So my main.py file for the racing game just pooped out for no reason and I can’t open it back up without getting an error. The only thing I am able to open is utils.py and the images. That’s it.

So, I copied the code from github and started off from where I think I left off.
Added the images for the game
main.py
GRASS = scale_image(pygame.image.load("imgs/grass.jpg"), 2.5)
TRACK = scale_image(pygame.image.load("imgs/track.png"), 0.9)
TRACK_BORDER = scale_image(pygame.image.load("imgs/track-border.png"), 0.9)
RED_CAR = scale_image(pygame.image.load("imgs/red-car.png"), 0.55)
GREY_CAR = scale_image(pygame.image.load("imgs/grey-car.png"), 0.55)
WIDTH, HEIGHT = TRACK.get_width(), TRACK.get_height()
WIN = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Racing Game!")
made a class called AbstractCar to initialize the velocity, angle and acceleration. PlayerCar inherits from this class.
main.py
class AbstractCar:
def __init__(self, max_vel, rotation_vel):
self.img = self.IMG
self.max_vel = max_vel
self.vel = 0
self.rotation_vel = rotation_vel
self.angle = 0
self.x, self.y = self.START_POS
self.acceleration = 0.1
def rotate(self, left=False, right=False):
if left:
self.angle += self.rotation_vel
elif right:
self.angle -= self.rotation_vel
def draw(self, win):
blit_rotate_center(win, self.img, (self.x, self.y), self.angle)
def move_forward(self):
self.vel = min(self.vel + self.acceleration, self.max_vel)
self.move()
def move(self):
radians = math.radians(self.angle)
vertical = math.cos(radians) * self.vel
horizontal = math.sin(radians) * self.vel
self.y -= vertical
self.x -= horizontal
def reduce_speed(self):
self.vel = max(self.vel - self.acceleration / 2, 0)
self.move()
PlayerCar is MUCH shorter and only has the image of the car that we need and the start position of the car.
class PlayerCar(AbstractCar):
IMG = GREY_CAR
START_POS = (180, 200)
and a draw function that draws the images, and the player car on the screen.
def draw(win, images, player_car):
for img, pos in images:
win.blit(img, pos)
player_car.draw(win)
pygame.display.update()
made while loop function that starts and quits the game (when the user clicks the x) and also has the keys to control the player car.
while run:
clock.tick(FPS)
draw(WIN, images, player_car)
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
break
keys = pygame.key.get_pressed()
moved = False
if keys[pygame.K_a]:
player_car.rotate(left=True)
if keys[pygame.K_d]:
player_car.rotate(right=True)
if keys[pygame.K_w]:
moved = True
player_car.move_forward()
if not moved:
player_car.reduce_speed()
pygame.quit()
result:
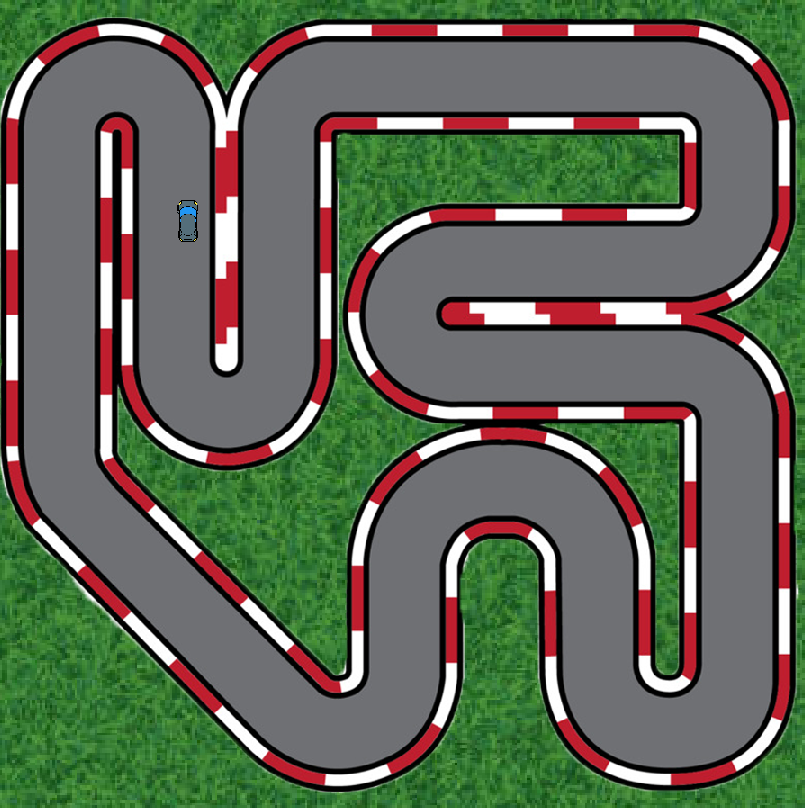
You can move it but these dang computers don’t have screen recording (curse you IT!!!!)