Extends Feb 9, 2024. Friday Classwork
After making the initial window in the last post, it was time to add a background. I made the functions ‘tick’ and ‘render’. While I have not done anything with ‘tick’ just yet, I did work with render.
In Game.java
public void run() {
long lastTime = System.nanoTime();
double amountOfTicks = 60.0;
double ns = 1000000000 / amountOfTicks;
double delta = 0;
long timer = System.currentTimeMillis();
int frames = 0;
while(running) {
long now = System.nanoTime();
delta += (now - lastTime) / ns;
lastTime = now;
while(delta >= 1) {
tick();
delta--;
}
if(running)
render();
frames++;
if(System.currentTimeMillis() - timer >1000) {
timer += 1000;
System.out.println("FPS: " + frames);
frames = 0;
}
}
stop();
}
private void tick() {
}
private void render() {
BufferStrategy bs = this.getBufferStrategy();
if(bs == null) {
this.createBufferStrategy(3);
return;
}
Graphics g = bs.getDrawGraphics();
g.setColor(Color.black);
g.fillRect(0, 0, WIDTH, HEIGHT);
g.dispose();
bs.show();
}
public static void main(String args[]) {
new Game();
}
}
Now, my window has a background! and thanks to the setColor function, I can make it whatever color I want. In this particular instance, I made it black.
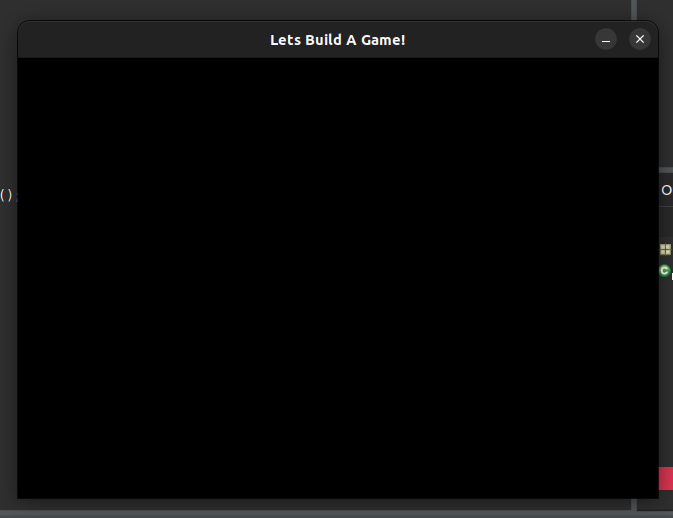
I can even make it blue, if I so please
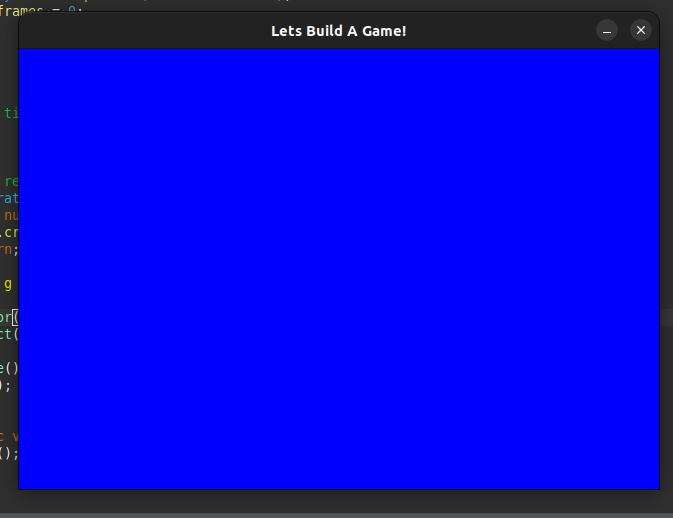
Now that we have our window finished for the most part, we are moving on to the rendering. To do this, we added classes GameObject.java and Handler.java. These classes will help show objects on the screen when we make them and their attributes.
Of course, this means we will need a system to tell what is an enemy, the player, or other objects such as coins or picking up power-ups, for example. For this, we add an Enum, short for Enumeration. We name it ID.java.
In ID.java
package com.tutorial.main;
public enum ID {
Player(),
Enemy();
}
This adds the IDs we will need so far, so we can tell apart Player and Enemy when adding functions to them.
We begin to add code for these, making Player.java. Guessing by the name, what we put in here should be specific and apply only to object with the class of Player
In Player.java
package com.tutorial.main;
import java.awt.Graphics;
public class Player extends GameObject{
public Player(int x, int y, ID id) {
super(x, y, id);
}
public void tick() {
}
public void render(Graphics g) {
}
}
Also, we have done code to give attributes in general for object, attributes all objects will need to generally have.
In GameObject.java
package com.tutorial.main;
import java.awt.Graphics;
public abstract class GameObject {
protected int x,y;
protected ID id;
protected int velX, velY;
public GameObject(int x, int y, ID id) {
this.x = x;
this.y = y;
this.id = id;
}
public abstract void tick();
public abstract void render(Graphics g);
public void setX(int x) {
this.x = x;
}
}
These set up the base velocity, or speed, that the object will go, as well as it’s ID.
Leave a Reply