This year went by FAST. Quicker than sophomore year and MUCH quicker than freshman year. I honestly don’t know what things we did this year that I liked the most. I did like learning about JavaScript and using it to make websites in the backend, even though it was pretty confusing. I do like messing around in the frontend more than the backend because it gives me more freedom, but the backend makes your website POP in a way that the frontend doesn’t. IDK I know there’s tons of other stuff we’ve done this year but my mind has forced me to not remember maybe because of how stressful and frustrating it was, but that’s how it is with coding. I kinda enjoyed this year. I just hope senior year is just as fun ;-;.
Tag: #javascript
Calculator in JS
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="calculator">
<input id="display" readonly>
<div id="keys">
<button onclick="appendToDisplay('+')" class="operator-btn">+</button>
<button onclick="appendToDisplay('7')">7</button>
<button onclick="appendToDisplay('8')">8</button>
<button onclick="appendToDisplay('9')">9</button>
<button onclick="appendToDisplay('-')" class="operator-btn">-</button>
<button onclick="appendToDisplay('4')">4</button>
<button onclick="appendToDisplay('5')">5</button>
<button onclick="appendToDisplay('6')">6</button>
<button onclick="appendToDisplay('*')" class="operator-btn">*</button>
<button onclick="appendToDisplay('1')">1</button>
<button onclick="appendToDisplay('2')">2</button>
<button onclick="appendToDisplay('3')">3</button>
<button onclick="appendToDisplay('/')" class="operator-btn">/</button>
<button onclick="appendToDisplay('0')">0</button>
<button onclick="appendToDisplay('.')">.</button>
<button onclick="calculate()">=</button>
<button onclick="clearDisplay()" class="operator-btn">C</button>
</div>
</div>
<script src="index.js"></script>
</body>
</html>
CSS:
body{
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: hsl(0, 0%, 90%);
}
#calculator{
font-family: Arial, sans-serif;
background-color: hsl(0, 0%, 15%);
border-radius: 15px;
max-width: 500px;
overflow: hidden;
}
#display{
width: 100%;
padding: 20px;
font-size: 5rem;
text-align: left;
border: none;
background-color: hsl(0, 0%, 20%);
}
#keys{
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
padding: 25px;
}
button{
width: 100px;
height: 100px;
border-radius: 50px;
border: none;
background-color: hsl(0, 0%, 30%);
color: white;
font-size: 3rem;
font-weight: bold;
cursor: pointer;
}
button:hover{
background-color: hsl(0, 0%, 40%);
}
button:active{
background-color: hsl(0, 0%, 60%);
}
.operator-btn{
background-color: hsl(198, 83%, 47%);
}
.operator-btn:hover{
background-color: hsl(198, 83%, 67%);
}
.operator-btn:active{
background-color: hsl(198, 83%, 87%);
}
I don’t have JS yet because I’ve not finished it :/
Created the display screen and buttons
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div id="calculator">
<input id="display" readonly>
<div id="keys">
<button onclick="appendToDisplay('+')" class="operator-btn">+</button>
<button onclick="appendToDisplay('7')">7</button>
<button onclick="appendToDisplay('8')">8</button>
<button onclick="appendToDisplay('9')">9</button>
<button onclick="appendToDisplay('-')" class="operator-btn">-</button>
<button onclick="appendToDisplay('4')">4</button>
<button onclick="appendToDisplay('5')">5</button>
<button onclick="appendToDisplay('6')">6</button>
<button onclick="appendToDisplay('*')" class="operator-btn">*</button>
<button onclick="appendToDisplay('1')">1</button>
<button onclick="appendToDisplay('2')">2</button>
<button onclick="appendToDisplay('3')">3</button>
<button onclick="appendToDisplay('/')" class="operator-btn">/</button>
<button onclick="appendToDisplay('0')">0</button>
<button onclick="appendToDisplay('.')">.</button>
<button onclick="calculate()">=</button>
<button onclick="clearDisplay()" class="operator-btn">C</button>
</div>
</div>
<script src="index.js"></script>
</body>
</html>
result:
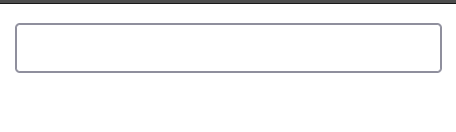
wow so cool!!!!!
