Today I’m followed Chris Courses’s tutorial to do a multi-room platformer game with Javascript and HTML Canvas. I create a canvas and draw a red box, which is the player character, it can move down and cause it to stop moving down when it stands at the bottom of the canvas.
To begin, I downloaded the Live Server app on visual studio code. And create a “index.html” file, set the background of the website is black and create a canvas. And also create a “index.js” file, and link it to the “index.html”.
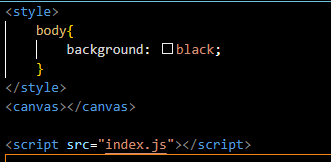
You will get a website like below:
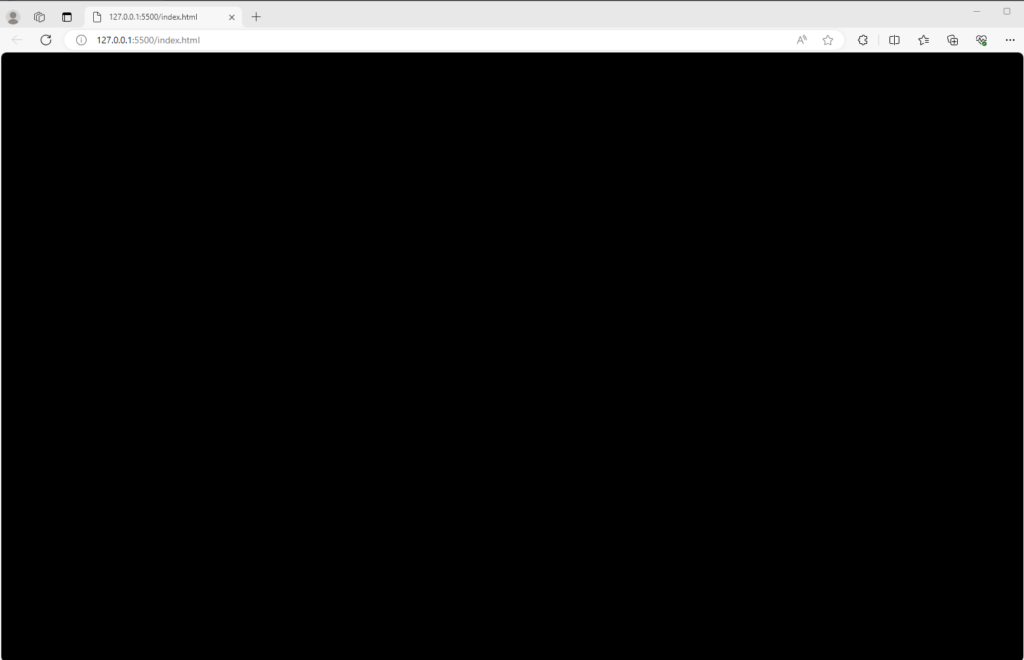
Next in the “index.js” file, make the canvas white and its width is 1024, height is 576.
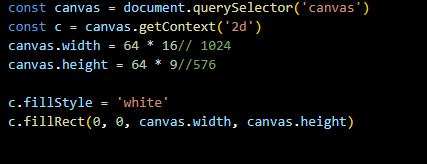
The canvas is drawn on the screen:
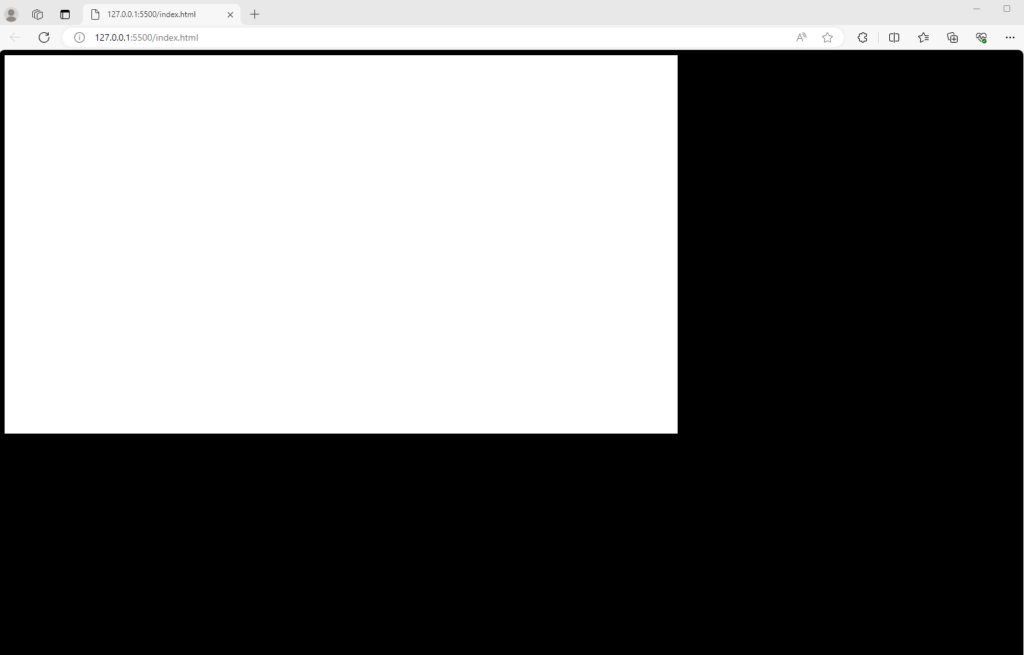
In the “index.js” file, I create a function called animate(). In this function, use the requestAnimationFrame() method to make a loop for the animation. That means we call a function that calls itself and this method allows the animations to run more smoothly. And draw a red square, which is the player, at (100, 100) and make it size is (100, 100).
