HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="calculator">
<input id="display" readonly>
<div id="keys">
<button onclick="appendToDisplay('+')" class="operator-btn">+</button>
<button onclick="appendToDisplay('7')">7</button>
<button onclick="appendToDisplay('8')">8</button>
<button onclick="appendToDisplay('9')">9</button>
<button onclick="appendToDisplay('-')" class="operator-btn">-</button>
<button onclick="appendToDisplay('4')">4</button>
<button onclick="appendToDisplay('5')">5</button>
<button onclick="appendToDisplay('6')">6</button>
<button onclick="appendToDisplay('*')" class="operator-btn">*</button>
<button onclick="appendToDisplay('1')">1</button>
<button onclick="appendToDisplay('2')">2</button>
<button onclick="appendToDisplay('3')">3</button>
<button onclick="appendToDisplay('/')" class="operator-btn">/</button>
<button onclick="appendToDisplay('0')">0</button>
<button onclick="appendToDisplay('.')">.</button>
<button onclick="calculate()">=</button>
<button onclick="clearDisplay()" class="operator-btn">C</button>
</div>
</div>
<script src="index.js"></script>
</body>
</html>
CSS:
body{
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: hsl(0, 0%, 90%);
}
#calculator{
font-family: Arial, sans-serif;
background-color: hsl(0, 0%, 15%);
border-radius: 15px;
max-width: 500px;
overflow: hidden;
}
#display{
width: 100%;
padding: 20px;
font-size: 5rem;
text-align: left;
border: none;
background-color: hsl(0, 0%, 20%);
}
#keys{
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
padding: 25px;
}
button{
width: 100px;
height: 100px;
border-radius: 50px;
border: none;
background-color: hsl(0, 0%, 30%);
color: white;
font-size: 3rem;
font-weight: bold;
cursor: pointer;
}
button:hover{
background-color: hsl(0, 0%, 40%);
}
button:active{
background-color: hsl(0, 0%, 60%);
}
.operator-btn{
background-color: hsl(198, 83%, 47%);
}
.operator-btn:hover{
background-color: hsl(198, 83%, 67%);
}
.operator-btn:active{
background-color: hsl(198, 83%, 87%);
}
I don’t have JS yet because I’ve not finished it :/
Created the display screen and buttons
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="calculator">
<input id="display" readonly>
<div id="keys">
<button onclick="appendToDisplay('+')" class="operator-btn">+</button>
<button onclick="appendToDisplay('7')">7</button>
<button onclick="appendToDisplay('8')">8</button>
<button onclick="appendToDisplay('9')">9</button>
<button onclick="appendToDisplay('-')" class="operator-btn">-</button>
<button onclick="appendToDisplay('4')">4</button>
<button onclick="appendToDisplay('5')">5</button>
<button onclick="appendToDisplay('6')">6</button>
<button onclick="appendToDisplay('*')" class="operator-btn">*</button>
<button onclick="appendToDisplay('1')">1</button>
<button onclick="appendToDisplay('2')">2</button>
<button onclick="appendToDisplay('3')">3</button>
<button onclick="appendToDisplay('/')" class="operator-btn">/</button>
<button onclick="appendToDisplay('0')">0</button>
<button onclick="appendToDisplay('.')">.</button>
<button onclick="calculate()">=</button>
<button onclick="clearDisplay()" class="operator-btn">C</button>
</div>
</div>
<script src="index.js"></script>
</body>
</html>
result:
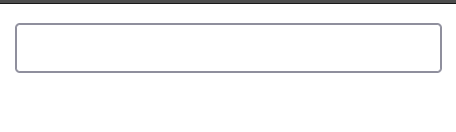
wow so cool!!!!!
and the buttons

okay not so cool…
Made the buttons less gross using Styles.css
styles.css
#keys{
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
padding: 25px;
}
button{
width: 100px;
height: 100px;
border-radius: 50px;
border: none;
background-color: hsl(0, 0%, 30%);
color: white;
font-size: 3rem;
font-weight: bold;
cursor: pointer;
}
#keys is the id of the buttons. it changes the size and spaces the buttons have. button customizes the buttons to make them look better.
result:
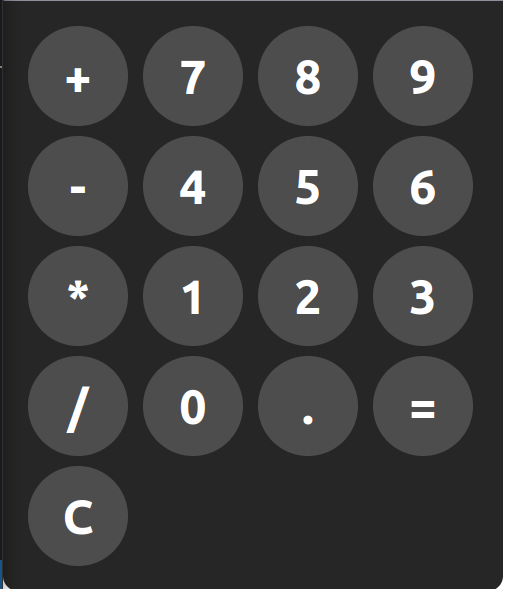
The buttons
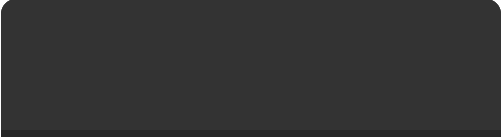
Display screen (I just changed the color and made it larger)
Code of the display screen:
#display{
width: 100%;
padding: 20px;
font-size: 5rem;
text-align: left;
border: none;
background-color: hsl(0, 0%, 20%);
}
Made the calculator center in the document.
