Mario vs. Donkey Kong is a 2004 puzzle-platform game developed by Nintendo Software Technology and released for the Game Boy Advance. I followed LeMasterTech’s tutorial step by step to rebuild Classic Mario vs. Donkey Kong in Python using the Pygame module.
Setting Up A Dynamically Resizable Pygame Game
First step to create a game, we need to create a window game. This code creates a window, fills the background with black, and adds the title of the window, “Classic Donkey Kong Rebuild!”.
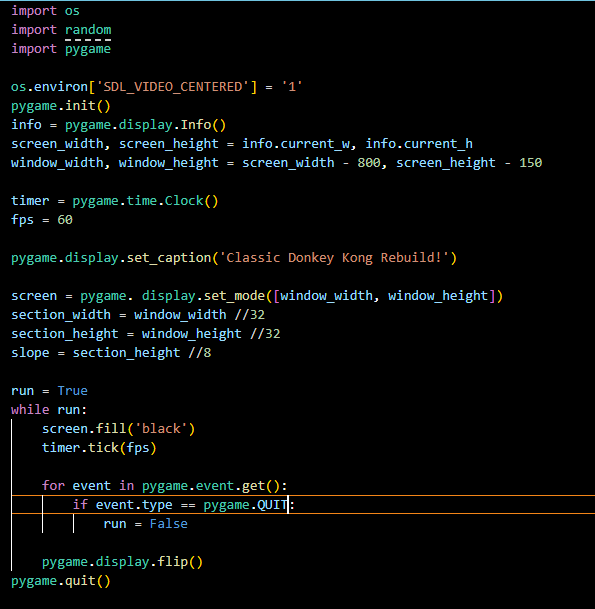
When you run this code, you’ll get a window like this:
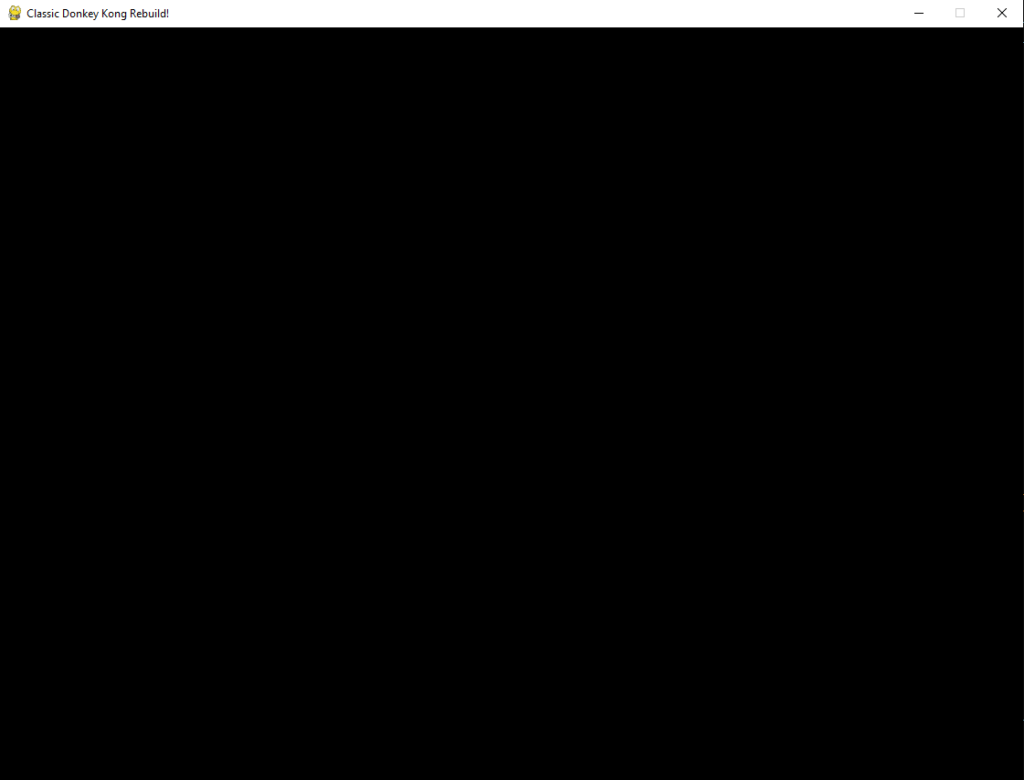
Setting Up A Class For Bridges And Platforms
Next we create a class Bridge, it needs 3 arguments(x_coordinate, y_coordinate, length of the bridge) to draw how the bridge looks. And in the draw_screen() function, we call the Bridge class and provide arguments, it will draw the brides into the screen.
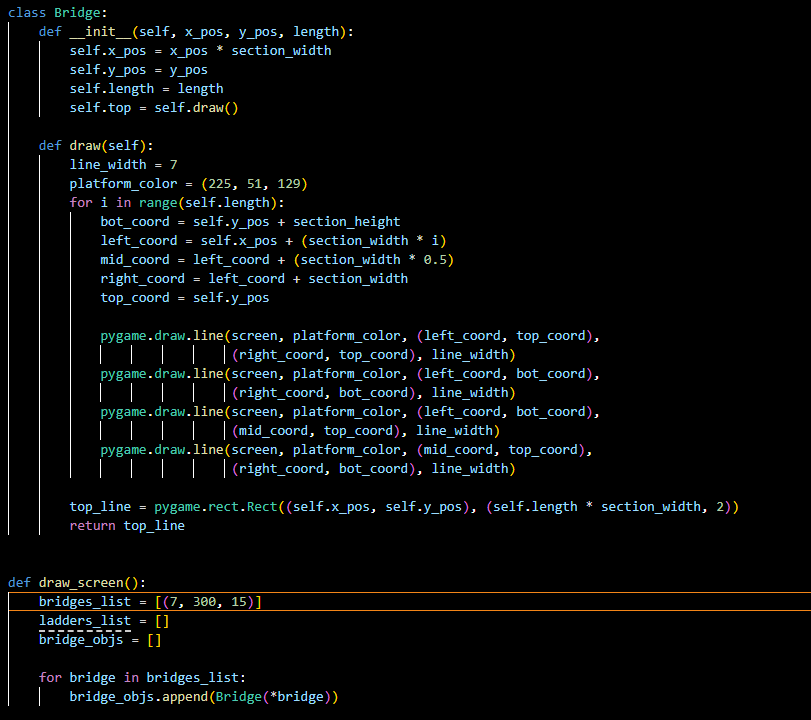
And there is an example that the x_coordinate is 7, y_coordinate is 300, and the length of the bridge is 15. And this is how it looks when you run it:
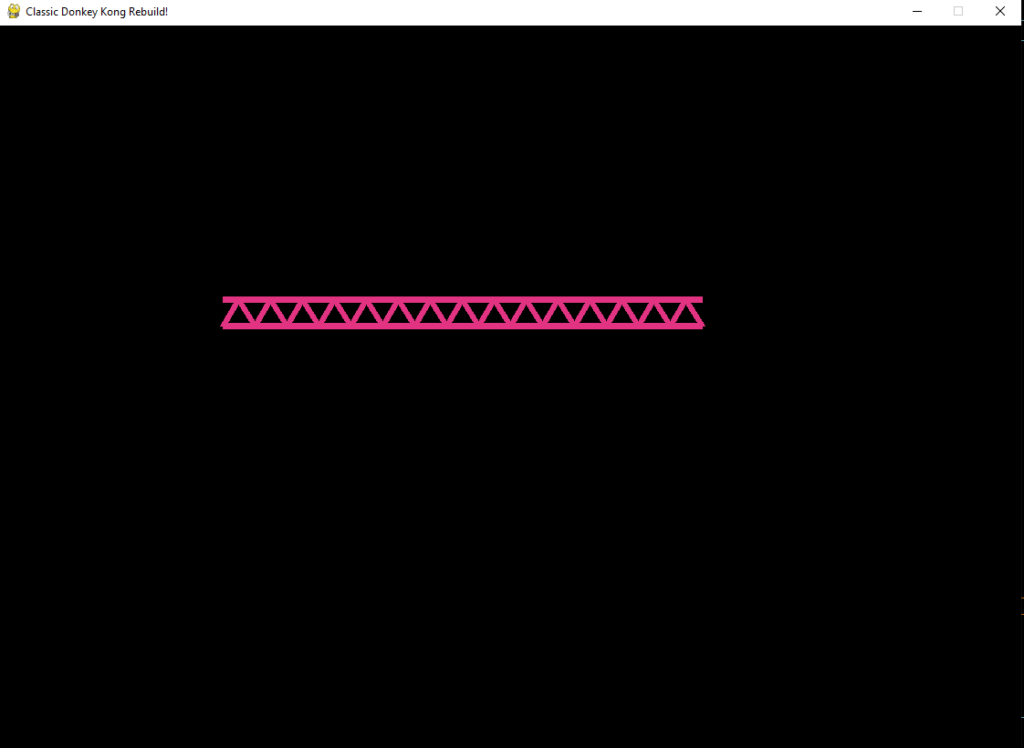
Setting Up A Level Dictionary With All Level Info
To draw the map of the game, we need to create level dictionaries to draw bridges and ladders. You can copy the list of bridges and ladders in LeMaster Tech’s Github. Just control + f and type levels, you will find the dictionaries that you need. And below is how the level dictionaries look and how it connects with the draw_screen() function to draw the bridges into the screen game.
