Continuing from the previous post, I added the command for the bird to jump, automatically create new pipes, and move left while the game is playing. I also generated random highs for the pipes so the game wouldn’t be too boring.
Jumping
First create a new script called BirdScript and open it. In the BirdScript, create a RigidBody2D called myRigidbody and create a float (which is the box you can input the number in the unity app) called flapStrength.
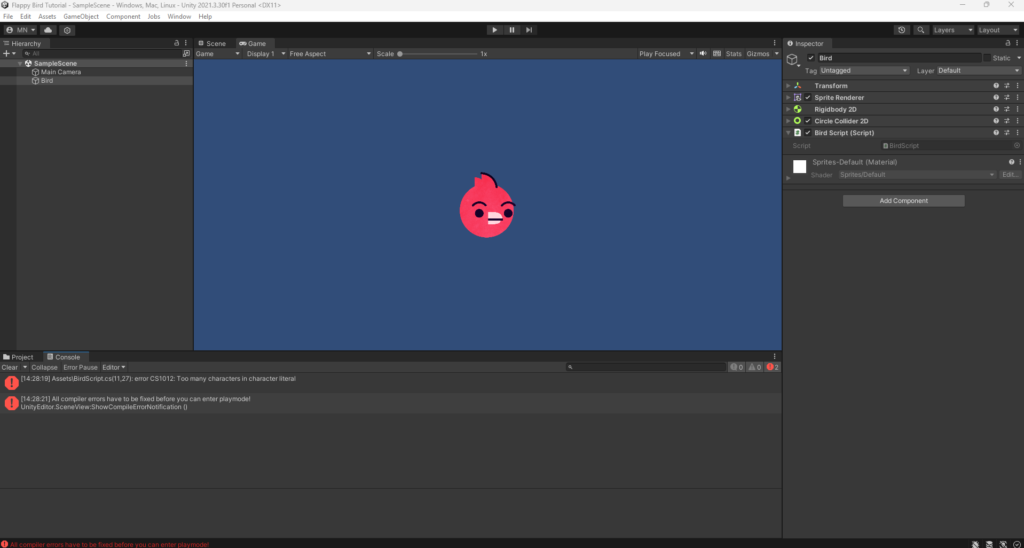
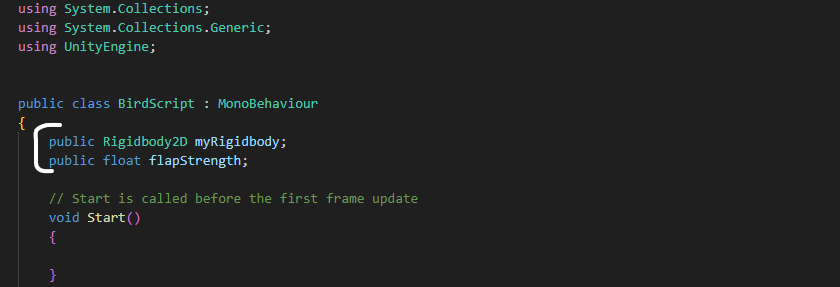
As you can see below, My Rigidbody and Flap Strength are in the BirdScript. Now to can take the bird’s velocity in the RigidBody2D, move the RigidBody2D into the My RigidBody.
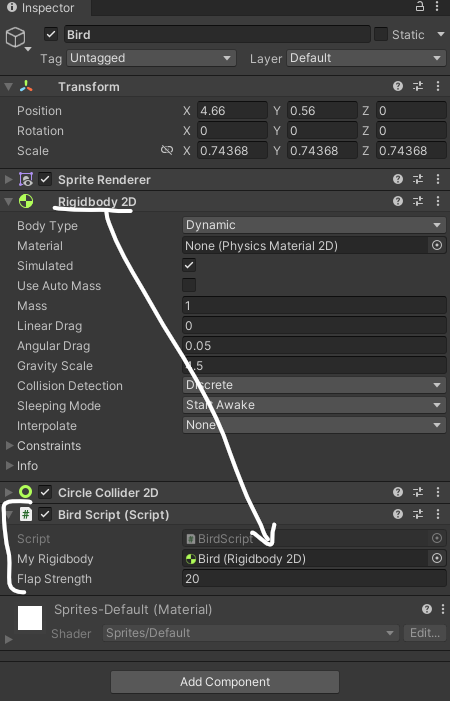
Then write if statement to check if the keydown is Space, myRigidBody’s velocity (which is the bird’s velocity) equal the Vector2.up times flapStrength. The Vector2.up is (0, 1), example if flapStrength is 5, Vector2.up times flapStrength is (0, 1) times 5 equal (0,5). So the bird’s y-coordinate go up 5.