styles.css
body{
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: hsl(0, 0%, 90%);
}
before:
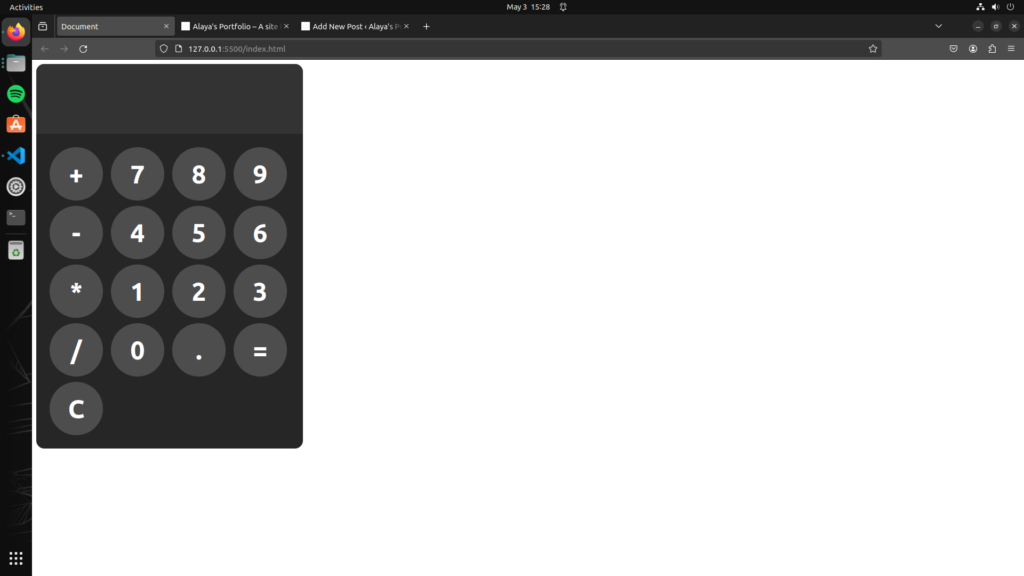
after:
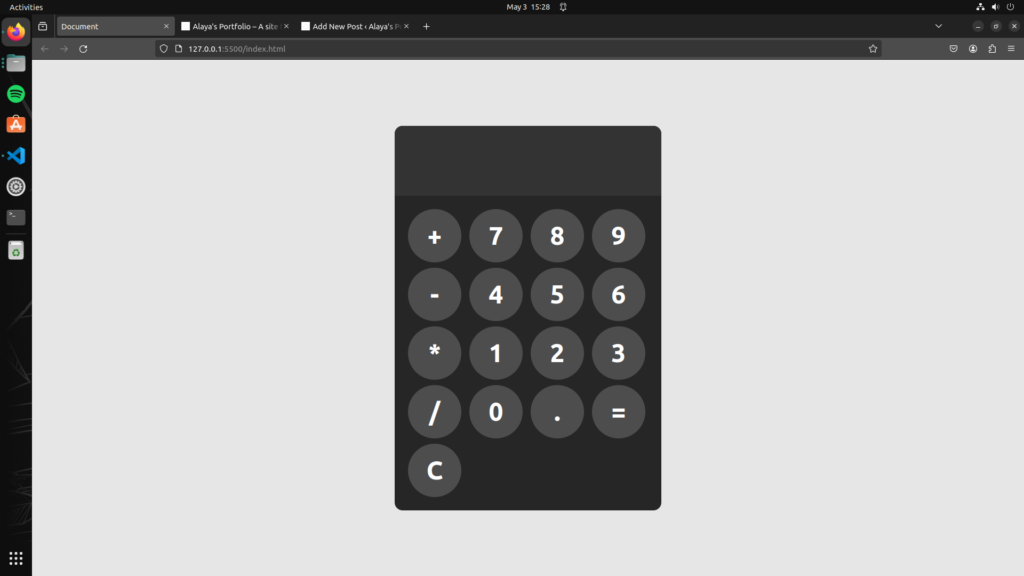
the screen is darker b/c i changed the background color.
Added button hovering and clicking indications(?)(and color)
styles.css
body{
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: hsl(0, 0%, 90%);
}
#calculator{
font-family: Arial, sans-serif;
background-color: hsl(0, 0%, 15%);
border-radius: 15px;
max-width: 500px;
overflow: hidden;
}
#display{
width: 100%;
padding: 20px;
font-size: 5rem;
text-align: left;
border: none;
background-color: hsl(0, 0%, 20%);
}
#keys{
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
padding: 25px;
}
button{
width: 100px;
height: 100px;
border-radius: 50px;
border: none;
background-color: hsl(0, 0%, 30%);
color: white;
font-size: 3rem;
font-weight: bold;
cursor: pointer;
}
button:hover{
background-color: hsl(0, 0%, 40%);
}
button:active{
background-color: hsl(0, 0%, 60%);
}
.operator-btn{
background-color: hsl(198, 83%, 47%);
}
.operator-btn:hover{
background-color: hsl(198, 83%, 67%);
}
.operator-btn:active{
background-color: hsl(198, 83%, 87%);
}
button hovering
button clicking
COLORED OPERATOR BUTTONS AYAYAYAYA
I did a miniscule bit of JS, but it’s not an ABSURD amount.