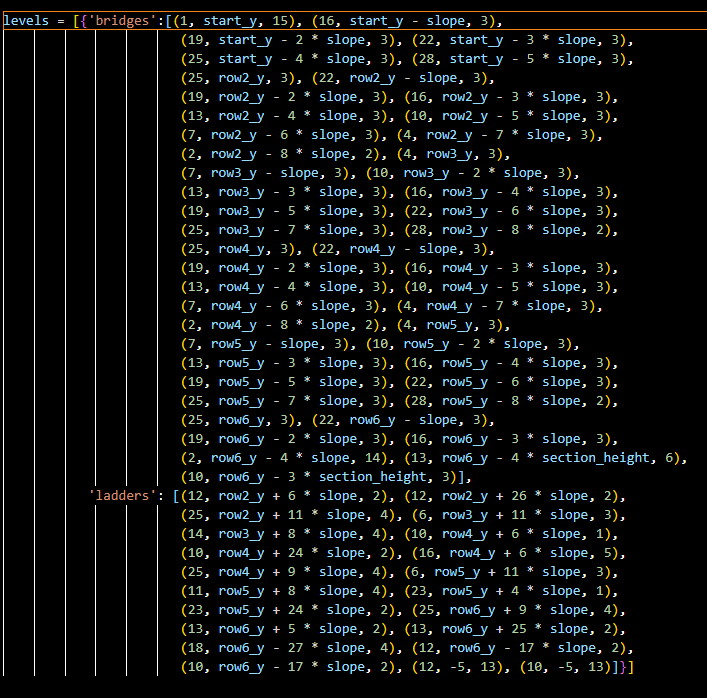
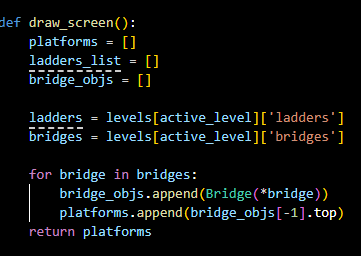
When you run it, you will receive the map of the game like below:
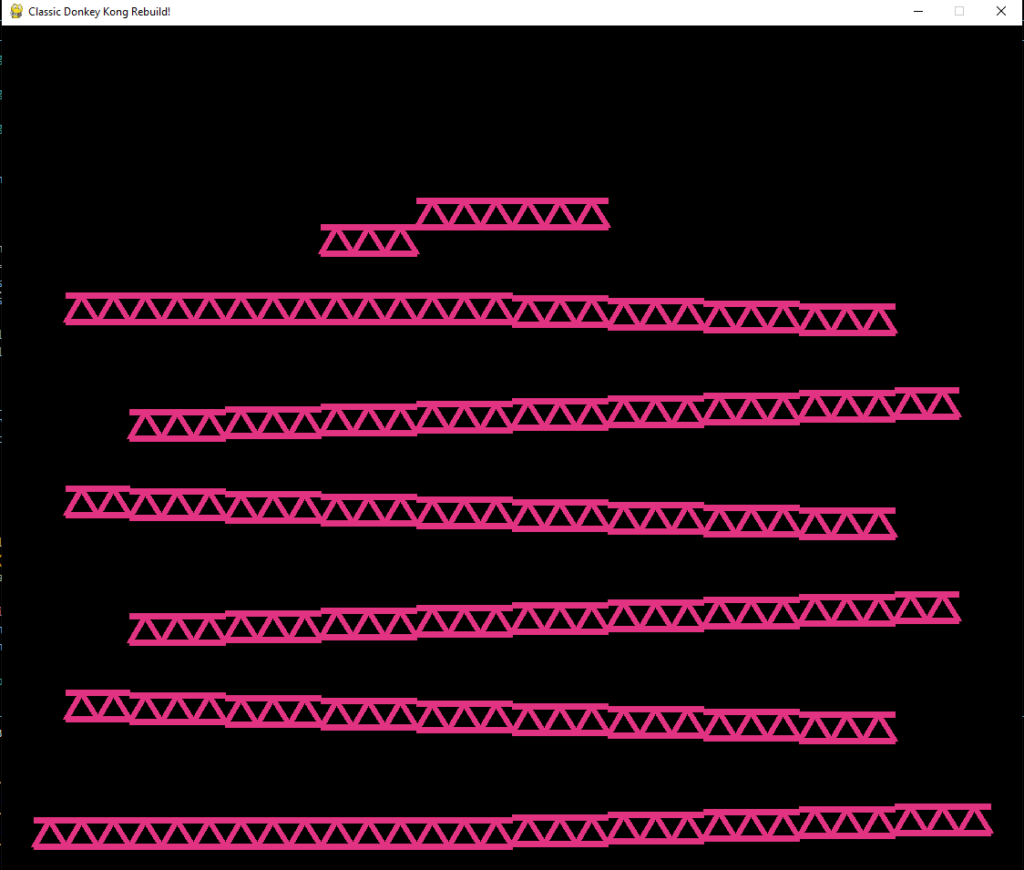
Setting Up A Class For Ladders
Next we create a Ladders class, this class needs 3 arguments (x_coordinate, y_coordinate, length) like the Bridges class. And using the ladders list in level dictionaries into the draw_screen() to draw the ladders into the screen.
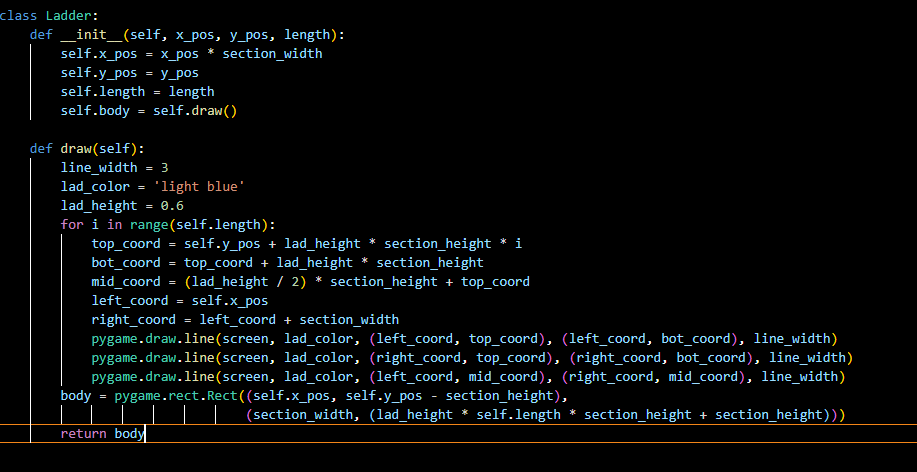
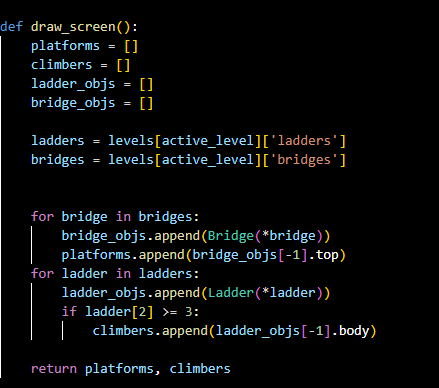
This is a classic Mario vs. Donkey Kong’s map.
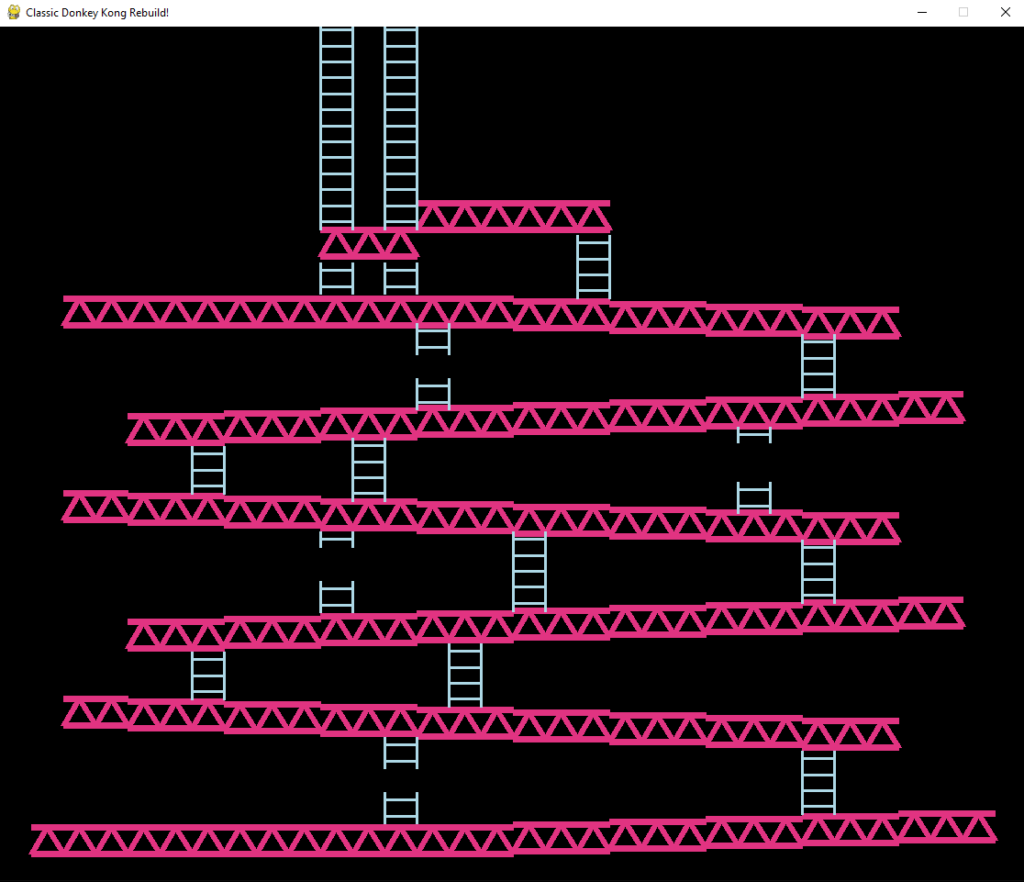
Setting Up A Pygame Sprite Group For Barrels
To have more challenges for Mario we draw the barrels into the window. We create a Barrel class and have update() and check_fall() function, we will need it later to make it move. For now, we load the barrel image and make it animate. To have the barrel image, you go to LeMaster Tech’s Github to download the images.

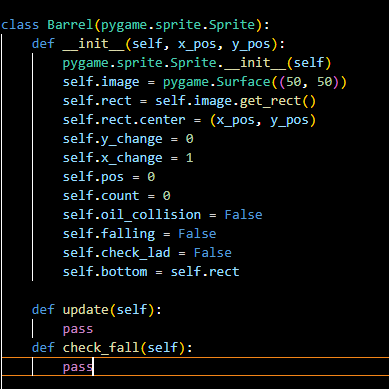
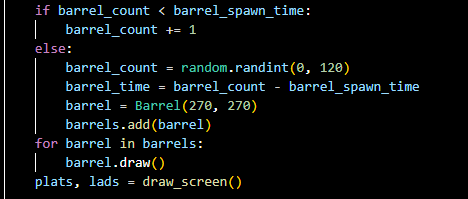
The barrel is drawn on the bridge on the screen game.