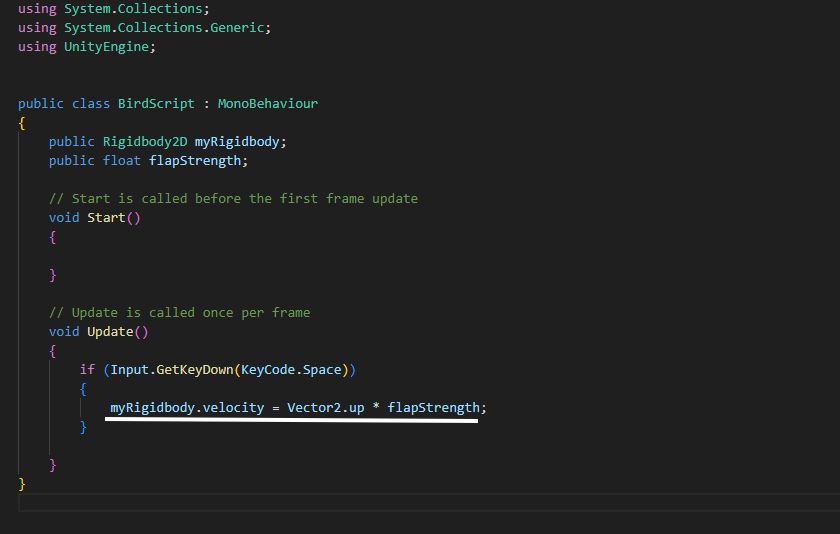
The Pipe
Create a new gameObject named Pipe, then create the top gameObjects of the pipe inside the Pipe.
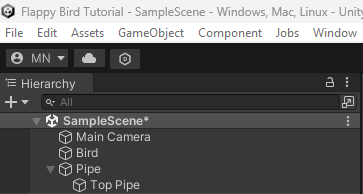
In Top Pipe, added new components are Sprite Renderer and Box Collider 2D. And move the image of the pipe in the project panel to the Sprite in the Sprite Renderer. And you can see the top pipe is on the screen.
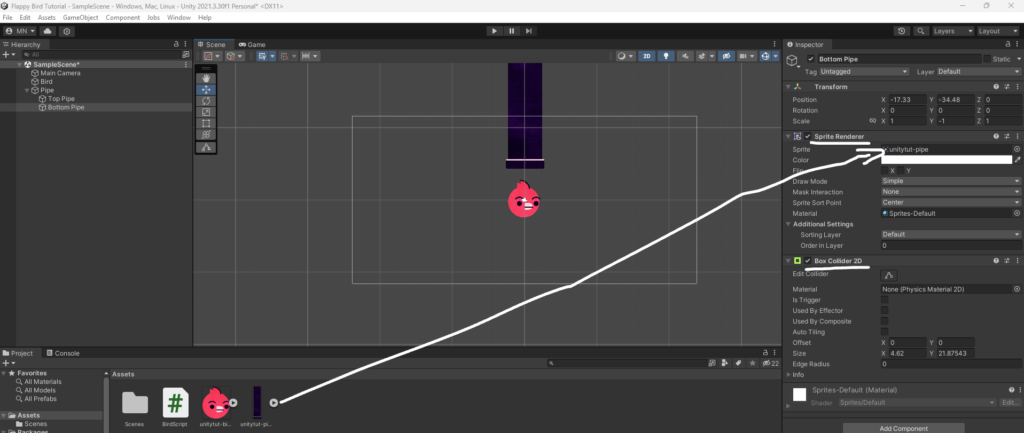
Then just duplicate the Top Pipe and change its name to Bottom Pipe.
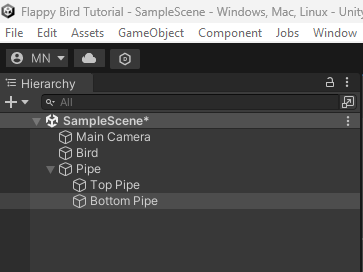
And change the y of the scale in the transform of the Bottom Pipe to -1. Then the pipe is on the screen now.
