Today I made a Shooting game with Unity. I created a white box represent the player and set its movement. And make it possible to fire bullets when clicking on the screen.
Movement
In the assets, import the white box image and move it into the hierarchy. And change its name to Player.
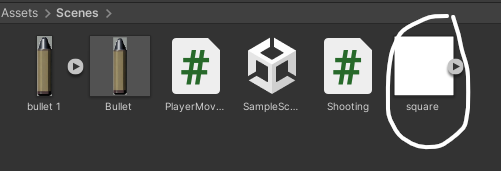
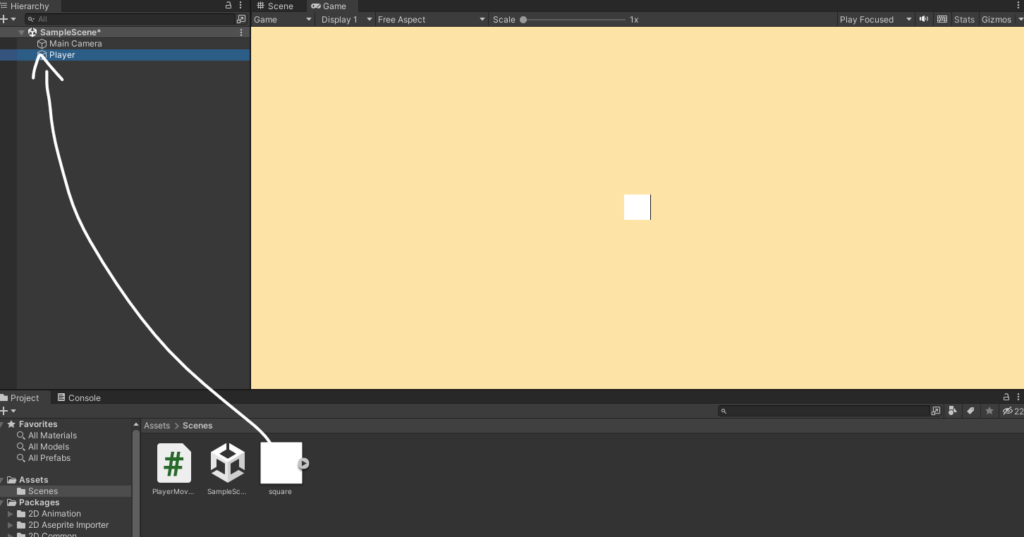
To be able to set the game object’s movement, we need to use the 2D Rigidbody component. In Unity, the Rigidbody 2D component controls the movement and rotation of game objects using the physics engine. So in the Player game object, add a 2D Rigidbody component and set it to Freeze Rotation. Freeze Rotation makes the player not rotate when hit by bullets.
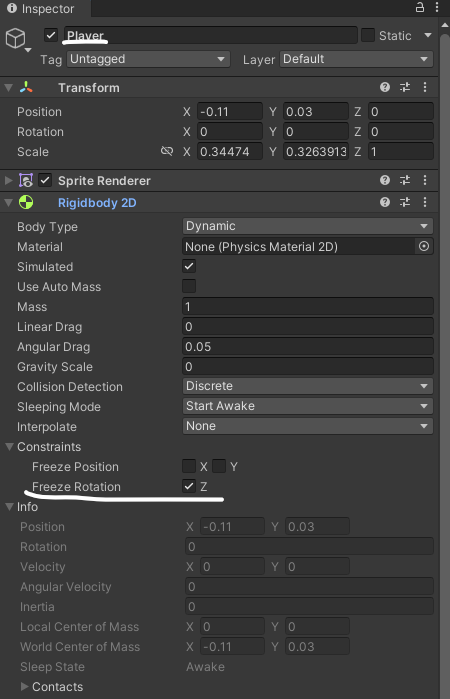
Create a new script called Player Movement. In this script, create a float variable called moveSpeed and a Rigidbody 2D called rb. And a Vector2 (a type of variable, like int, float, bool, string) variable called movement.