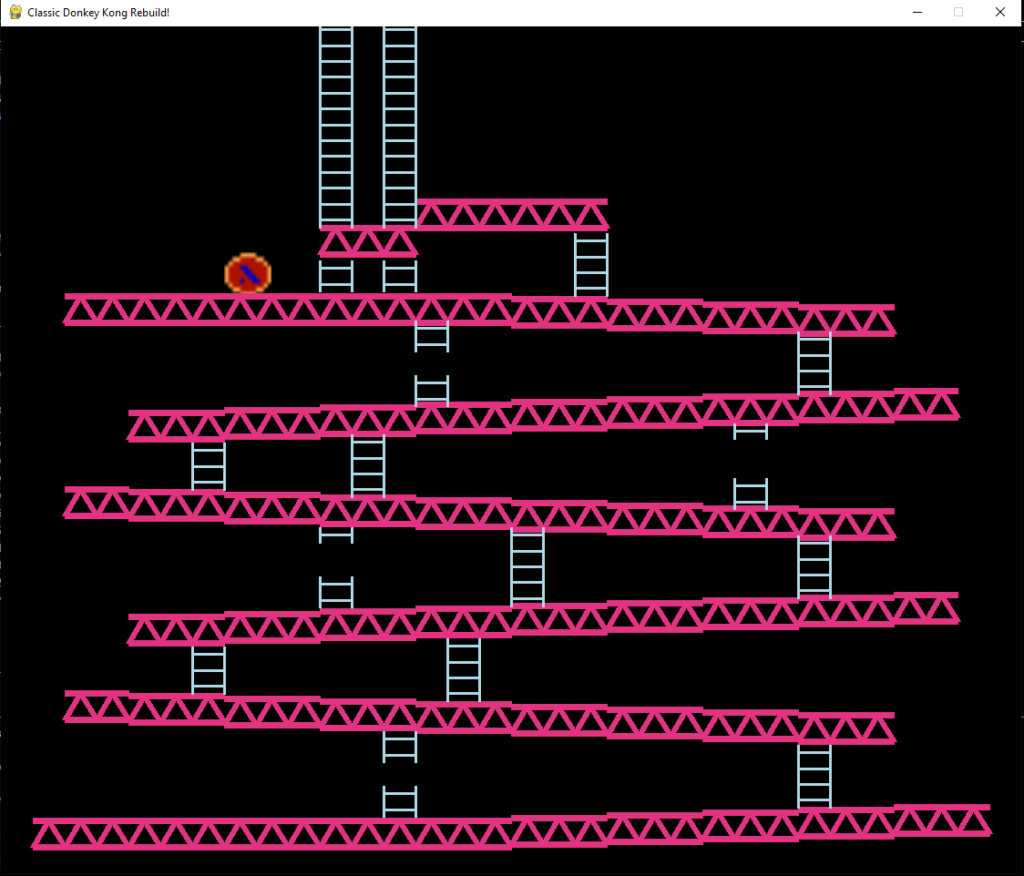
In the Barrel class, we have an update() and check_fall() function. The update() which is to update its position and check itself, that if one barrel falls down one bridge then it will create a new barrel. And the check_fall is checked does the barrel falls down one bridge or not.
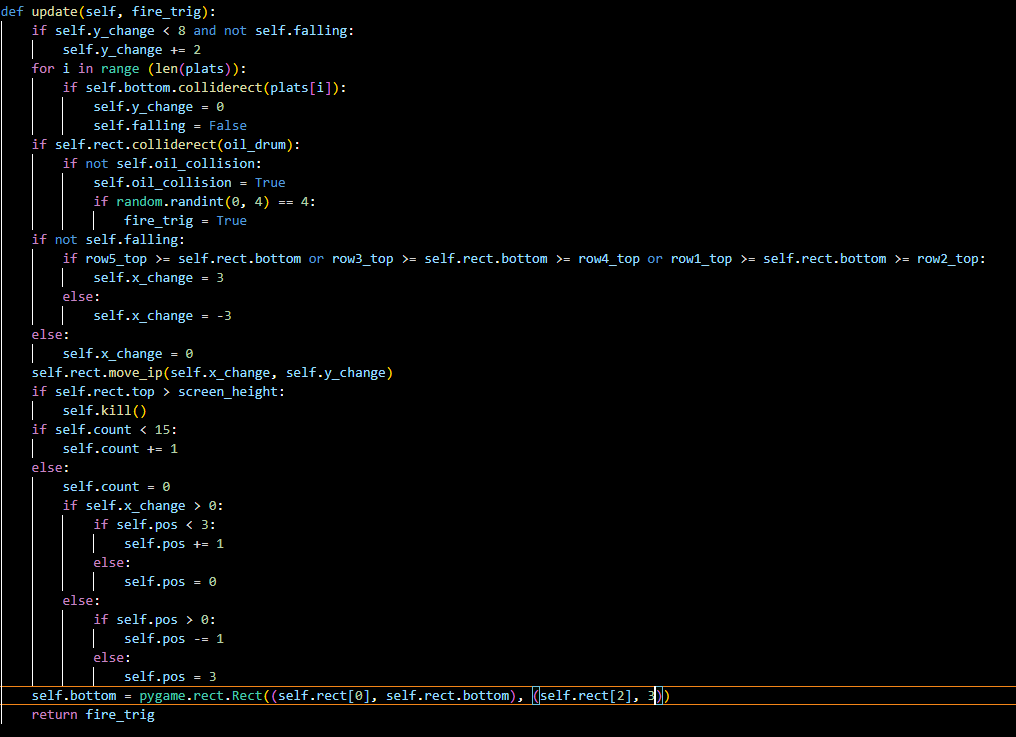
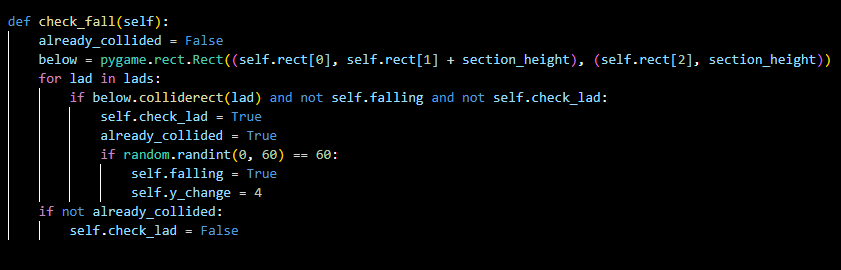
Below are the barrels that are animate and moving.
![]() | ![]() |
Drawing Oil Drum, Peach, And Donkey Kong On Screen
We create a draw_oil() function to draw the oil drum, and call it in the draw_extras() function. The draw_extras() is to draw the oil drum, barrel, Donkey Kong, and princess also.
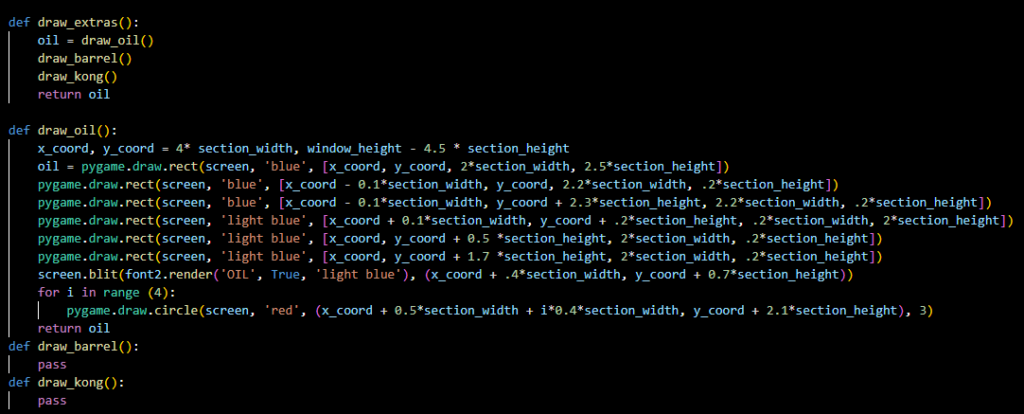
The oil drum is drawn on the screen.
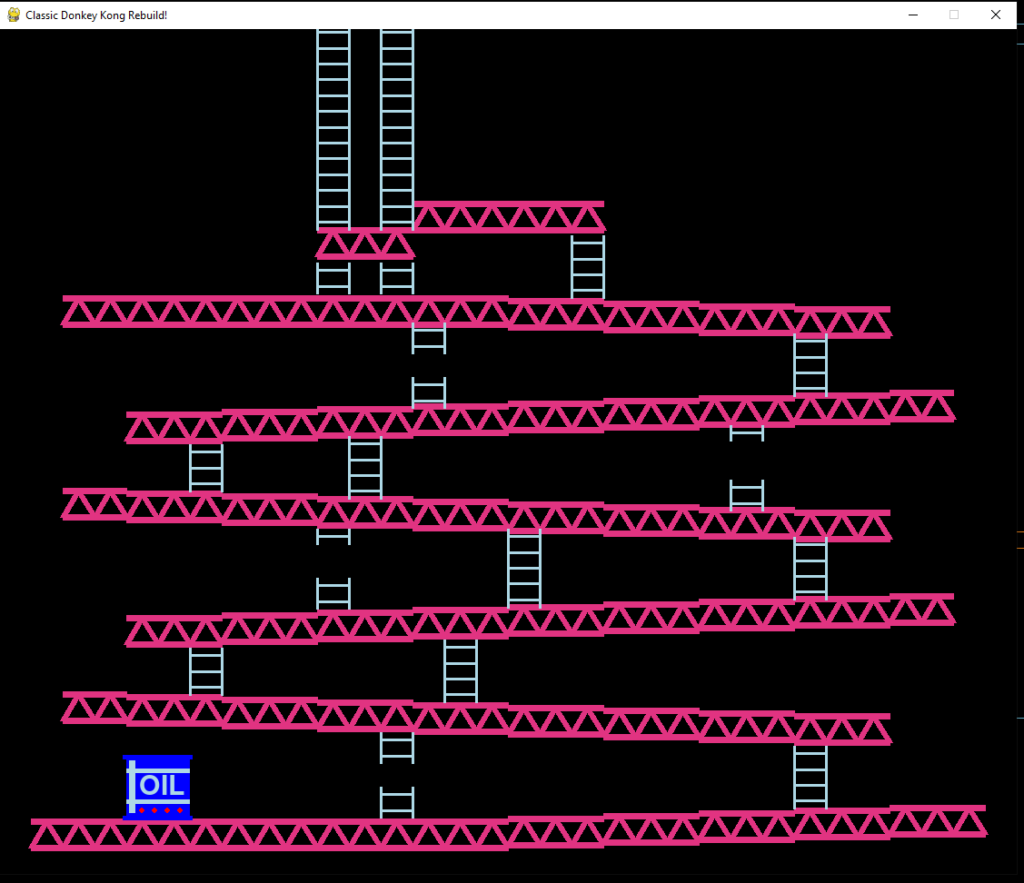
Next we load the fire image and create a counter variable equal to 0. And create a program is check if the counter is below 60, it adds to 1 and if the counter is above 60, the counter is 0. And in draw_oil() function, we add a if elif function, it checks if the counter below 15 or between 30 and 45 will draw the fire on top of the oil drum. And otherwise it will flip the fire.

