Added the Y tile lines for the game
editor.py
for row in range(rows + 1):
y = origin_offset.y + row * TILE_SIZE
pygame.draw.line(self.display_surface, LINE_COLOR, (0,y), (WINDOW_WIDTH,y))
Same as x, but it makes lines horizontal from the left to the right of the window
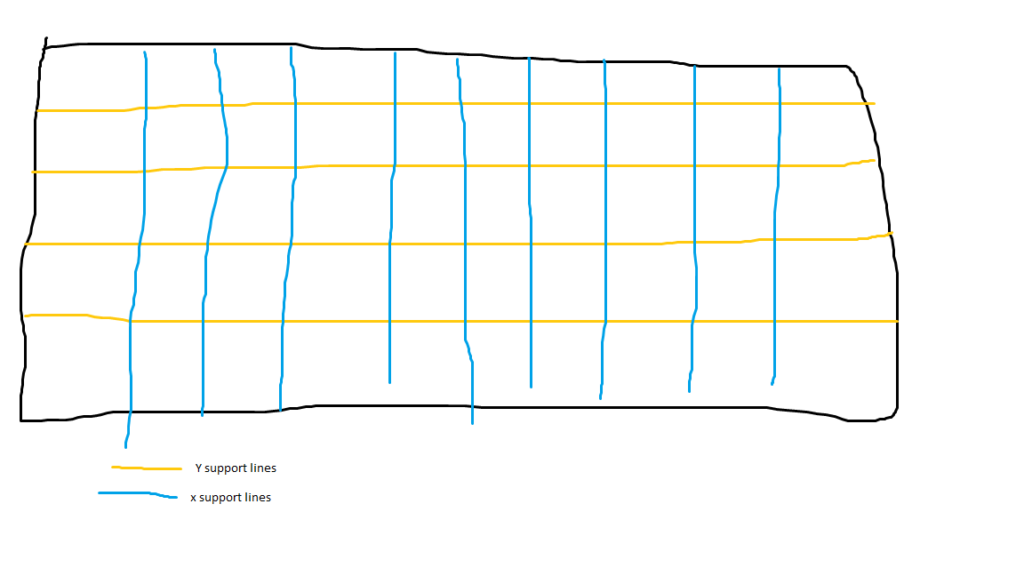
This is what the it should look like. The y tile lines will be horizontal, and the x tile lines will be vertical.
Result:
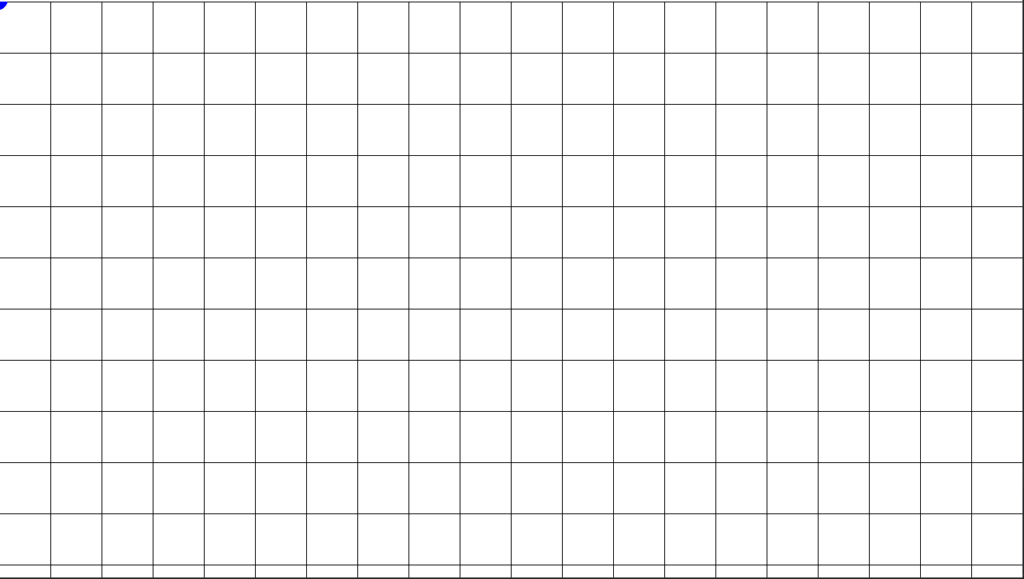
Tried to make the lines green so it will look better and…
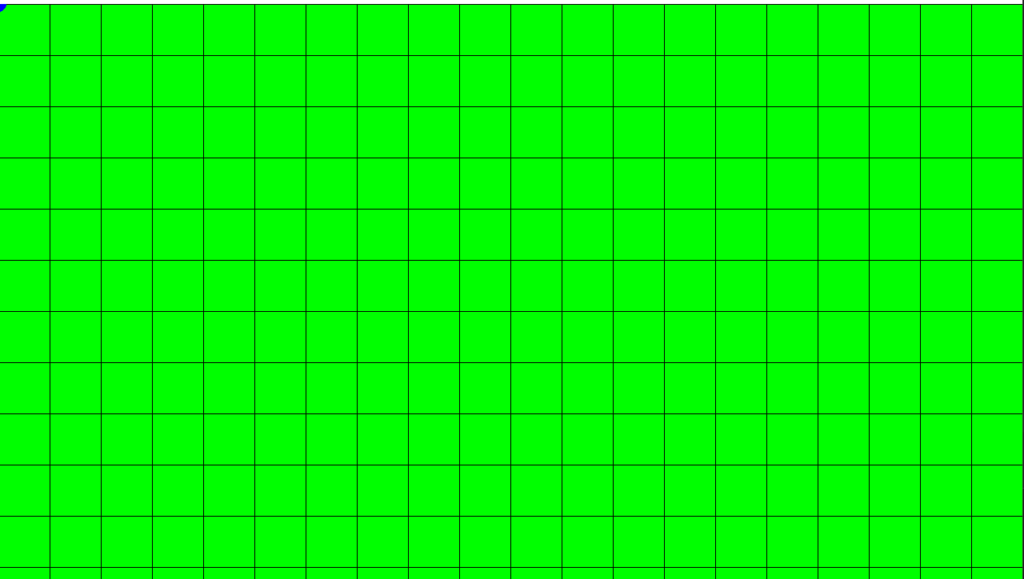
oh boy…
Okay I took out the green and made the lines a little transparent.

Changing the mouse cursor
Changed the mouse cursor to this:

What we need in order to change the mouse is to find the clickable area, which would be somewhere around the tip of the mouse, the rest is the attached stuff to it.
main.py
surf = load('.//graphics//cursor//mouse.png').convert_alpha()
cursor = pygame.cursors.Cursor((0,0), surf)
pygame.mouse.set_cursor(cursor)
We load the image in, then we set where it should be clickable. In this case, it’s (o,0). After that, we replace the mouse with the cursor that we have.
result:
Creating the menu
To make the menu work, in editor.py we’ll have a variable called selection_index that will have value between 2 and 18. Each number represents a certain kind of tile in the editor.
Ex. 2: water, 3; terrain, 4: gold coin and so on.
The selection_index can be changed by clicking on the menu or via hotkeys (this is where that colossal file settings.py comes in).
settings.py
# general setup
TILE_SIZE = 64
WINDOW_WIDTH = 1280
WINDOW_HEIGHT = 720
ANIMATION_SPEED = 8
# editor graphics
EDITOR_DATA = {
0: {'style': 'player', 'type': 'object', 'menu': None, 'menu_surf': None, 'preview': None, 'graphics': '../graphics/player/idle_right'},
1: {'style': 'sky', 'type': 'object', 'menu': None, 'menu_surf': None, 'preview': None, 'graphics': None},
2: {'style': 'terrain', 'type': 'tile', 'menu': 'terrain', 'menu_surf': '../graphics/menu/land.png', 'preview': '../graphics/preview/land.png', 'graphics': None},