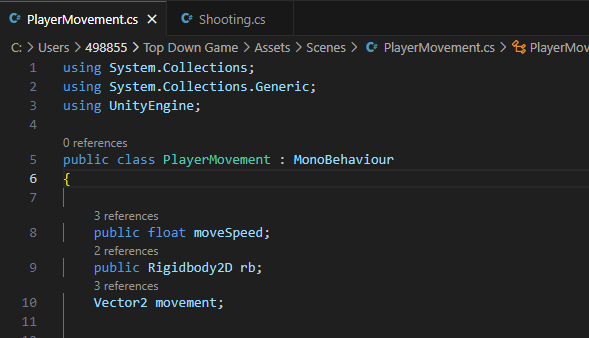
In the Update() function, the x coordinate movement is equal to Input.GetAxisRaw(“Horizontal”) and multiplied by moveSpeed. Input is allows your users to control your game or app using a device, touch, or gestures. GetAxisRaw returned the value in the range of -1 to 1 for the keyboard. So when the input is horizontal, it means the keyboard input is left and right (left is -1, right is 1), then it returns the value and multiplies it with the moveSpeed. Do the same thing with the y-coordinate of movement but it is vertical.
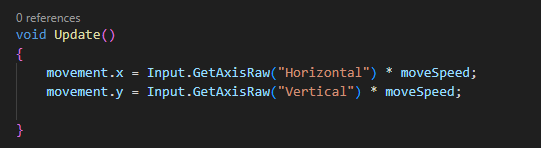
In the FixedUpdate() function, rb.MovePosition (rb.position + movement * moveSpeed *the Time.fixedDeltaTime).
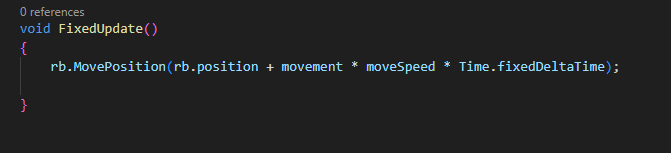
Example if the player’s position is (2, 3).
Step 1: Movement
- movement.x = 1 (because the right arrow key is pressed)
- movement.y = 0.
Step 2: Multiply by moveSpeed
- Horizontal movement: 1 * 5 = 5
- Vertical movement: 0 * 5 = 0
So the movement is (5, 0).
Step 3: Multiple by TimefixedDeltaTime
TimefixedDeltaTime is 0.02
- Horizontal movement: 5 * 0.02 = 0.1
- Vertical movement: 0 * 0.02 = 0
So the movement is (0.1, 0)
Step 4: Add to current position
- new x-coordinate: 2 + 0.1 = 2.1
- new y-coordinate: 3 + 0 = 3
So the player’s new position is (2.1 , 3).
Then control + s to save and go back to Unity. And set the move speed is 5 and move the Rigidbody 2D to rb.
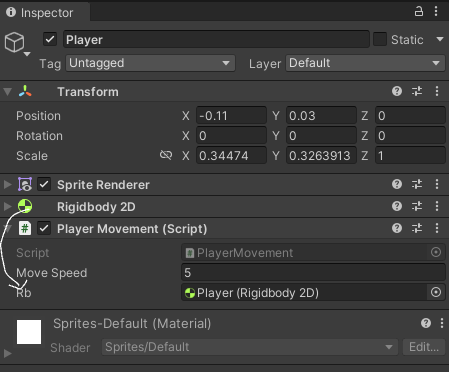
And now we can control the player.
Shooting
Create a game object called fire point. And then move it in front of the player.
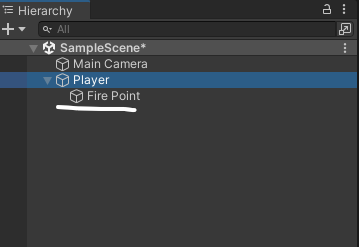
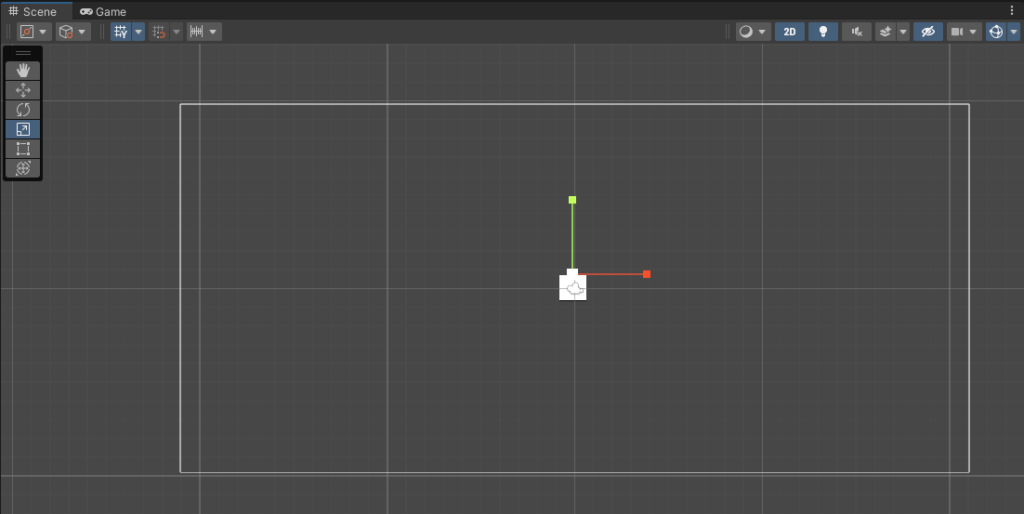
Next import a bullet image in the assets. And move it into the hierarchy.
