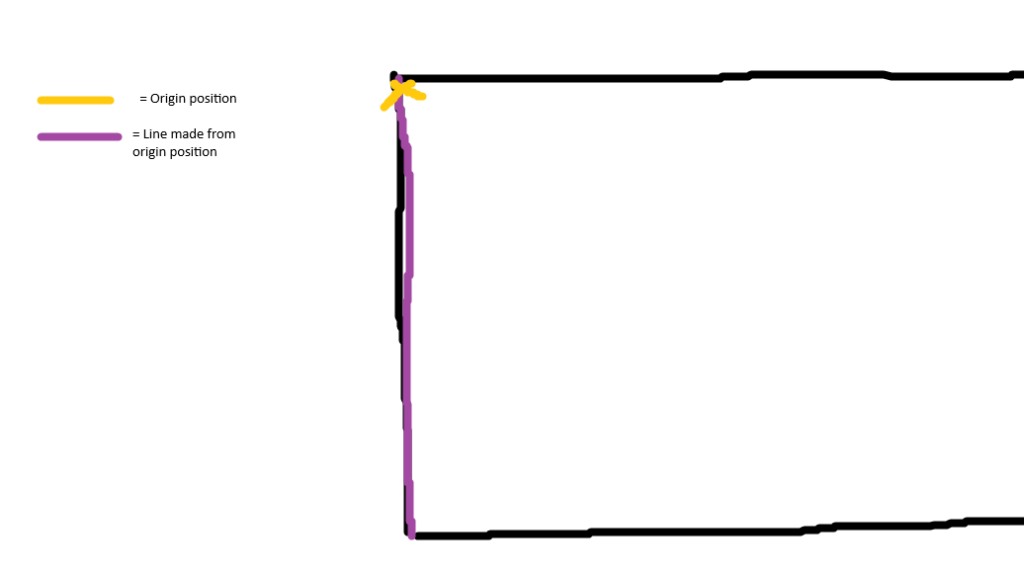
if col is 1 and we multiply that by 64, we’ll get 64, which will move from the first point to the point to the right and so on until we reach the end of the columns.
If we run it, this is our result:
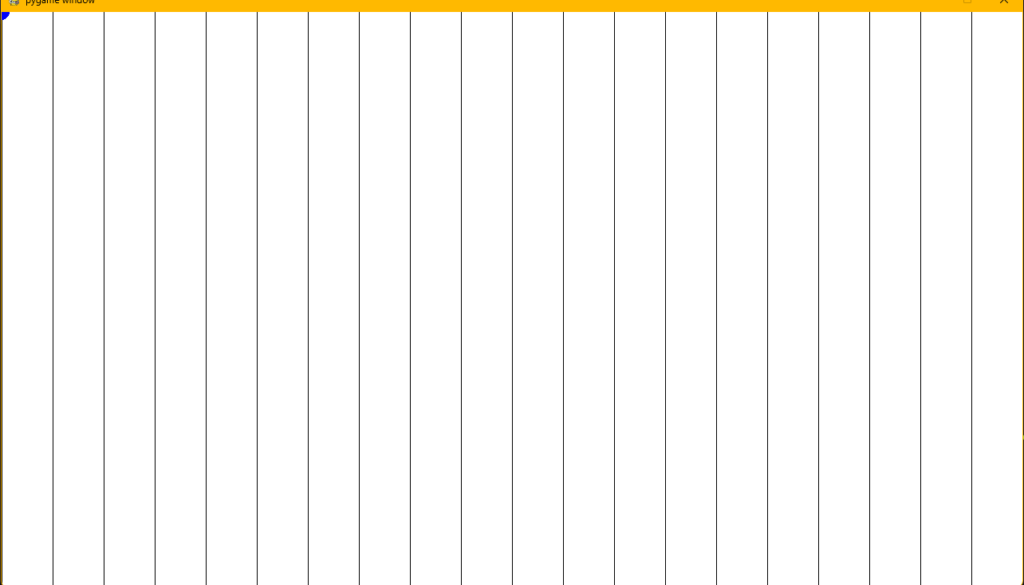
When you move the origin point the lines will move with it.
There’s a problem though. We run out of points when we move and there’s just a giant empty white space. The same thing happens when you move the origin point to the left.
What we have to do is make sure that the lines are always on the display and that we’re never running out of lines.
We will have to create columns between the origin’s position and the next column so that we can move the origin wherever we want (and so they don’t go out the window).
To do this, we have to divide origin_offset with the TILE_SIZE. Let’s say it is 100. We divide that by the TILE_SIZE (64) and then multiply that number by the TILE_SIZE, which would be 1, which means we’re in the 1st column. Same thing for y.
editor.py
origin_offset = vector(
offset_vector.x - int(self.origin.x / TILE_SIZE)* TILE_SIZE,
y = self.origin.y - int(self.origin.y / TILE_SIZE)* TILE_SIZE)
Result:
That’s all I did. Keep in mind that this tutorial is over 10 hours long…