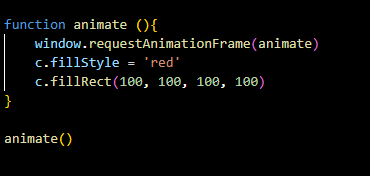
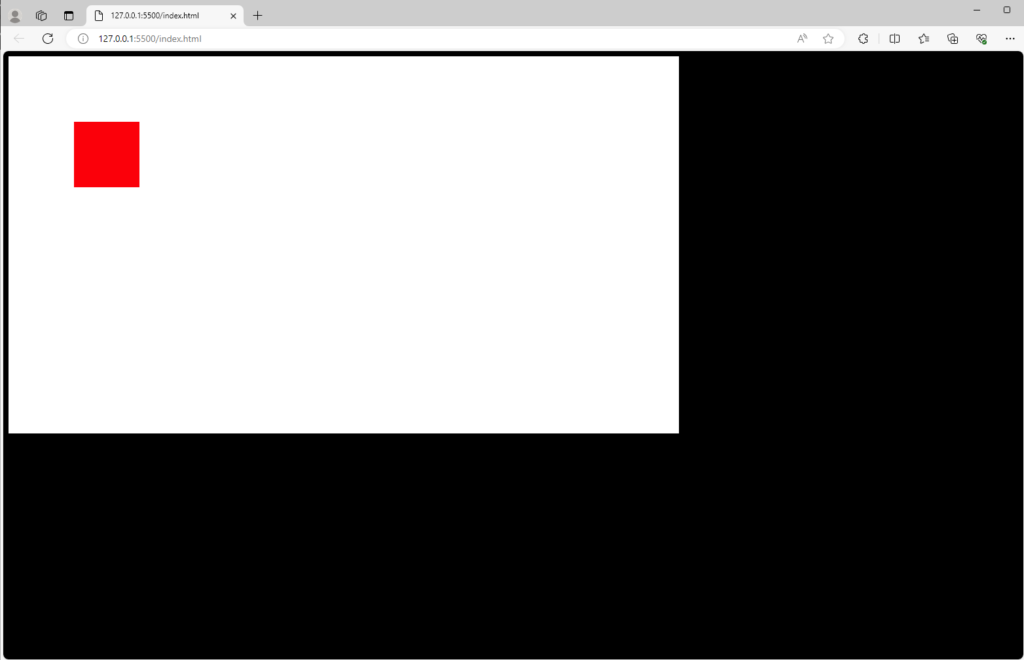
To make the red box move down, we just make change on its y-coordinate. Create a “y” variable is 100 and replace it on the second argument of the fillRect() method. And after it draw a square, make the “y” added 1.