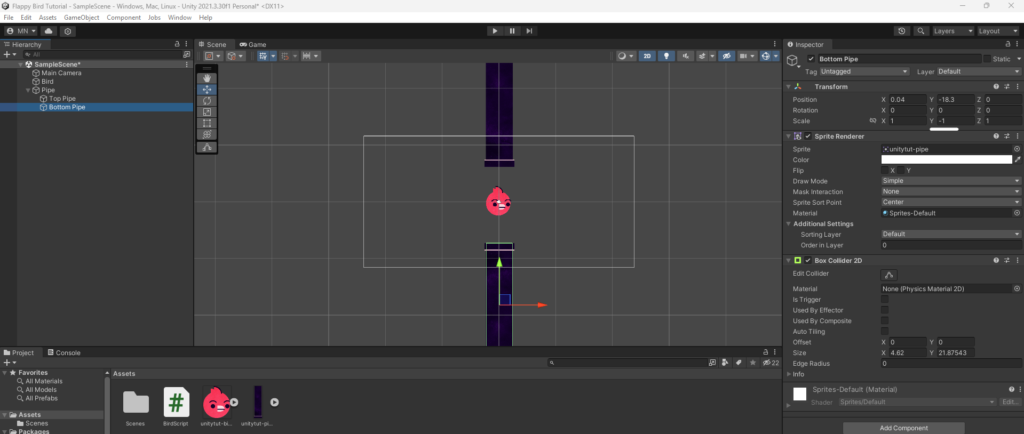
Moving Pipe
In the Pipe gameObject, add a script called Pipe Move Script and open it.
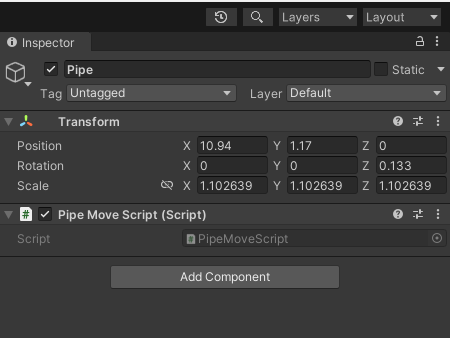
Create a float named moveSpeed of 5 and Transform.position equal to Transform.position (current coordinates) plus Vector3.left multiplied by MoveSpeed. Vector3.left is (-1, 0, 0) which is (x, y, z) and multiplied by the moveSpeed which is (-5, 0, 0) and added to the current position. So the pipe’s x coordinate will move back 5 units each time.
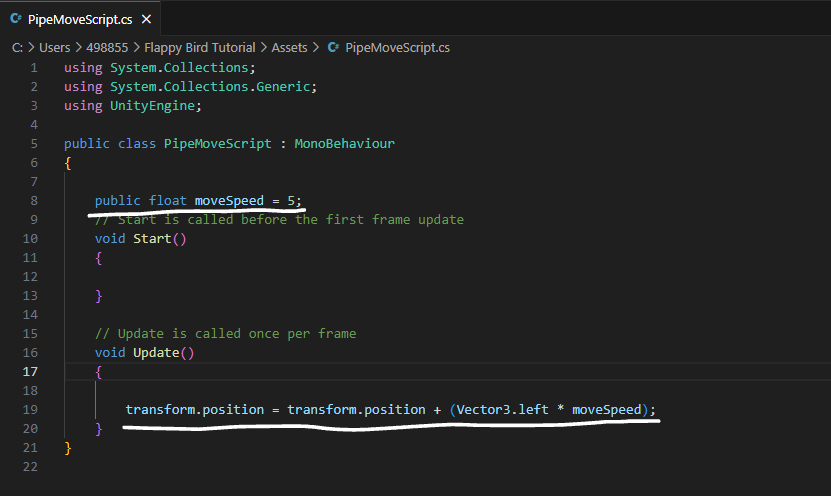
As you can see above, the pipe’s movement is quite fast. So to fix this problem, just multiply all the moves left command by Time.deltatime. The Time.deltatime is a property in Unity that measures the time in seconds between the current frame and the previous frame. It makes the game run smoother.
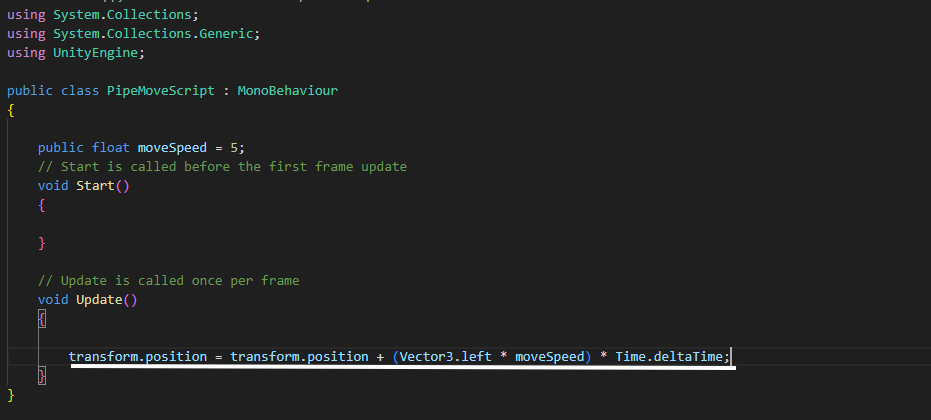
Pipe Spawning
Move the Pipe gameObject into the project panel and delete it in the hierarchy panel.Then create a new gameObject called Pipe Spawner.
