Today, I worked on type conversion with Java Script. Here is some of the code used for type conversion.
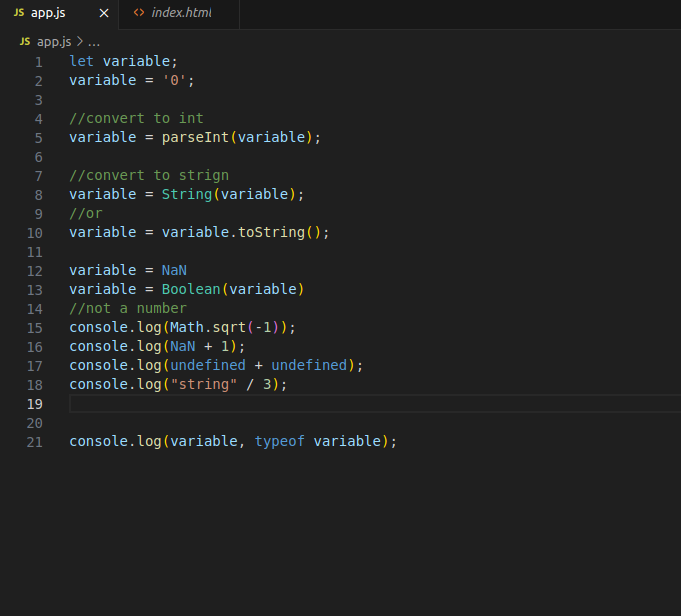
This code converts a data type from one to another. ‘0’ as a string turns into 0 as an int.
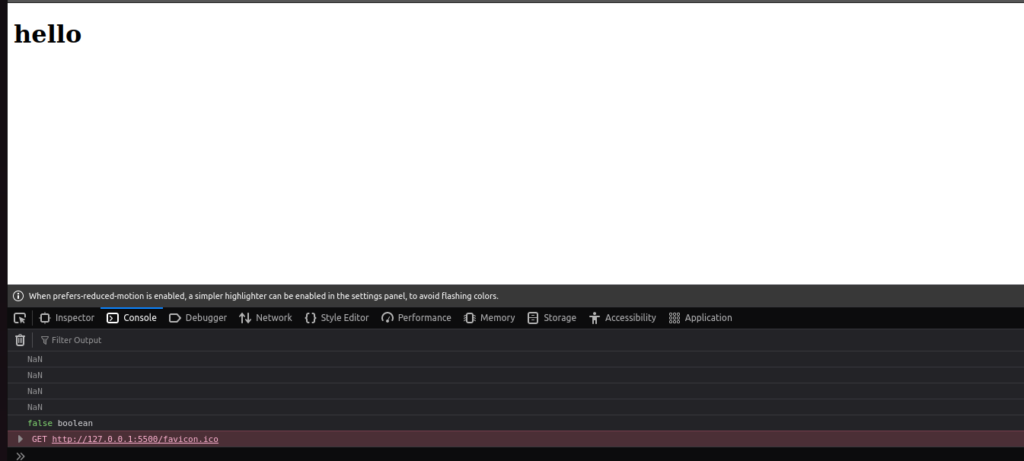
Later on, this code will determine which, and which is not a number. Overall, JavaScript has a lot of complexities, which is why it’s used for big websites for big companies.