I recently added an enemy spawning system to my top-down shooting game in Unity. Enemies now spawn from three different directions, making the game more challenging and requiring players to stay alert to attacks from multiple angles.
To set up enemy spawning, create a Spawner GameObject and an EnemySpawner script. In the script use the [SerializeField] to create variables that can be edit in the Inspector:
- spawnRate is a float variable set to 1f, which determines how frequently enemies spawn.
- enemyPrefabs is a GameObject array that holds the different enemy types you want to spawn.
- canSpawn is a bool variable set to true, indicating whether enemies can spawn or not.
The [SerializeField] attribute lets you keep variables private, but we can change the value of that variable in the inspector.
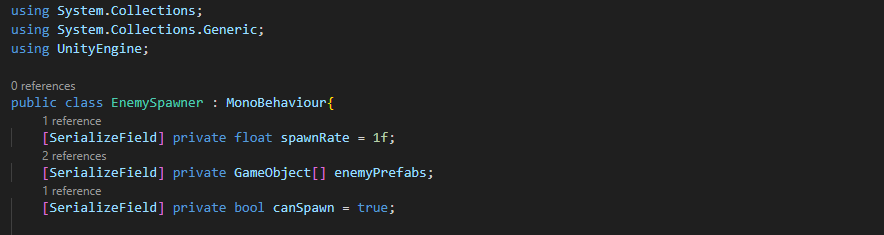
Next, create an IEnumerator method called Spawner. In this method, create a variable named wait and set it to WaitForSeconds(spawnRate). WaitForSeconds in the Unity function pauses the coroutine for a specified amount of time, allowing you to control how often enemies spawn based on the spawnRate variable.

Use a while loop to continuously spawn enemies. The while (canSpawn) condition means it will run as long as canSpawn is true. In this loop, use yield return wait the coroutine for the amount of time specified by the wait variable. Then create an integer variable called rand and set it to Random.Range(0, enemyPrefabs.Length), which will randomly select an index from the enemyPrefabs array. Next, create a GameObject variable named enemyToSpawn and set it to enemyPrefabs[rand]. Finally, use the command Instantiate(enemyToSpawn, transform.position, Quaernion.indentity) to create an instance of the selected enemy at the spawner’s position with no rotation. The Instantiate method is used to create a copy of the specified GameObject in the scene, allowing you to dynamically spawn enemies during gameplay.
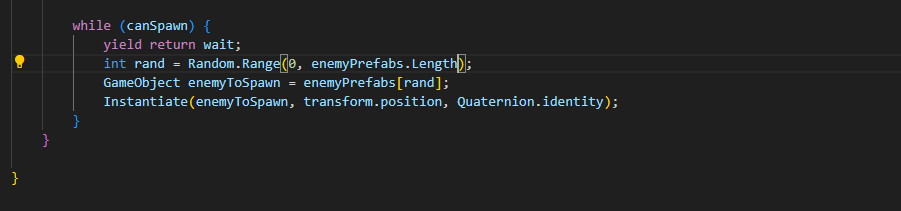
In the Unity Inspector, the Spawner now has three variables: spawnRate, enemyPrefabs, and canSpawn. We can change its value in the inspector.
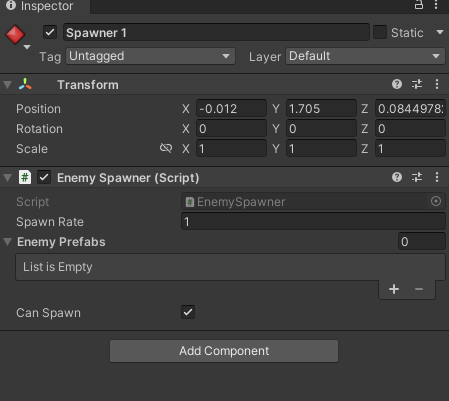
Now move the Enemy GameObject into the Enemy Prefabs. To make it can spawn the enemies.
You can duplicate the enemy and name it enemy 2 and move it to the Enemy Prefabs. So it can randomly spawn different types of enemy.
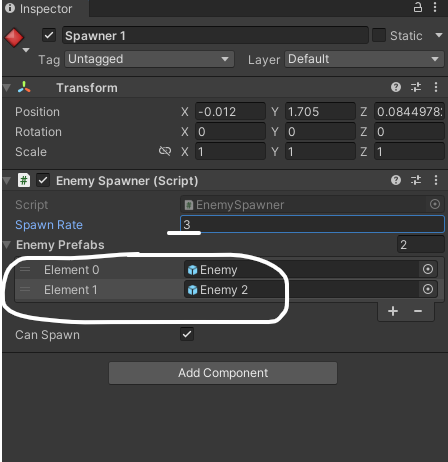
To spawn enemies from multiple directions, duplicate the Spawner GameObject and place the copies in three different locations. Each spawner will spawn enemies from its location, allowing for varied gameplay.
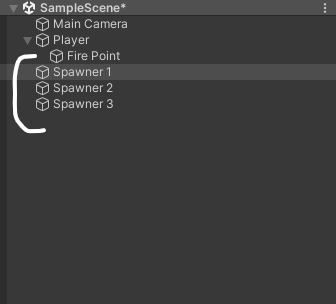
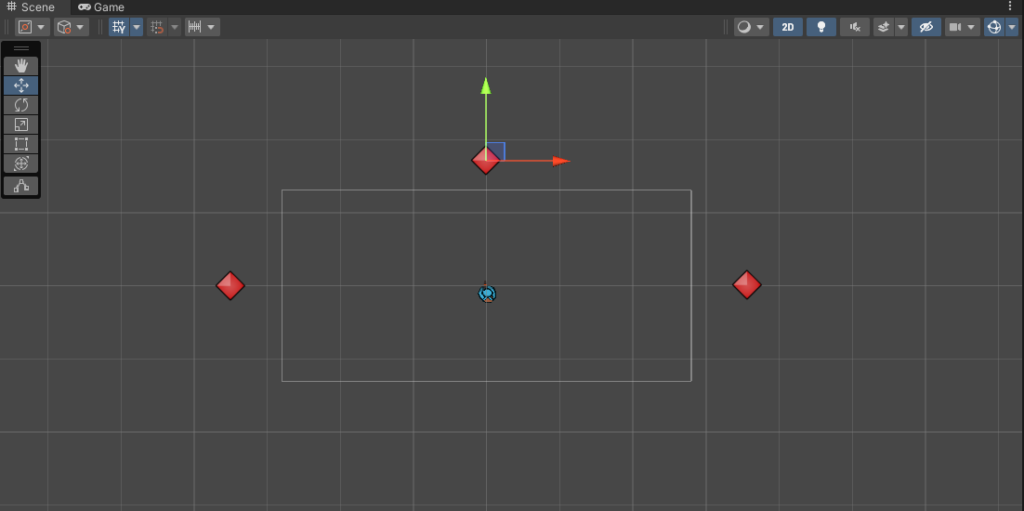
No Responses