3: {'style': 'water', 'type': 'tile', 'menu': 'terrain', 'menu_surf': '../graphics/menu/water.png', 'preview': '../graphics/preview/water.png', 'graphics': '../graphics/terrain/water/animation'},
4: {'style': 'coin', 'type': 'tile', 'menu': 'coin', 'menu_surf': '../graphics/menu/gold.png', 'preview': '../graphics/preview/gold.png', 'graphics': '../graphics/items/gold'},
5: {'style': 'coin', 'type': 'tile', 'menu': 'coin', 'menu_surf': '../graphics/menu/silver.png', 'preview': '../graphics/preview/silver.png', 'graphics': '../graphics/items/silver'},
6: {'style': 'coin', 'type': 'tile', 'menu': 'coin', 'menu_surf': '../graphics/menu/diamond.png', 'preview': '../graphics/preview/diamond.png', 'graphics': '../graphics/items/diamond'},
7: {'style': 'enemy', 'type': 'tile', 'menu': 'enemy', 'menu_surf': '../graphics/menu/spikes.png', 'preview': '../graphics/preview/spikes.png', 'graphics': '../graphics/enemies/spikes'},
8: {'style': 'enemy', 'type': 'tile', 'menu': 'enemy', 'menu_surf': '../graphics/menu/tooth.png', 'preview': '../graphics/preview/tooth.png', 'graphics': '../graphics/enemies/tooth/idle'},
9: {'style': 'enemy', 'type': 'tile', 'menu': 'enemy', 'menu_surf': '../graphics/menu/shell_left.png', 'preview': '../graphics/preview/shell_left.png', 'graphics': '../graphics/enemies/shell_left/idle'},
10: {'style': 'enemy', 'type': 'tile', 'menu': 'enemy', 'menu_surf': '../graphics/menu/shell_right.png', 'preview': '../graphics/preview/shell_right.png', 'graphics': '../graphics/enemies/shell_right/idle'},
11: {'style': 'palm_fg', 'type': 'object', 'menu': 'palm fg', 'menu_surf': '../graphics/menu/small_fg.png', 'preview': '../graphics/preview/small_fg.png', 'graphics': '../graphics/terrain/palm/small_fg'},
12: {'style': 'palm_fg', 'type': 'object', 'menu': 'palm fg', 'menu_surf': '../graphics/menu/large_fg.png', 'preview': '../graphics/preview/large_fg.png', 'graphics': '../graphics/terrain/palm/large_fg'},
13: {'style': 'palm_fg', 'type': 'object', 'menu': 'palm fg', 'menu_surf': '../graphics/menu/left_fg.png', 'preview': '../graphics/preview/left_fg.png', 'graphics': '../graphics/terrain/palm/left_fg'},