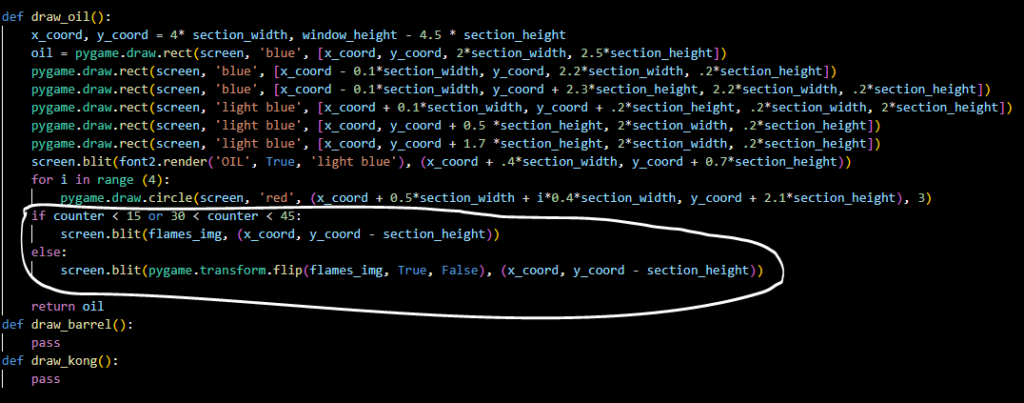
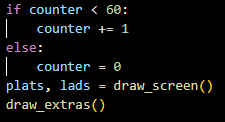
The fire on top of the oil drum is drawn and it animates.
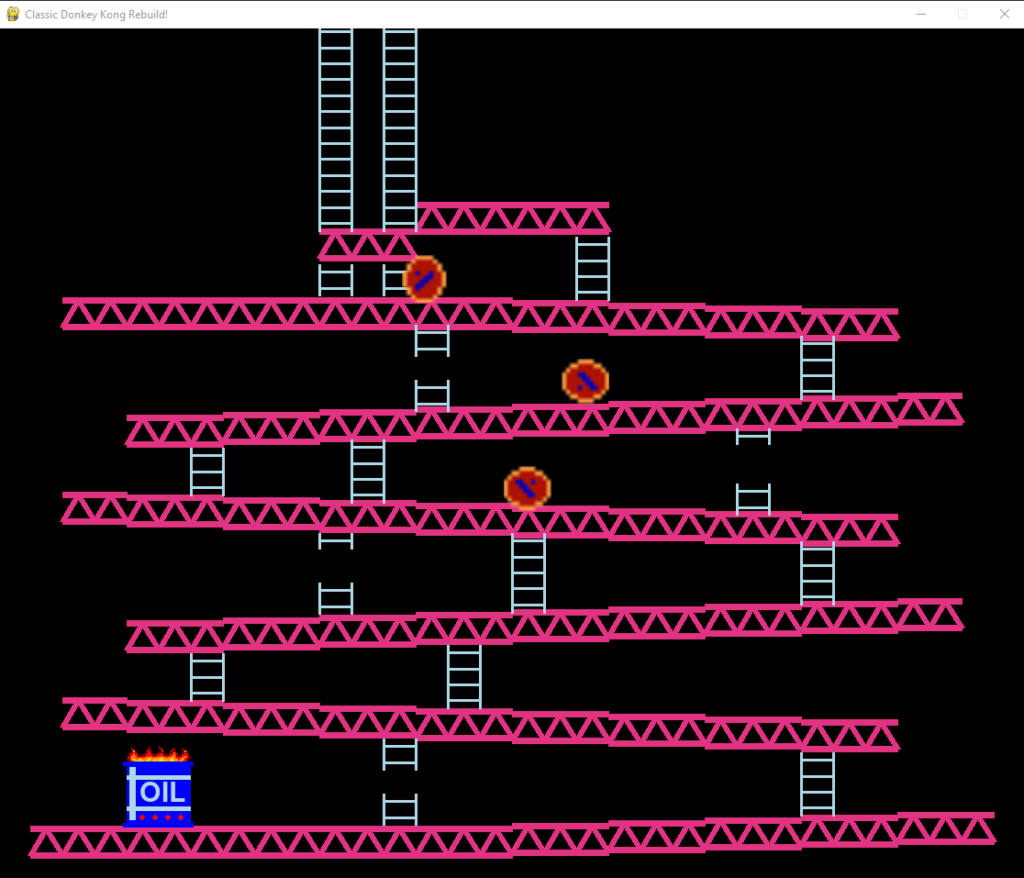
On the classic Mario vs. Donkey Kong has 4 barrels on the top left of the screen game. To have it we load the barrel side image and create a draw_barrel() function to use the blit() method to copy the image and rotate it. And call that function in the draw_extras() function to make the barrels appear on the screen game.


The barrels appear on the screen game.
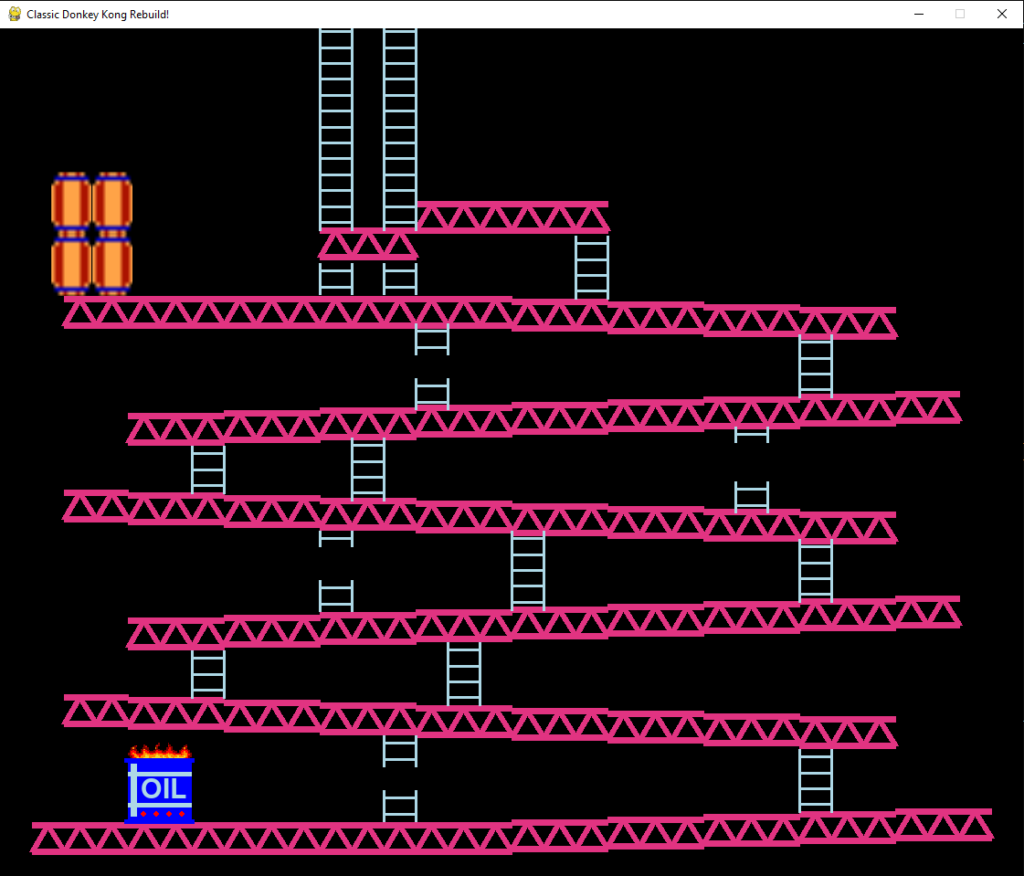
Next to draw the Donkey Kong, we load its images and create a draw_kong() function. In the draw_kong() function, it will use the blit() function to copy the images and make Donkey Kong animate.

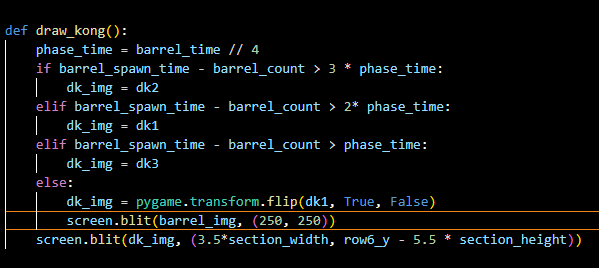
And the Donkey Kong appears on the screen game and it can turn right to throw the barrel and turn back forward. But it just turn right one time and does not turn right again.