and the buttons

okay not so cool…
Made the buttons less gross using Styles.css
styles.css
#keys{
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
padding: 25px;
}
button{
width: 100px;
height: 100px;
border-radius: 50px;
border: none;
background-color: hsl(0, 0%, 30%);
color: white;
font-size: 3rem;
font-weight: bold;
cursor: pointer;
}
#keys is the id of the buttons. it changes the size and spaces the buttons have. button customizes the buttons to make them look better.
result:
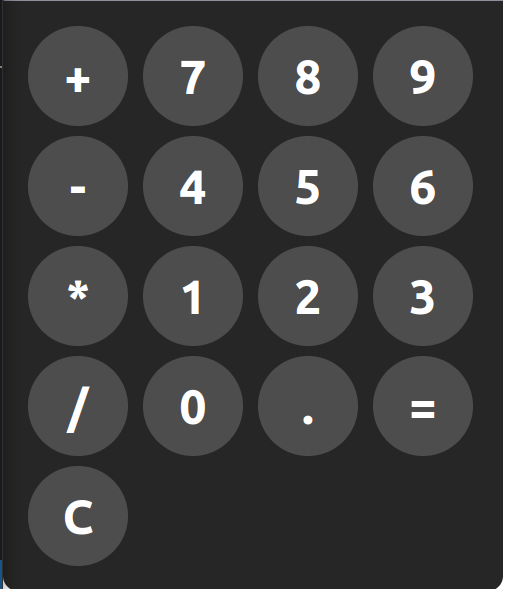
The buttons
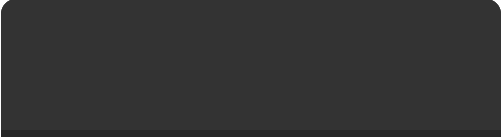
Display screen (I just changed the color and made it larger)
Code of the display screen:
#display{
width: 100%;
padding: 20px;
font-size: 5rem;
text-align: left;
border: none;
background-color: hsl(0, 0%, 20%);
}
Made the calculator center in the document.
styles.css
body{
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: hsl(0, 0%, 90%);
}
before:
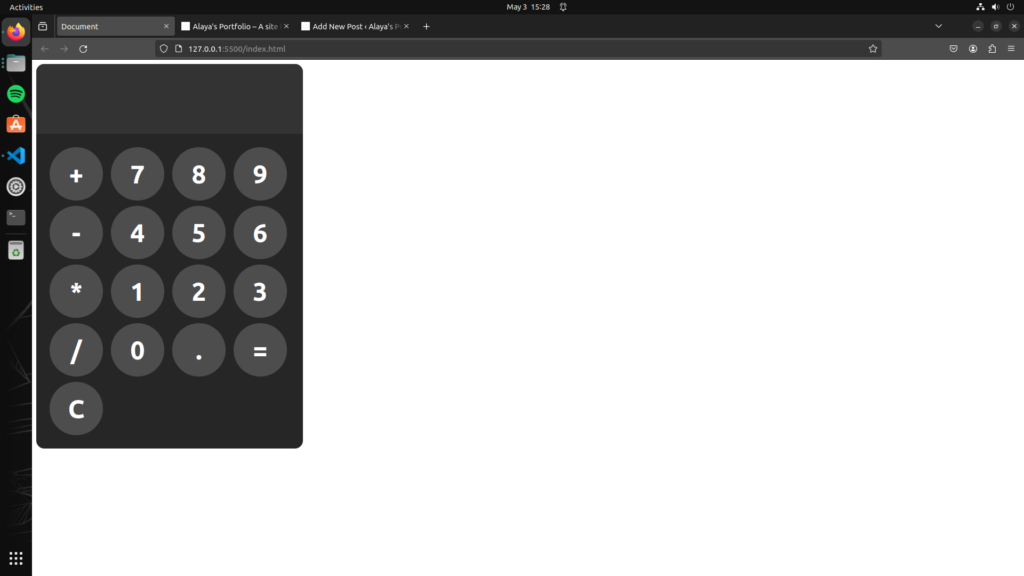
after:
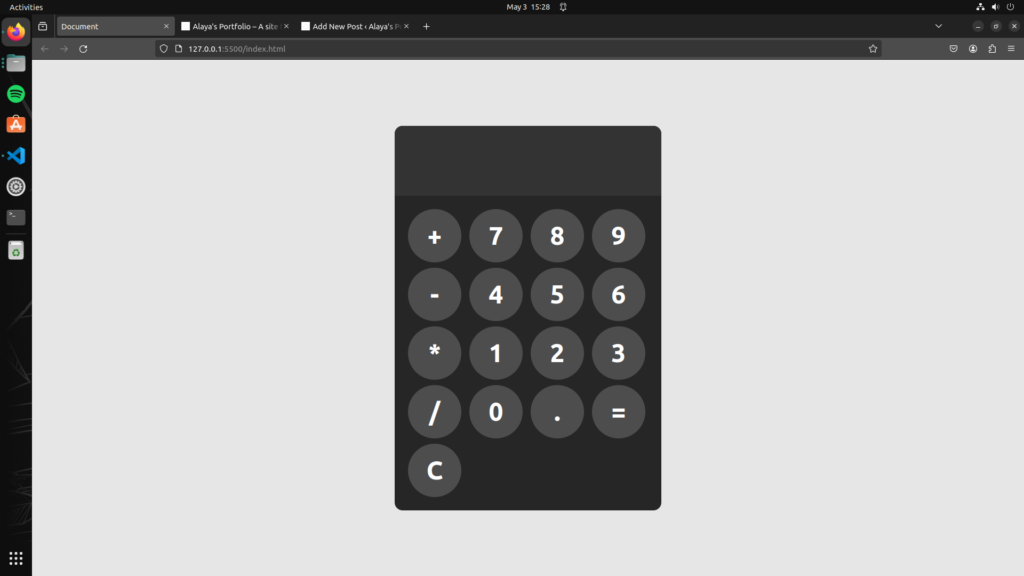
the screen is darker b/c i changed the background color.
Added button hovering and clicking indications(?)(and color)
styles.css
body{
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: hsl(0, 0%, 90%);
}
#calculator{
font-family: Arial, sans-serif;
background-color: hsl(0, 0%, 15%);
border-radius: 15px;
max-width: 500px;
overflow: hidden;
}
#display{
width: 100%;
padding: 20px;
font-size: 5rem;
text-align: left;
border: none;
background-color: hsl(0, 0%, 20%);
}
#keys{
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
padding: 25px;
}
button{
width: 100px;
height: 100px;
border-radius: 50px;
border: none;
background-color: hsl(0, 0%, 30%);
color: white;
font-size: 3rem;
