Continuing from my previous post, today I added an enemy and made it chase the player. And the player will die if it collides with an enemy and the enemy will disappear when the bullet touches it. I also added health for the enemy. If I set the enemy health is 3, the enemy will disappear when the bullet collides with it 3 times.
Enemy
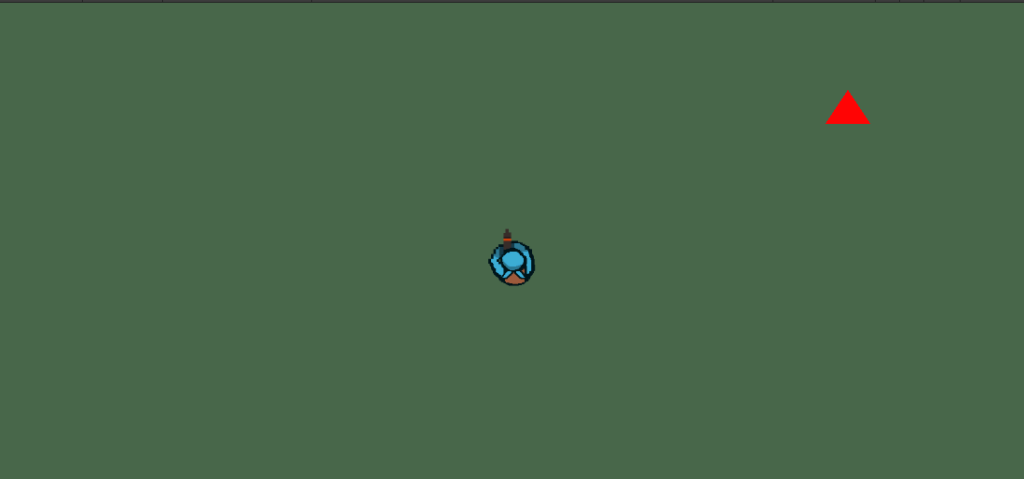
To create an enemy like image above, create a new game object named Enemy, then add the Sprite Renderer component. As for the enemy image, since I haven’t drawn the enemy yet, I just made it as a triangle and its color is red. We can change the enemy image later.
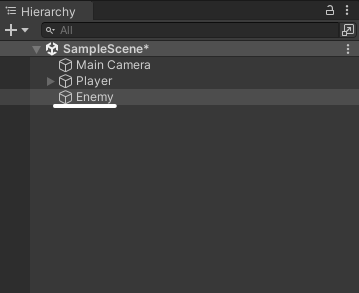
Enemy Rotate
In the Enemy game object, add Rigidbody 2D, Polygon Collider 2D, and an Enemy script. In the Rigidbody 2D, change the body type to Kinematic. In Unity, Kinematic is the body moves under simulation, but and isn’t affected by forces like gravity.
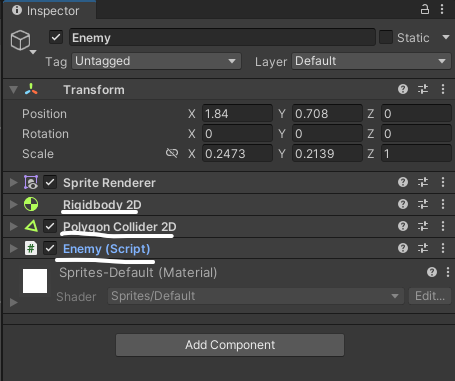
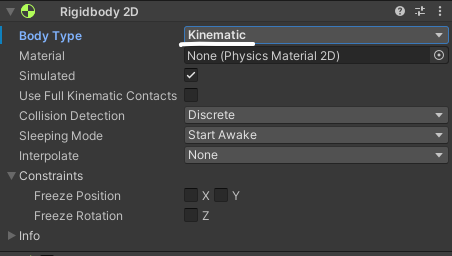
In the Enemy script, create a target in transform type. The Transform component determines the Position, Rotation, and Scale of each object in the scene. Then create speed and rotateSpeed variable with float type. And a rb variable with Rigidbody2D type.
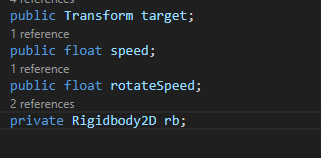
Create a Start() function, and rb equal GetComponent<Rigidbody2D>. This command mean it will find the component is Rigidbody2D in Enemy game object and rb is equal that component. We will use the rb to change the enemy position later.

Then in the Update() function, use the if-else statement to check if it not have the target then call the GetTarget() function and otherwise call the RotateTowardsTarget() function.
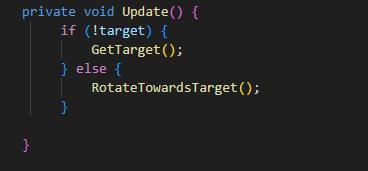
GetTarget() function is made the target equal the Player game object by using the FindGameObjectWithTag(“Player”).transform command. It will take all information of player, like position.

And the RotateTowardsTarget() function is find the angle to make the enemy rotate follow the player.