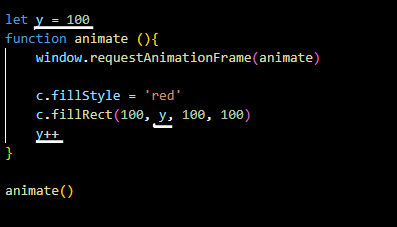
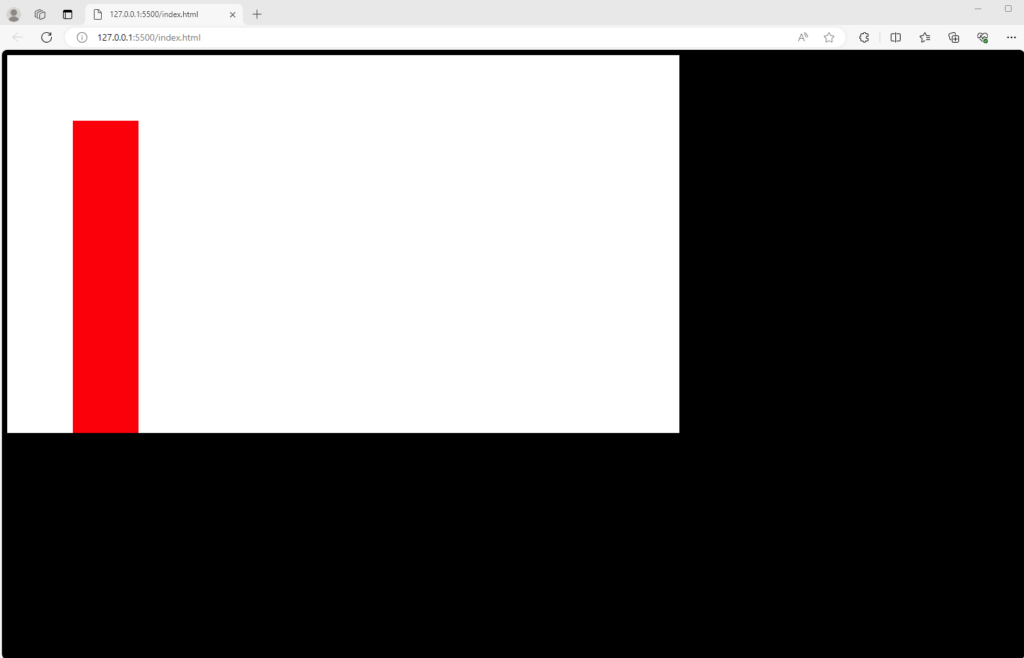
The box is moving down but the previous box is still on the screen. So to fix that, just move the method that creates the white canvas into the animate() function. It will draw the white canvas and the red box again. So the previous box will not appear on the screen.
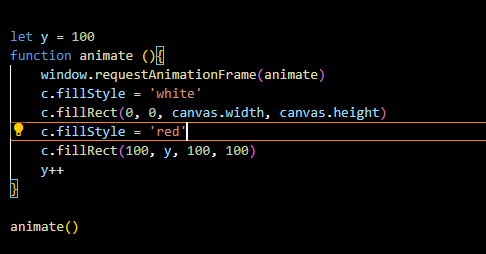
![]() | ![]() |
The red box is moving down out of the screen, to make it stand on the bottom of the canvas and stop moving down. Create a “bottom” variable is “y” plus 100 and write an if-elif statement that checks if the “bottom” is smaller than the white canvas height, add the “y” with 1 and “bottom” is “y” plus 100.
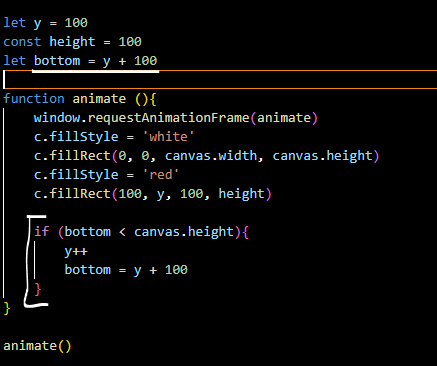
After run the code, the box stops moving down when it is at the bottom of the canvas:
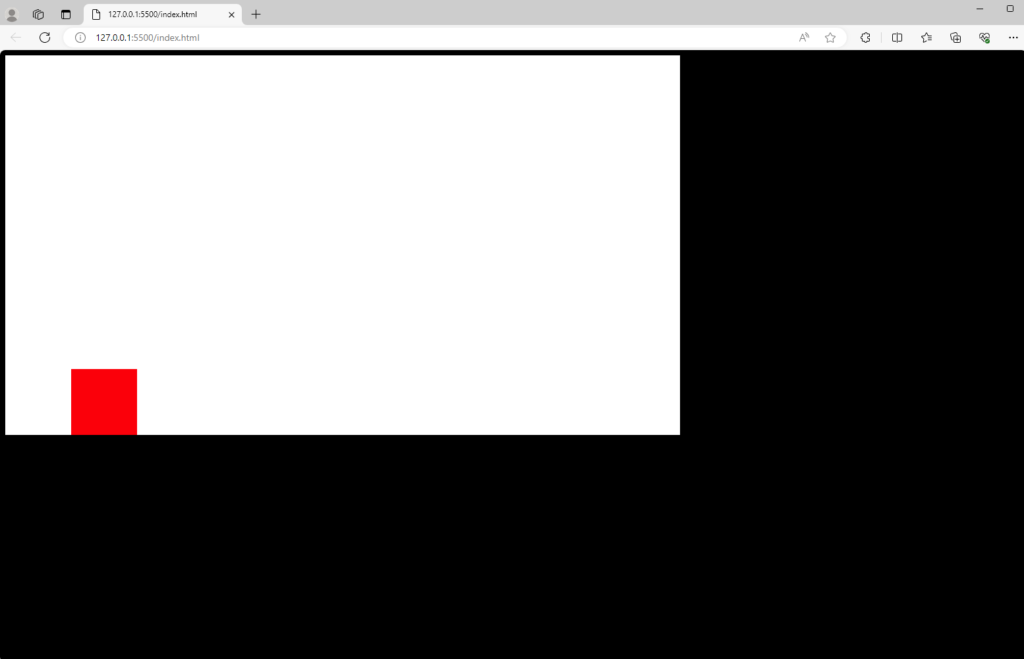
To make the code easy to organize. Create a Player() class, and use the constructor() method to construct an object and assign values to the object’s members. And also create a draw() function, which draws the red box; update() function, which is to make the box move and make it stop when it is at the bottom of the canvas. And call them in the animate() function.
