Extends Feb 2, 2024 , Friday Classwork
After a lot of code, and confusion, I finally managed to OPEN A WINDOW!!
in Game.java
package com.tutorial.main;
import java.awt.Canvas;
public class Game extends Canvas implements Runnable{
private static final long serialVersionUID = 1550691097823471818L;
public static final int WIDTH = 640 , HEIGHT = WIDTH / 12*9;
public Game(){
new Window(WIDTH, HEIGHT, "Lets Build A Game!", this);
}
public synchronized void start() {
}
public void run() {
}
public static void main(String args[]) {
new Game();
}
}
and in Window.java
package com.tutorial.main;
import java.awt.Canvas;
import java.awt.Dimension;
import javax.swing.JFrame;
public class Window extends Canvas{
private static final long serialVersionUID = -240840600533728354L;
public Window(int width, int height, String title, Game game) {
JFrame frame = new JFrame(title);
frame.setPreferredSize(new Dimension(width, height));
frame.setMaximumSize(new Dimension(width, height));
frame.setMinimumSize(new Dimension(width, height));
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.setLocationRelativeTo(null);
frame.add(game);
frame.setVisible(true);
game.start();
}
}
All of this, just to make. to make…
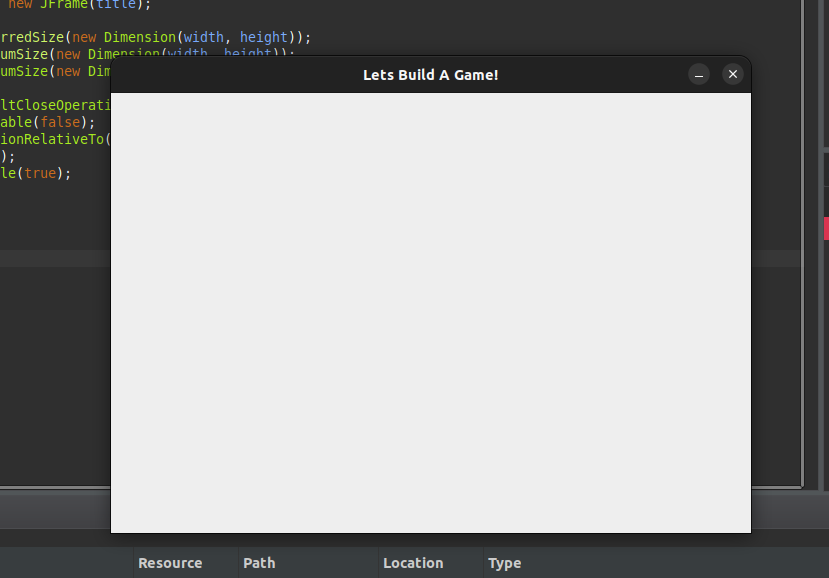
This little guy, this silly little box. I can already tell this is going to be a long ride, and I’ve got my work cut out for me.
After following the tutorial a bit more (15:07 left off), I managed to get some of the run code
In Game.java (No changes made to Window.java)
package com.tutorial.main;
import java.awt.Canvas;
public class Game extends Canvas implements Runnable{
private static final long serialVersionUID = 1550691097823471818L;
public static final int WIDTH = 640 , HEIGHT = WIDTH / 12*9;
private Thread thread;
private boolean running = false;
public Game(){
new Window(WIDTH, HEIGHT, "Lets Build A Game!", this);
}
public synchronized void start() {
thread = new Thread(this);
thread.start();
running = true;
}
public synchronized void stop() {
try {
thread.join();
running = false;
}catch(Exception e) {
e.printStackTrace();
}
}
public void run() {
long lastTime = System.nanoTime();
double amountOfTicks = 60.0;
double ns = 1000000000 / amountOfTicks;
double delta = 0;
long timer = System.currentTimeMillis();
int frames = 0;
while(running) {
long now = System.nanoTime();
delta += (now - lastTime) / ns;
lastTime = now;
while(delta >= 1) {
tick();
delta--;
}
if(running)
render();
frames++;
if(System.currentTimeMillis() - timer >1000) {
timer += 1000;
System.out.println("FPS: " + frames);
frames = 0;
}
}
stop();
}
public static void main(String args[]) {
new Game();
}
}
According to the video, this is the popular way with Java to run a game:
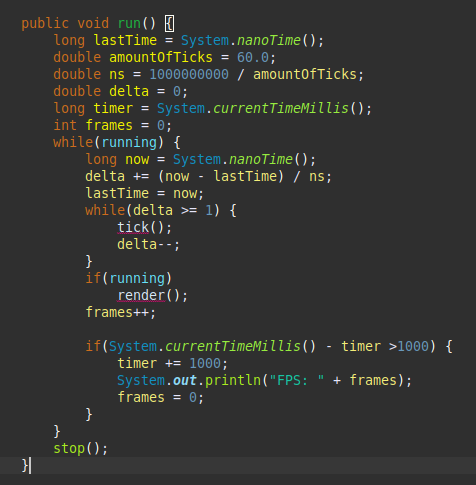
And it is often recommended to run it this way.
This is the basic setup code to help run and end the game when it is started. Obviously, 2 functions need to be made for it to properly work just yet. Based on the names, it is likely to be about the frames and refresh rate.
However, I am out of time here. I didn’t get very far, but as this tutorial is not really for beginners, so I suppose it makes sense that I would be pausing a lot 🙁
Leave a Reply