After you run the game, the enemy now is rotate follow the player.
Chasing Enemy
Create a new function called Fixedupdate(), and rb.velocity (the enemy’s velocity) equals the transform.up times speed.
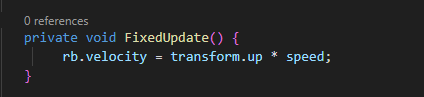
As you can see above, the enemy is supposed to push the player away but it only rotates when it touches the player. So to fix this error, I added a Circle Collider 2D component and made its radius is 0.5 in the Player game object. Then the problem is fixed.
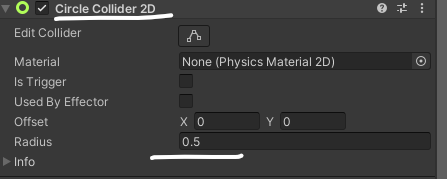
Destroy Player
Create an OnCollisionEnter2D (Collision2D other) function. OnCollisionEnter2D is a command that check other object collide with this object (enemy). And Collision2D other is other objects. In this function, use the if-else statement to check if other game object collide with enemy is Player then destroy the player and the enemy’s target is null.
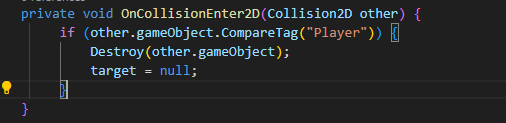
But when the player is destroyed, it has a new error shown in the console.
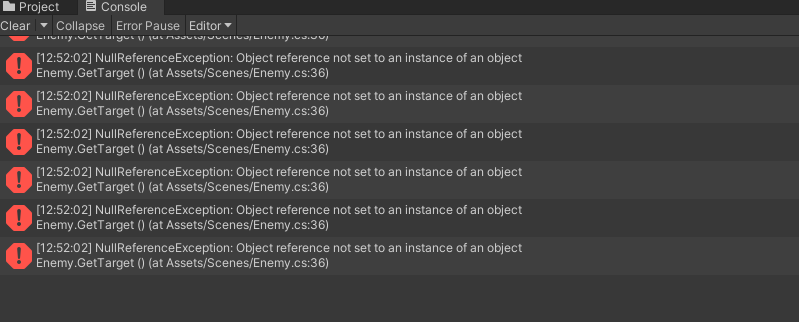
To fix this error, added if-else statement in the GetTarget() function. It checks if gameObject is Player then the enemy’s target is player.

The problem is fixed.
Bullet Collision
As you can see above, the bullet is not touching the enemy, it just goes through the enemy. In the Enemy game object, change the Body Type to Dynamic and its gravity to 0 in Rigidbody2D.
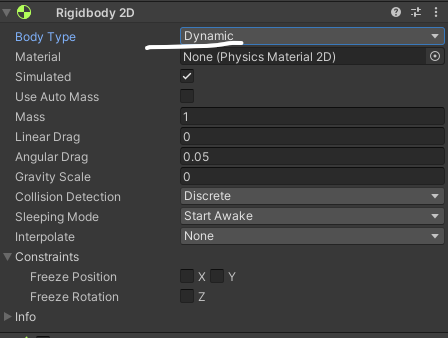
Destroy Enemy
In the Bullet game object, create a new tag named Bullet and set its tag to Bullet.
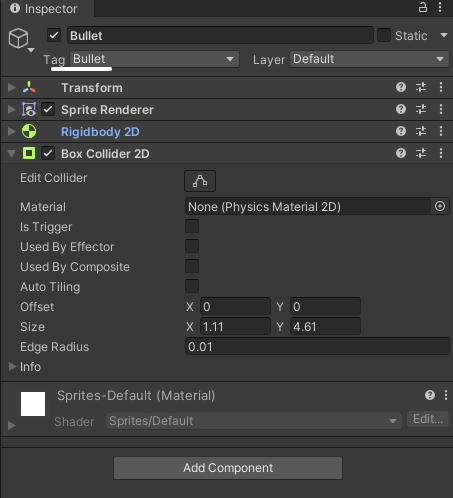
In the Enemy script, write a command that destroys the enemy and the bullet if they collide.
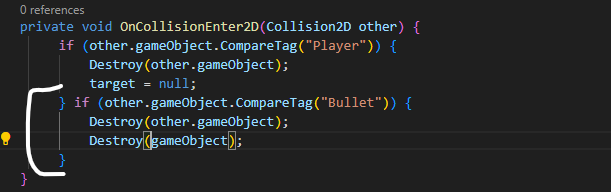
Enemy Health
Create an int variable called lives and it equals 3. And if the bullet touched the enemy then minus lives 1 and if the enemy’s lives equal 0 then destroy the enemy.

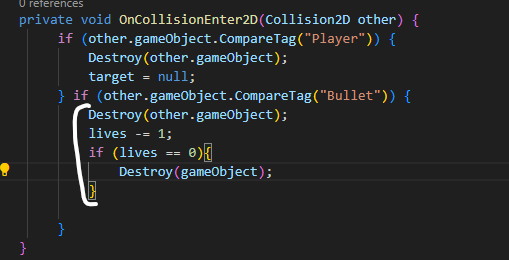
The enemy disappears when the player shoots it 3 bullets.
No Responses