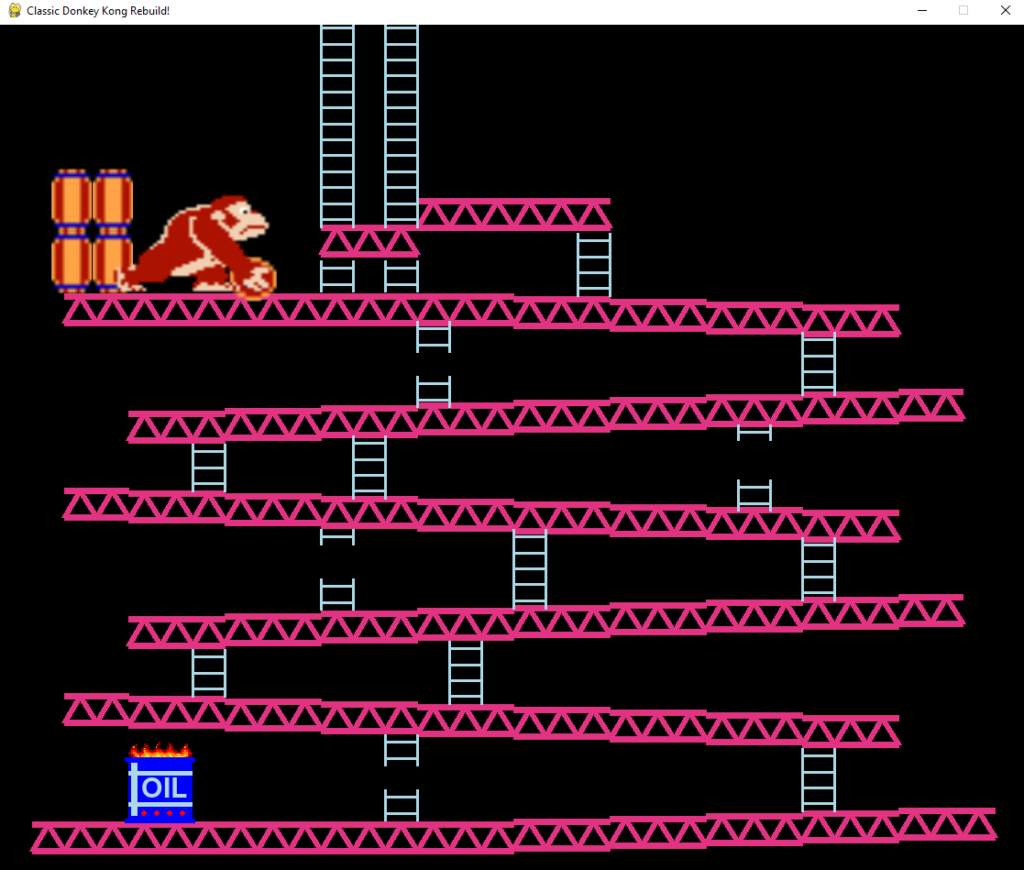
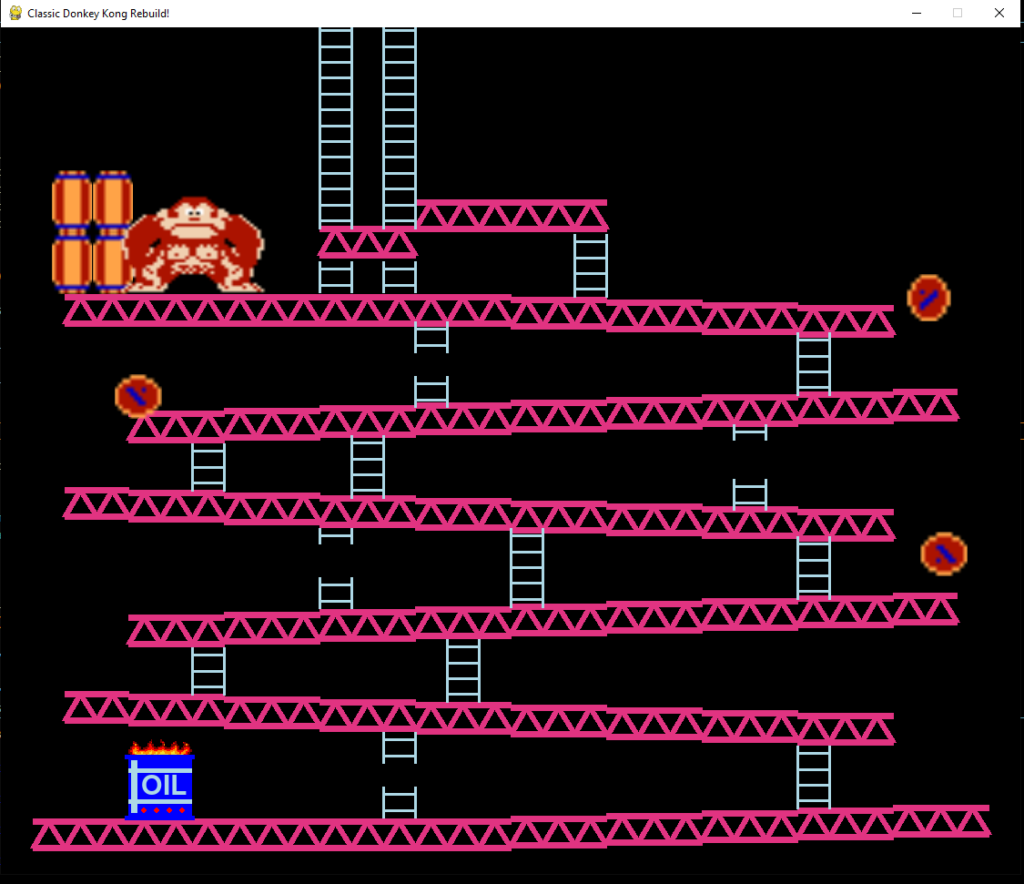
Now we fix the Donkey Kong to make it can turn left grab the barrel and turn right throw the barrel away.
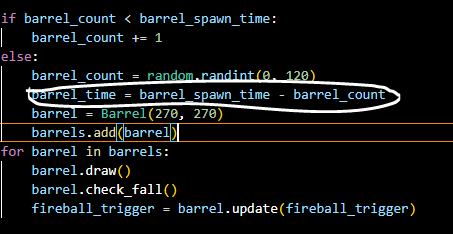
And this is how the Donkey Kong animates.
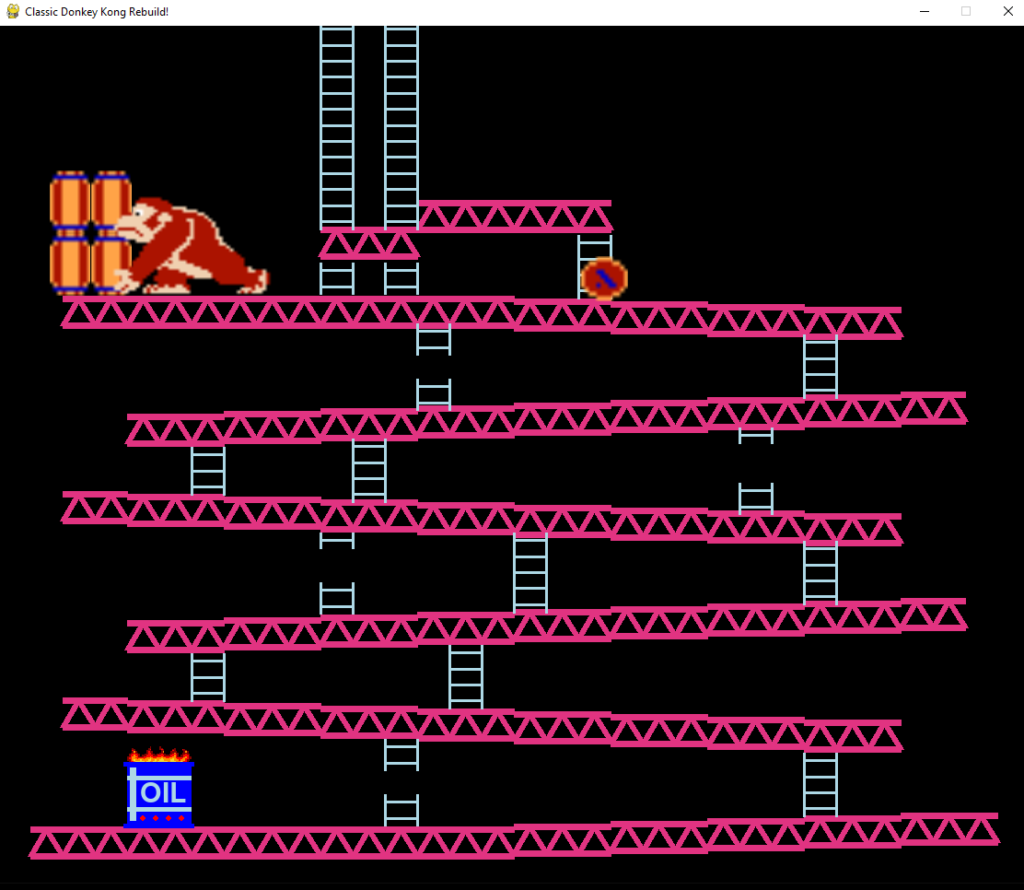
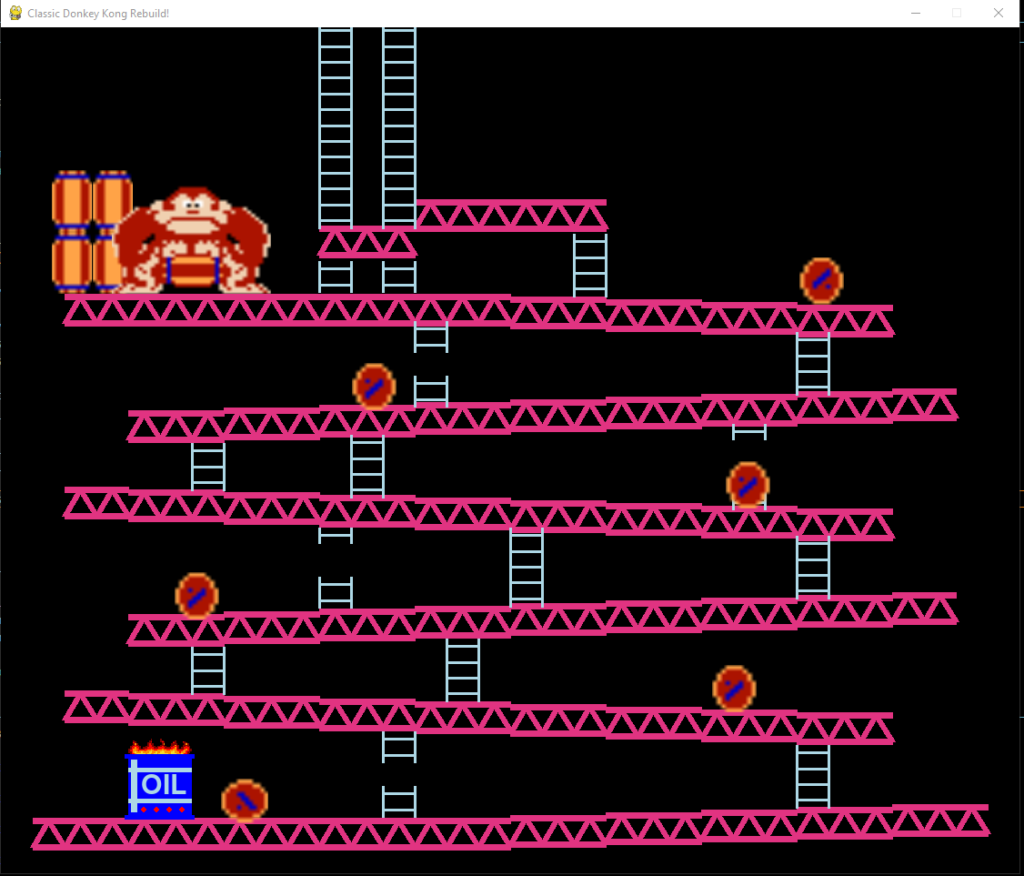
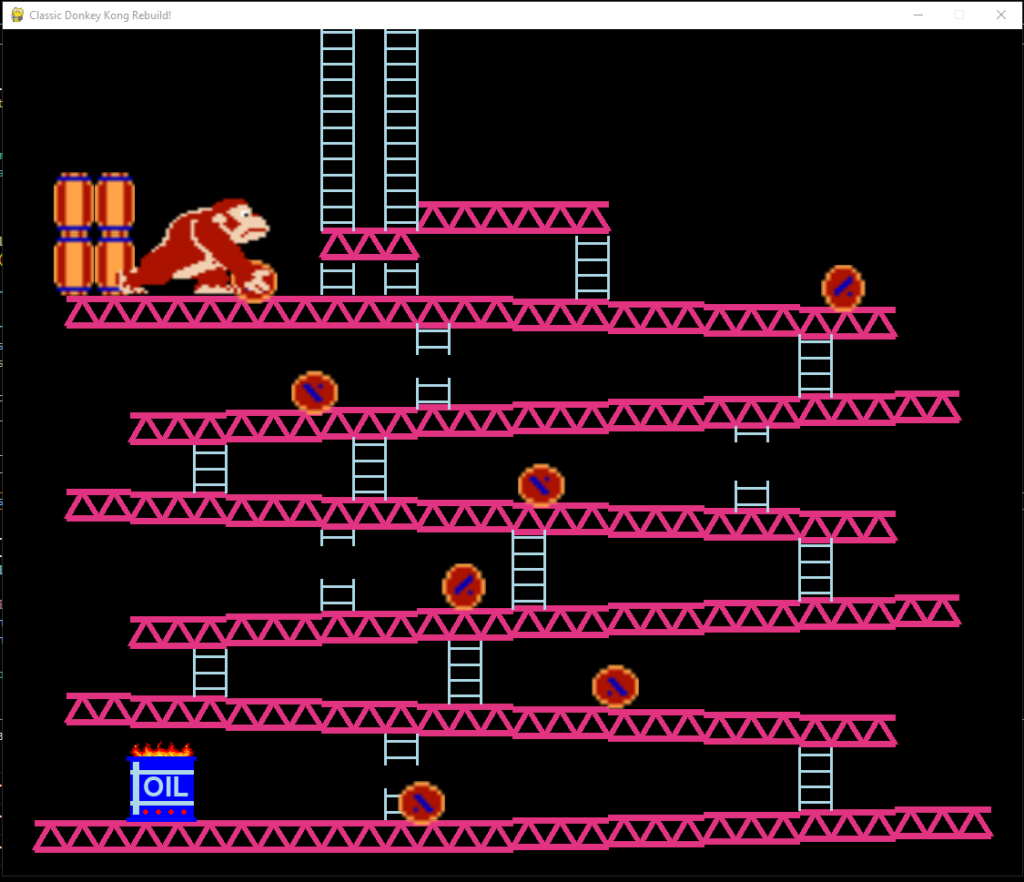
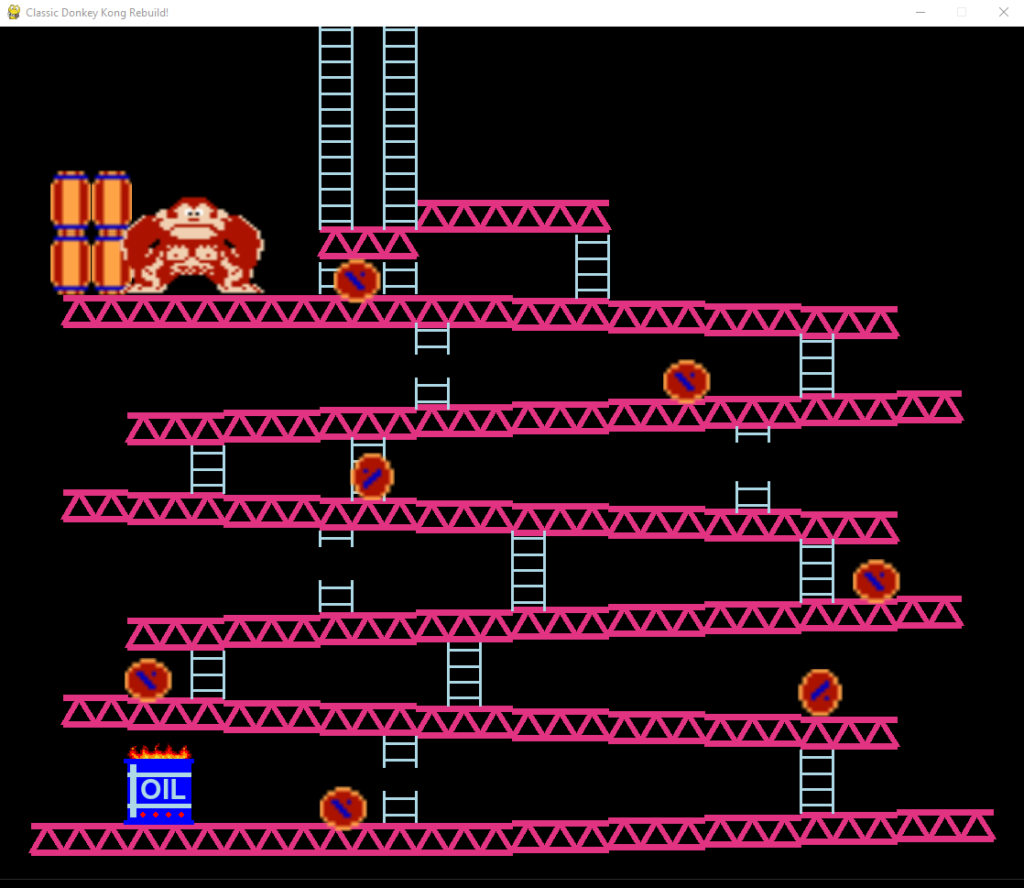
Finally we can draw the princess into the screen game. We still load its images and draw it in the draw_extras() function. The princess will appear on the screen game.

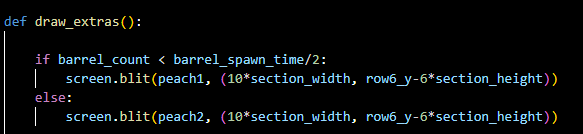
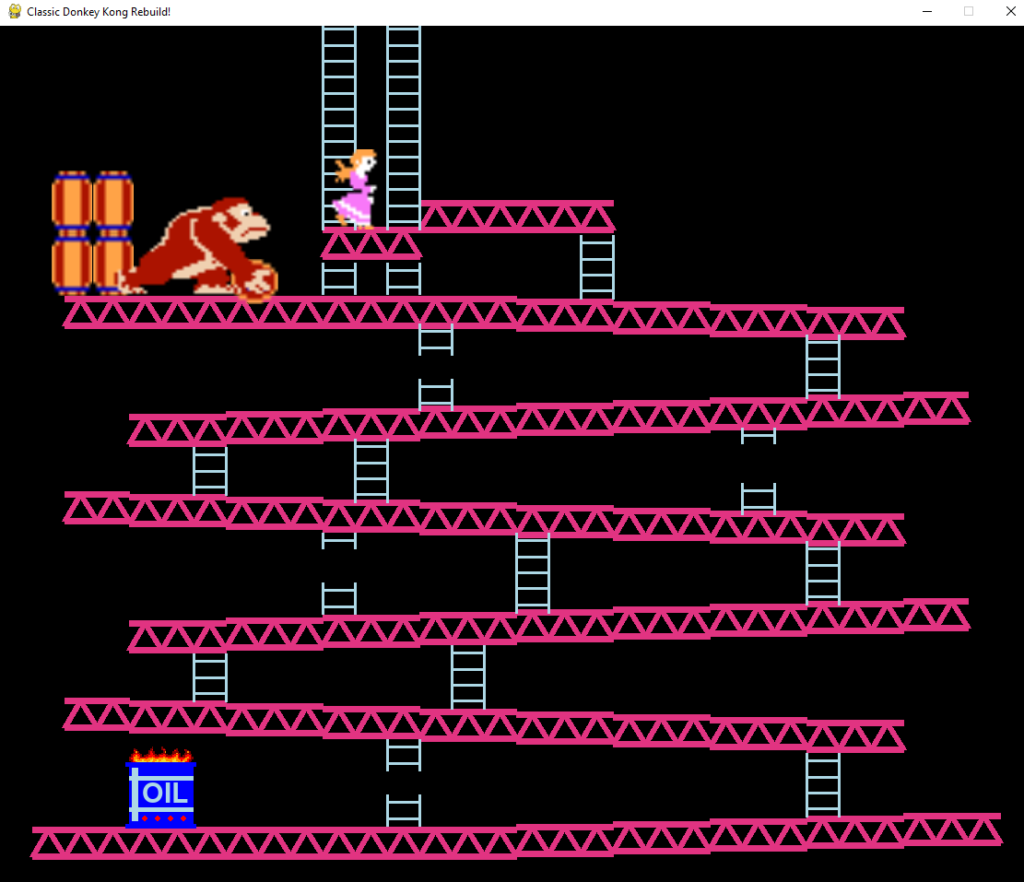
