Continue from my last blog post, I made the player’s health bar work. I also fixed a bug where, after the enemy collide with the player and was destroyed by the player’s attack, something was still pushing the player back. And I updated the code so the Game Over screen is now call from the player script instead of the enemy script.
In the Player Script
I realized I missed a step to get the player’s health bar working, which is using GetComponentInChildren() to determine the healthBar.

Once I added that, the player’s health bar is work.
However, I got a new error where the player gets pushed back after destroying the enemy. As you can see below.
To fix this, I change the player’s movement logic to use Rigidbody2D’s velocity instead of using transform.position. Because I realize that transform.position is to teleport the object by changing its position. Using RigidBody2D’s velocity is better because it controls how fast the object moves, making the movement smoother and more natural. So I deleted the player’s movement in Update().
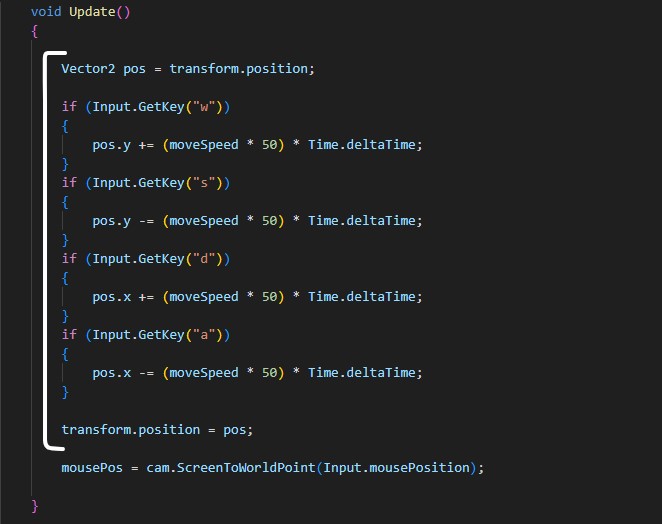
Next, I used the Vector2 to store the movement direction and Input.GetAxisRaw to detect whether the player is pressing movement keys. And applied the player’s input to the Rigidbody’s velocity.
- Input.GetAxisRaw(“Horizontal”) detects input from A/D keys or Left/Right arrow keys.
- Input.GetAxisRaw(“Vertical”) detects input from W/S keys or Up/Down arrow keys.
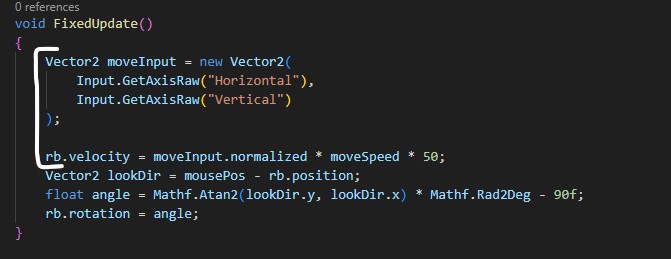
In the Enemy Script
Then in the CollisionEnter2D, before destroying the enemy object when its health reaches 0, I first stop its movement, disable physics to prevent force reactions, turn off collision, and then destroy the object.
Stop Movement
It sets velocity to zero to immediately stop any movement.
rb.velocity = Vector2.zero;
Disables Physics
When isKinematic is true, the RigidBody stops responding to physic forces, meaning it won’t be affected by gravity, collisions, or other rigid bodies.
rb.isKinematic = true;
Turn Off Collsion
The enemy can’t block the player’s movement or get hit by another attack after death.
GetComponent<Collider2D>().enabled = false;
Destroyed the Enemy Object
This removes the enemy from the game after 0.1 seconds.
Destroy(gameObject, 0.1f);
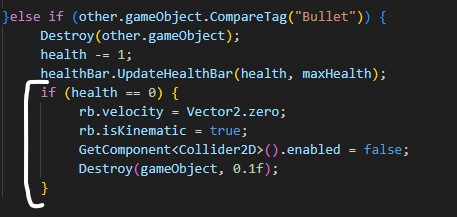
The problem is fixed.
In the Player Script
Next, I moved the logic for displaying the game over screen from the enemy script to the player script. I created a logic variable of type LogicScript in the script.
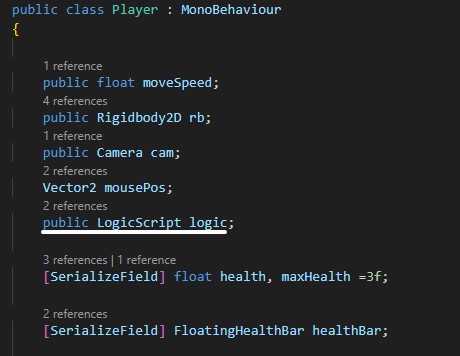
Then use GameObject.FindGameObjectWithTag(“Logic”) to find the GameObject with the tag “Logic”. And .GetComponent<LogicScript>() gets the LogicScript component attached to that GameObject.

Then call the gameOver() function in getEnemyDamage() function when the player’s health reaches 0.
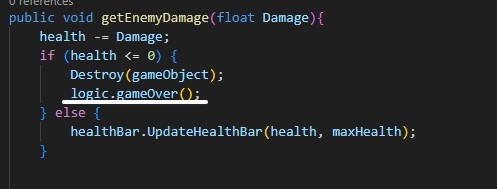
The Game Over screen appears when the player is dead.
No Responses