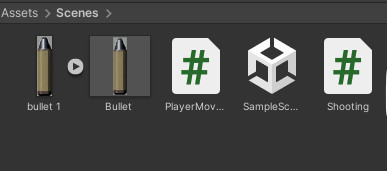
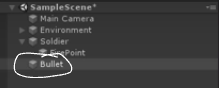
Move the Bullet in front of the player and change its size. Then add the Rigidbody 2D and Box Collider 2D. Change the gravity in the Rigidbody 2D to 0 and in the box collider, edit the collider to make it around the bullet. Then move the Bullet back to the assets and delete it in the hierarchy. Next is create a new script called shooting.
In the Shooting script, create a transform (determines the Position, Rotation, and Scale of each object in the scene) variable called firePoint. Create a GameObject variable called bulletPrefab and a float variable called bulletForce.
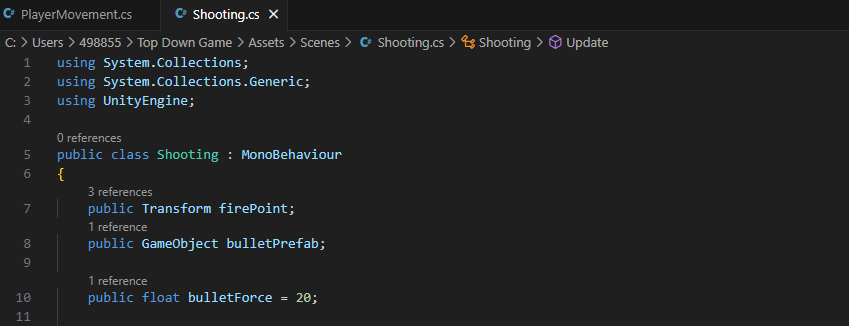
In the Update() function, it checks if Input.GetButtonDown(“Fire1”). Fire1 is a button name and it is the left mouse button. So this function checks if the button pressed is left mouse button, then call the Shoot() function.

In the Shoot() function, create a new GameObject called Bullet and it equals Instantiate(bulletPrefab, firePoint.position, firePoint.rotation). The instantiate is to create a new bullet in the game screen. BulletPrefab is the bullet image, firePoint.position is the position of the firePoint GameObject, and the firePoint.rotation is the rotation of the firePoint GameObject.
Next create a Rigidbody2D called rb and it equals bullet.GetComponent<Rigidbody2D>(). This command searches for the Rigidbody2D component attached to the bullet.
Then rb.AddForce(firePoint.up * bulletForce, ForceMode2D.impulse). This command applies a force to the bullet, causing it to move in a certain direction. The firePoint.up is (0, 1) and it is multiplied by bulletForce, and ForceMode2D.Impulse is the force that is applied instantly in a single burst, causing immediate acceleration.

Back in Unity, move the Shooter script to the player. Move the Fire Point game object to the fire point in the Shooting script, and also move the Bullet game object to the Bullet Prefab.
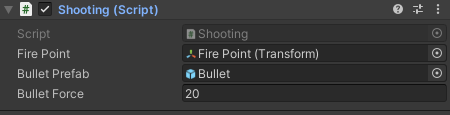
You can now move the player with the arrow keyboard and make it fire when clicking on the screen.
No Responses