14: {'style': 'palm_fg', 'type': 'object', 'menu': 'palm fg', 'menu_surf': '../graphics/menu/right_fg.png', 'preview': '../graphics/preview/right_fg.png', 'graphics': '../graphics/terrain/palm/right_fg'},
15: {'style': 'palm_bg', 'type': 'object', 'menu': 'palm bg', 'menu_surf': '../graphics/menu/small_bg.png', 'preview': '../graphics/preview/small_bg.png', 'graphics': '../graphics/terrain/palm/small_bg'},
16: {'style': 'palm_bg', 'type': 'object', 'menu': 'palm bg', 'menu_surf': '../graphics/menu/large_bg.png', 'preview': '../graphics/preview/large_bg.png', 'graphics': '../graphics/terrain/palm/large_bg'},
17: {'style': 'palm_bg', 'type': 'object', 'menu': 'palm bg', 'menu_surf': '../graphics/menu/left_bg.png', 'preview': '../graphics/preview/left_bg.png', 'graphics': '../graphics/terrain/palm/left_bg'},
18: {'style': 'palm_bg', 'type': 'object', 'menu': 'palm bg', 'menu_surf': '../graphics/menu/right_bg.png', 'preview': '../graphics/preview/right_bg.png', 'graphics': '../graphics/terrain/palm/right_bg'},
}
NEIGHBOR_DIRECTIONS = {
'A': (0,-1),
'B': (1,-1),
'C': (1,0),
'D': (1,1),
'E': (0,1),
'F': (-1,1),
'G': (-1,0),
'H': (-1,-1)
}
LEVEL_LAYERS = {
'clouds': 1,
'ocean': 2,
'bg': 3,
'water': 4,
'main': 5
}
# colors
SKY_COLOR = '#ddc6a1'
SEA_COLOR = '#92a9ce'
HORIZON_COLOR = '#f5f1de'
HORIZON_TOP_COLOR = '#d1aa9d'
LINE_COLOR = 'black'
what we will need are the indexes 2-18. 0 and 1 are ignored b/c 0 is the player and 1 is the sky. They will also be in the editor so they don’t need to be created.
2-18 the player can create like the terrain, coin, etc…
Making the hotkeys for the menu
editor.py
def selection_hotkeys(self, event):
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_RIGHT:
self.selection_index += 1
if event.key == pygame.K_LEFT:
self.selection_index -= 1
Detects if the user is pressing a button. It’s not checking if we’re holding down a button so there’s no need for a timer.
The problem is that when you repeatedly press the button the number can go below 0 and above 18.
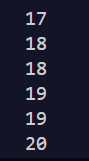
Above 18 when pressing right arrow.
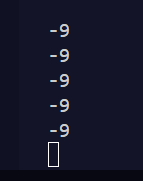
Below 0 when pressing left arrow.