font-weight: bold;
cursor: pointer;
}
button:hover{
background-color: hsl(0, 0%, 40%);
}
button:active{
background-color: hsl(0, 0%, 60%);
}
.operator-btn{
background-color: hsl(198, 83%, 47%);
}
.operator-btn:hover{
background-color: hsl(198, 83%, 67%);
}
.operator-btn:active{
background-color: hsl(198, 83%, 87%);
}
button hovering
button clicking
COLORED OPERATOR BUTTONS AYAYAYAYA
I did a miniscule bit of JS, but it’s not an ABSURD amount.
Data Types In JavaScript
There are six different data types in JavaScript: Strings, integers, floats (decimal numbers), Boolean, null (which is “None” in Python), undefined and Symbol.
Strings
Strings are words (or numbers) that are surrounded by quotation marks (“”).
Here’s an example of one in JavaScript:
const name = "Zende";
As you can see, the word Zende is the string because it has quotes around it.
Output:

*the variable const is used so that we can’t change the value of the variable. if we were to try to change the value of name, we would get an error message say we’re not allowed.

Numbers
Here’s an example of an integer:
const age = 16;
And example of a float:
const temp = 98.6;
BOOLEAN
Here’s an example of a boolean:
const hasKis = true;
This makes the variable true. if we were to type false, it will make it false.
Null
Null, which is the same as “None” in python, happens when a variable doesn’t exist yet.
Here’s an example:
const homeRoom = null;
Undefined
undefined happens when a variable exist, but isn’t defined in the program.
Here’s an example:
const kd = undefined;
Symbol
symbols variables that always have to be unique. They cannot be the same.
Here’s an example:
const id = Symbol('id');
const id2 = Symbol('id');
And those are all the data types.