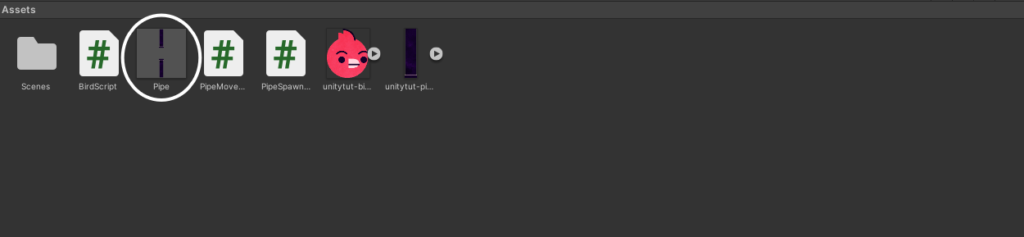
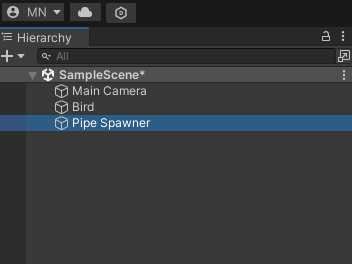
And create a new script called Pipe Spawn Script. In this script, create a new gameObject called pipe.Then move the Pipe in the project panel into the Pipe gameObject we just created in the Pipe Spawn Script.
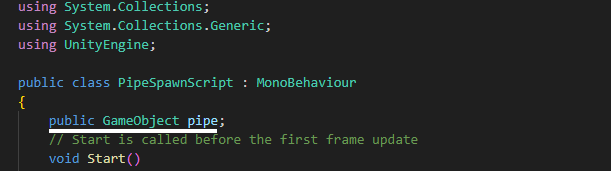
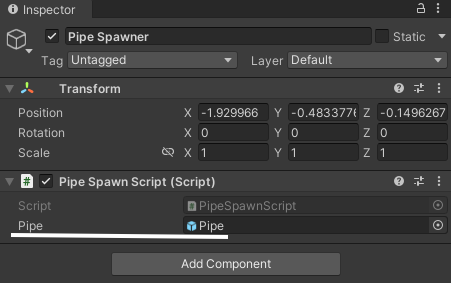
And the last step to make it automatically create new pipes is to use the instantiate() command. The instantiate creates new objects at runtime, also known as “spawning”. In the Pipe Spawn Script, add a command line: instantiate(pipe, transform.position, transform.rotation).
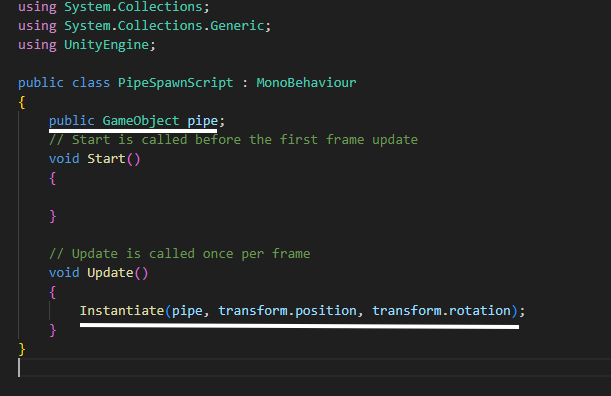
The spawn was successful but we got a new problem where it appeared in every frame. To fix this problem, creating a new float called the spawnRate is 2 and the timer is 0. And use if statement to check if the timer is less than spawnRate, then the timer will add to Time.deltatime. And otherwise it creates the pipe and the timer is 0.