To fix it we just needed one line of code.
editor.py
self.selection_index = max(2,min(self.selection_index,18))
This will cap the max at 18 and the min at 2.
Building the menu
First, we’ll create the general area of the menu.
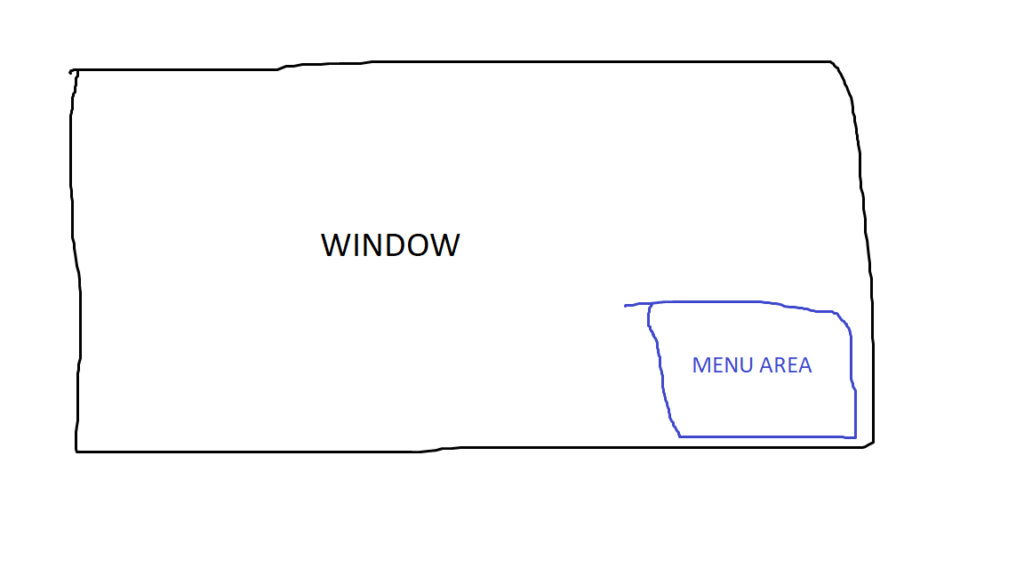
In the menu area we’ll have smaller squares the user can click on.
menu.py
size = 180
margin = 6
The width and height of the menu will be size. The left and top of the menu will be the WINDOW_WIDTH – size – margin.
Then we draw it on the window.
menu.py
pygame.draw.rect(self.display_surface, 'blue', self.rect)
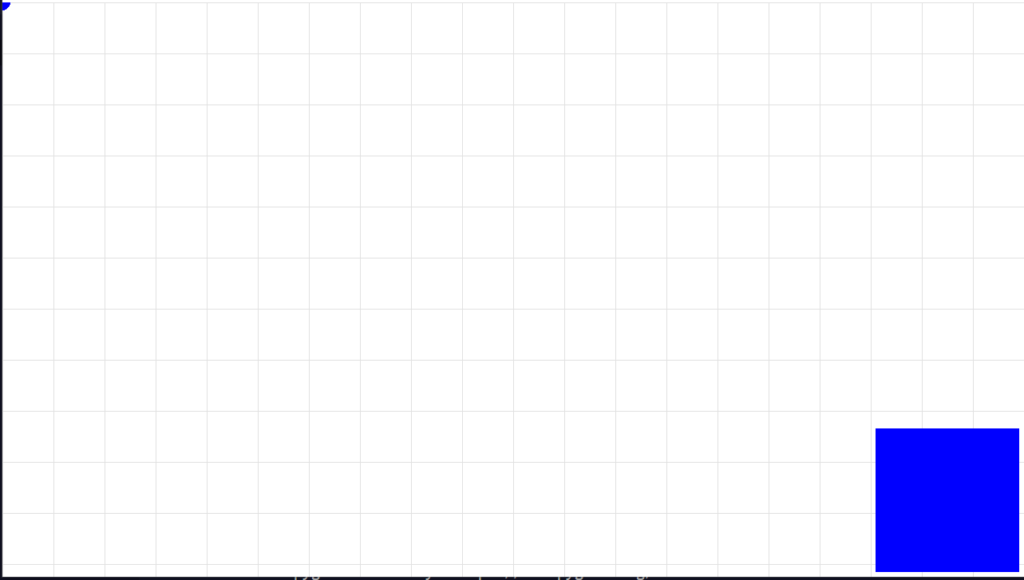
The menu took a little bit for me b/c there was something wrong with the place of the rectangle. It kept going offscreen (I put a random number for one of the window dimensions instead of its variable).