Setting Up A Pygame Sprite Group For Flames
To create a fireball and it can move forward and backward, we load the fireball image and create a Flame class. In Flame class, we have update() and check_climb() function. The update() in Flame class is familiar with the update() of the barrel. So with a barrel if it wasn’t touching the ground, we wanted it to touch the ground, so the update() in Flame class works the same as that. And the check_climb() is the same as the check_fall() of the Barrel class.

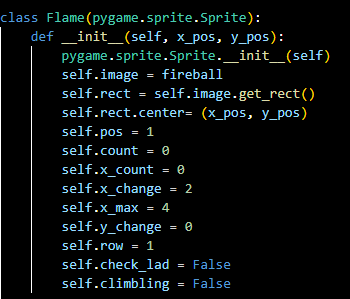
This is the update() function.
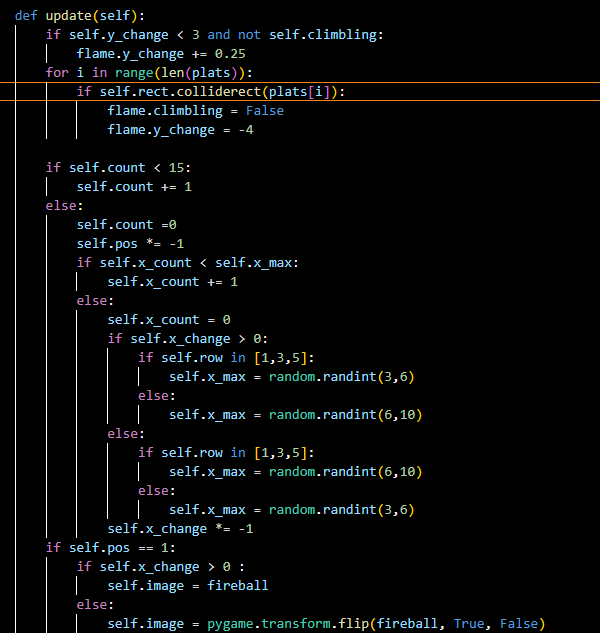
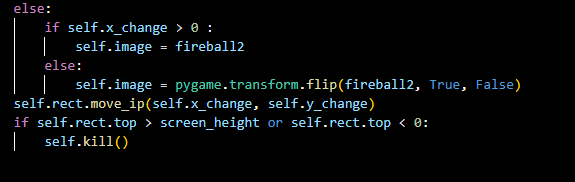
And this check_climb() function.