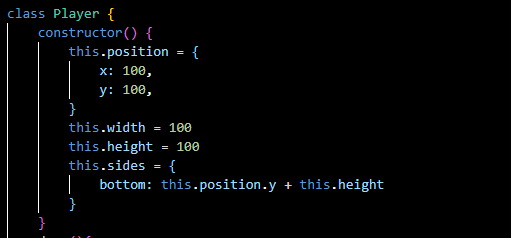
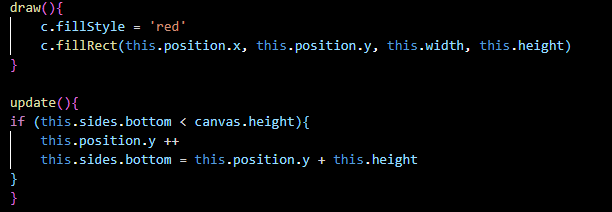
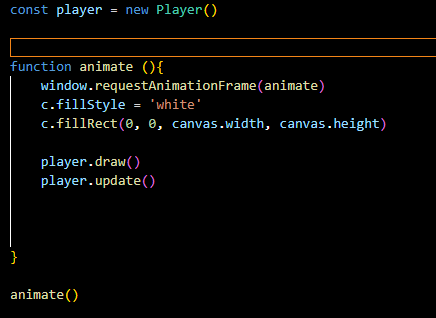
Next create a “JS” folder, in this folder create a “classes” folder, and create a “Player.js” file in the “classes” folder. Move the Player() class from the “index.js” to the “Player.js” to easily organize the code. And link the file in the “index.html”.
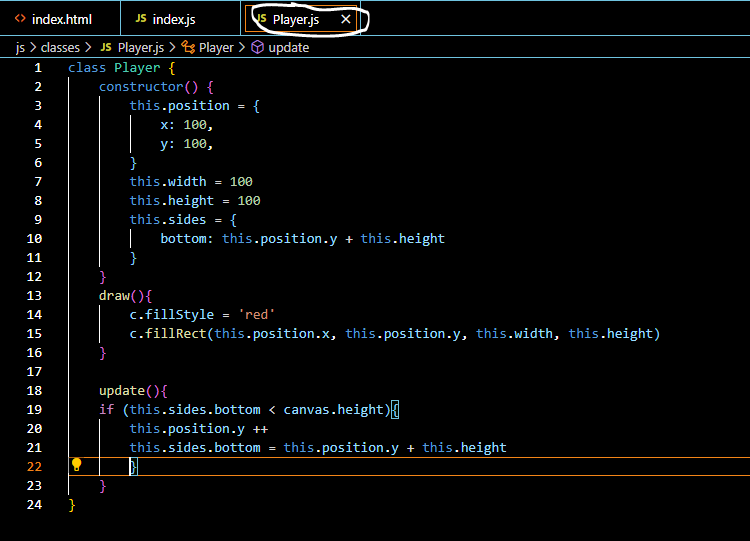
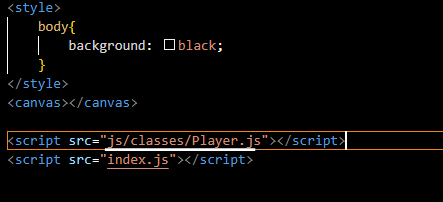
No Responses