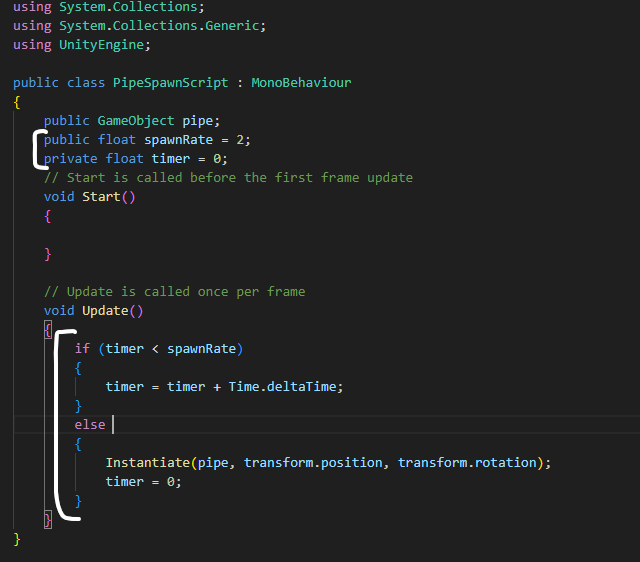
Our spawning was successful now, but when the game starts we have to wait a bit for the pipe to appear, so we want it to have a pipe available from the start and create new pipes automatically. So create a new void (same as the function in python) called spawnPipe(). Move the command that creates the pipe into that function. And called that again in the Start() and Update() function.
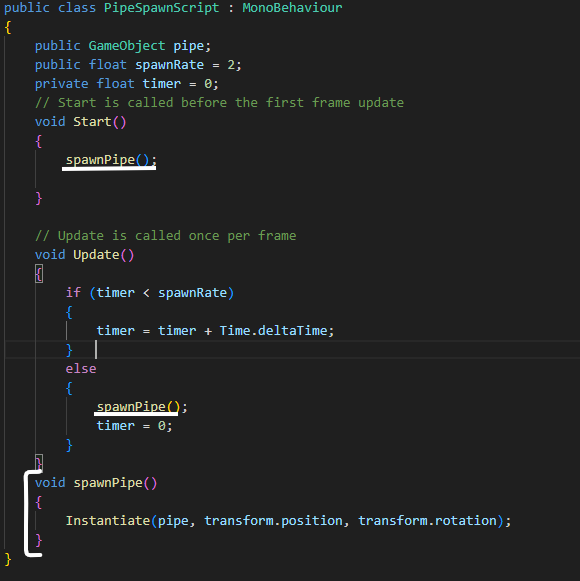
Height of the Pipe
To make the game look more like Flappy Bird, I made the pipe have random highs. Create a float called heightOffset is 10. And in the spawnPipe() function, make the lowestPoint the pipe’s y coordinate minus the heightOffset, and make the highestPoint the pipe’s y coordinate plus the heightOffset. And in the command line that creates the new pipe, change transform.position to new Vector3(transform.position.x. Random.Range(lowestPoint, highPoint), 0). Vector3 is (x, y, z), so it only changes y coordinates the random number between the lowestPoint and the highestPoint.
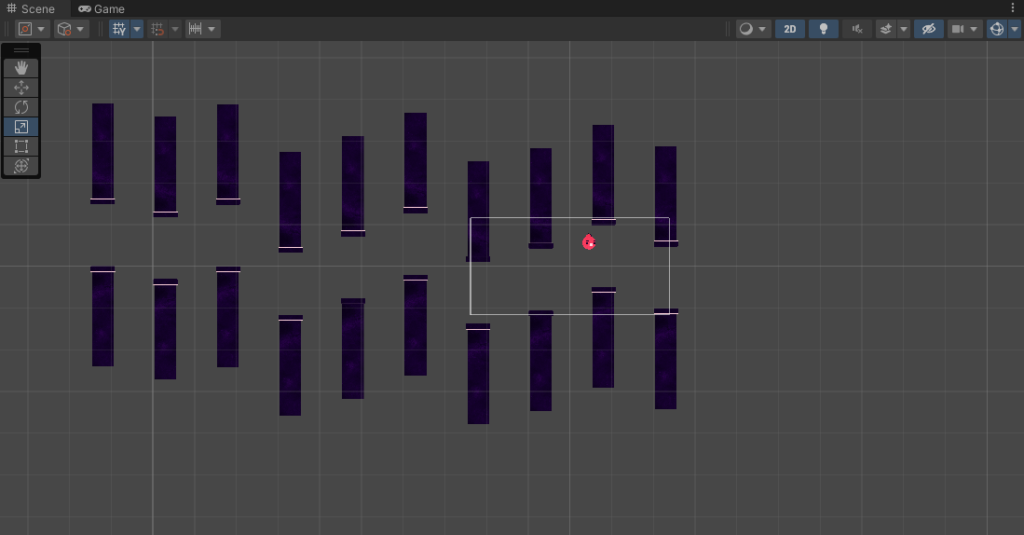
These are the ones I made while watching Game Maker Toolkit’s video tutorial, I will try to complete this tutorial in the next post.
No Responses