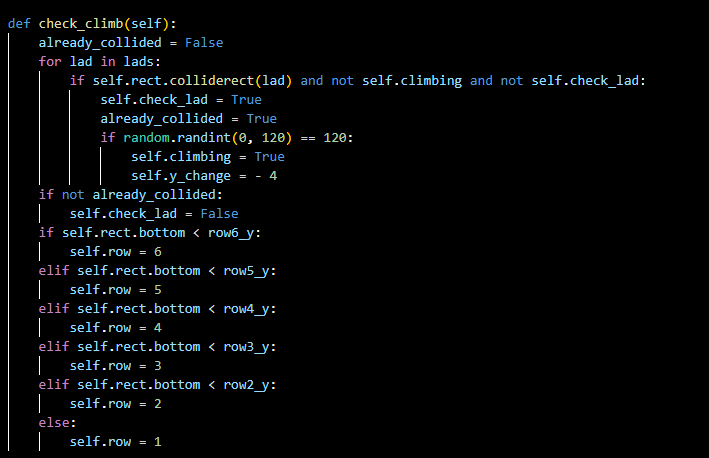
Next step, we call the Flame class and make the fireball animate.
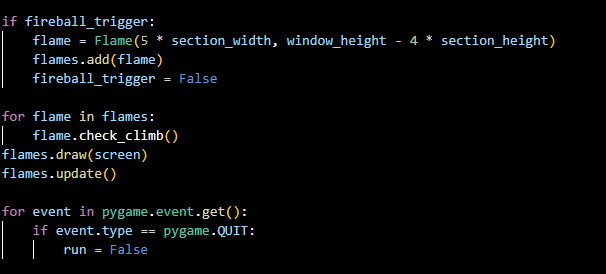
The image below is that a fireball appears on the bottom left of the screen and it moving forward and backward.
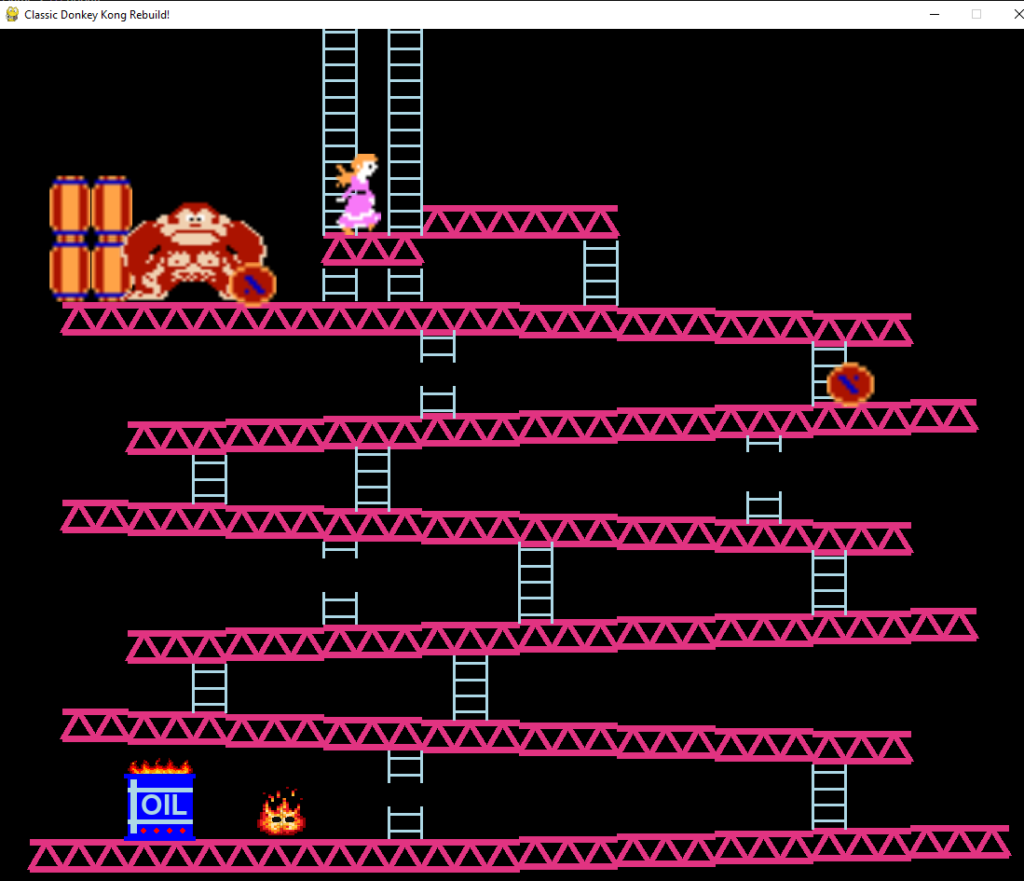
Setting Up The Player Sprite For Mario
First we need to load all the images that we need to make Mario appear on the screen game. Then, we create a Player class. Inside the Player class, we have update(), draw(), and calc_hitbox() function. The update() is to check the player position and make it move backward, forward, climb up and climb down the ladder. And also make how long the player can hold the hammer. In the draw() function, we draw the Mario image into the screen game; standing, jumping, running, etc. And in the calc_hitbox(), it is calculated what part of the player is colliding with a flame, a barrel, a bridge or a ladder.

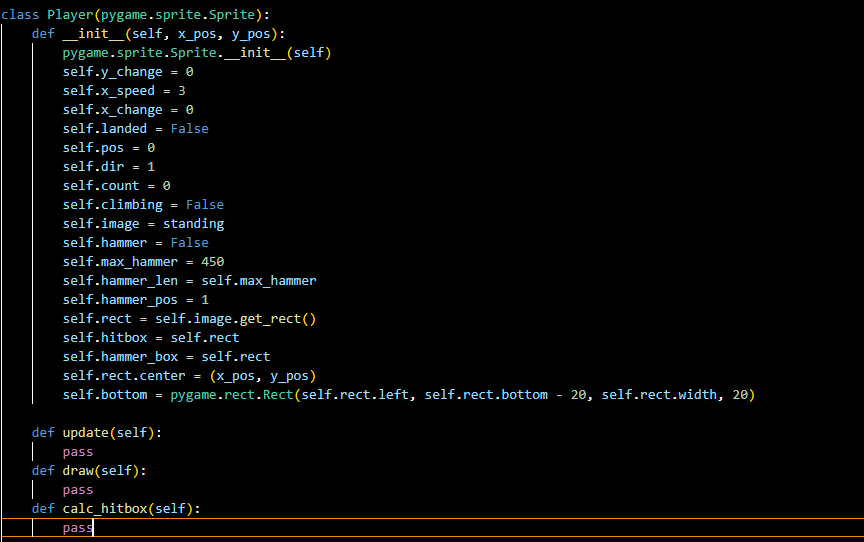
In the update() function, it checks if the player is landed and climbing, so we just change the y coordinate by adding 0.5. In def __init__ () we make the hammer_len equal max_hammer (max_hammer = 450), so in the update() function, we check if the player have hammer so we will make the hammer_len minus 1 until to 0 which means how long the player can hold the hammer. When the hammer_len equals 0 so we update the player not holding the hammer and make the hammer_len equal max_hammer again.
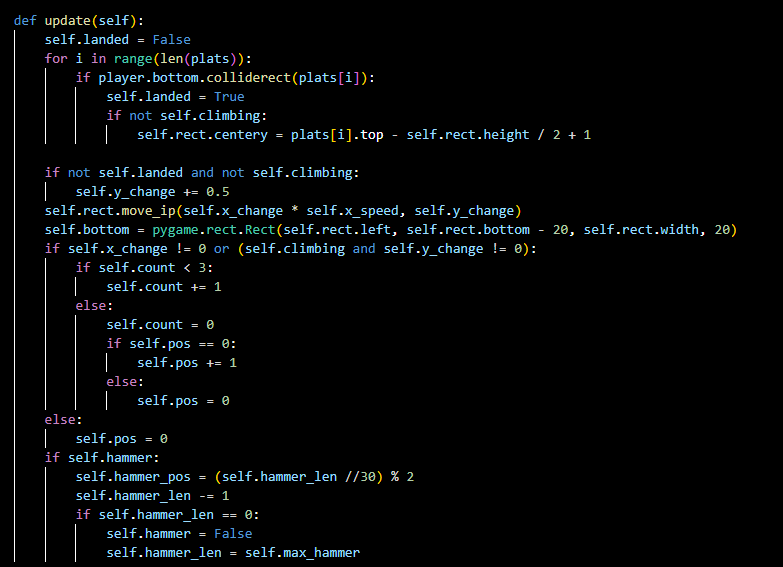
In the draw() function, it checks if the player is not climbing and landed so the image of the Mario is standing, otherwise it is running. If it has not landed and is not climbing so Mario is jumping.
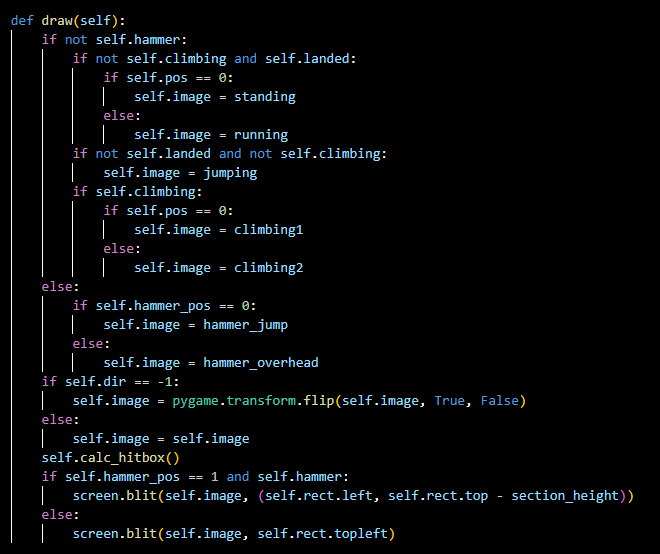
This is the calc_hitbox() function, which checks which part of the player colliding with other objects.
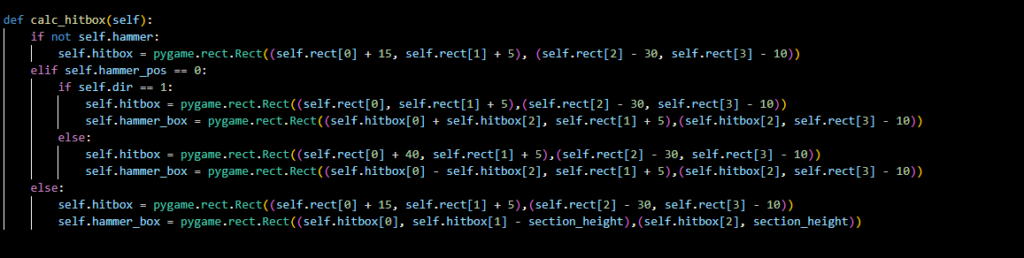
Then we call the Player class and call the update() and draw() function.
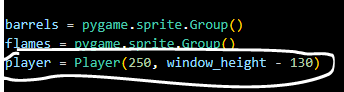
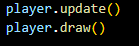
When you run the code, you will have Mario appear on the window. But it cannot move yet, we will make it moving to the next section.
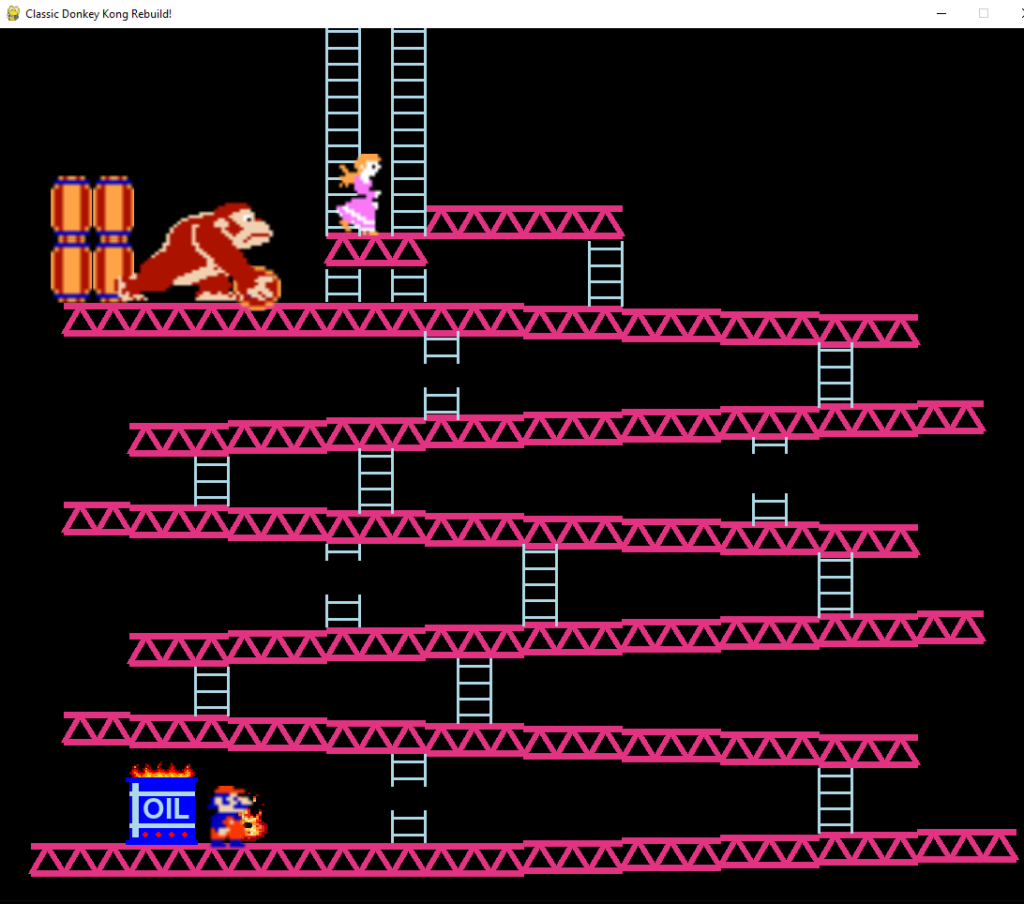
Setting Up Player Controls And Ladder Climbing
On this section, we set the left, right, up, down, and space keyboard to make Mario move, jumping, climbing. And make Mario only be able to climb unbroken ladders.
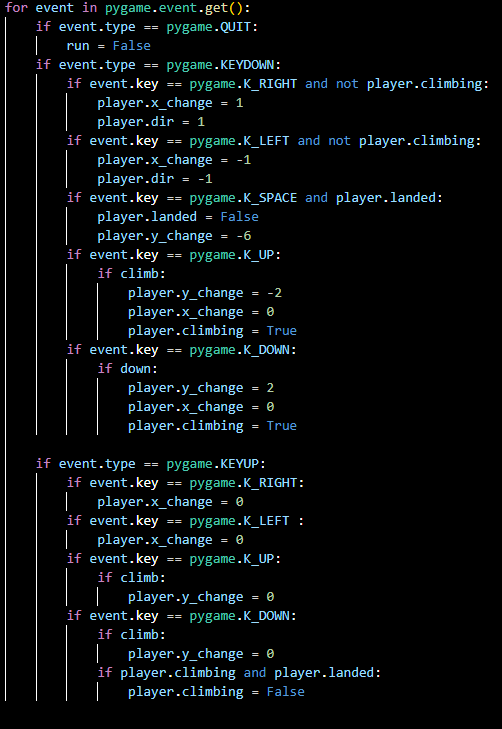
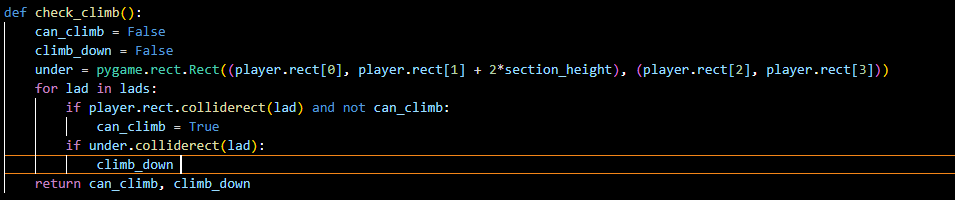
![]() | ![]() |
![]() | ![]() |
The image below is the problem we have with Mario. It is when Mario is climbing, it will climb over ladders and still climb up whether it does not have the ladder.
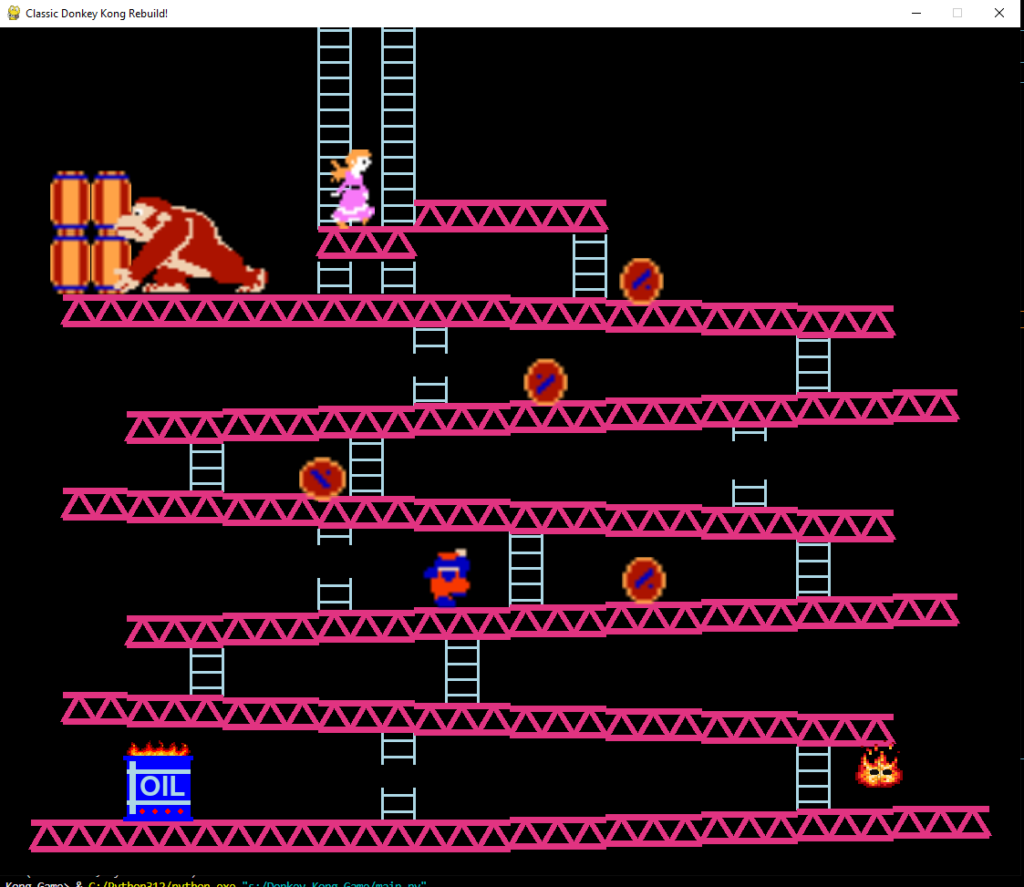
To fix this we need to add an if elif in the check_climb() function.
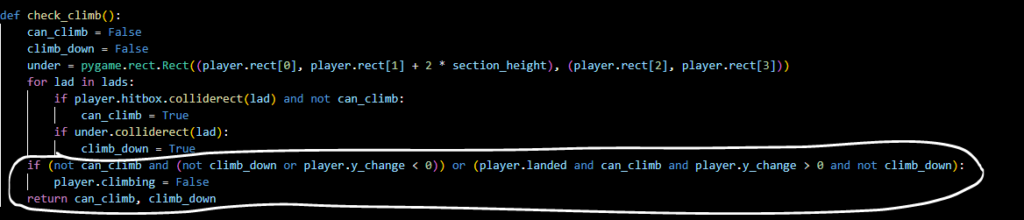
Now Mario can only climb up or down where there is a unbroken ladder.
![]() | ![]() |
Setting Up A Pygame Sprite Group For Hammers
To draw the hammer into the screen, we add 2 hammers position in level dictionaries. And create the Hammer class and draw() function. Create a check If the player does not use the hammer yet, it will draw the hammers. And also makes the player able to use hammers.
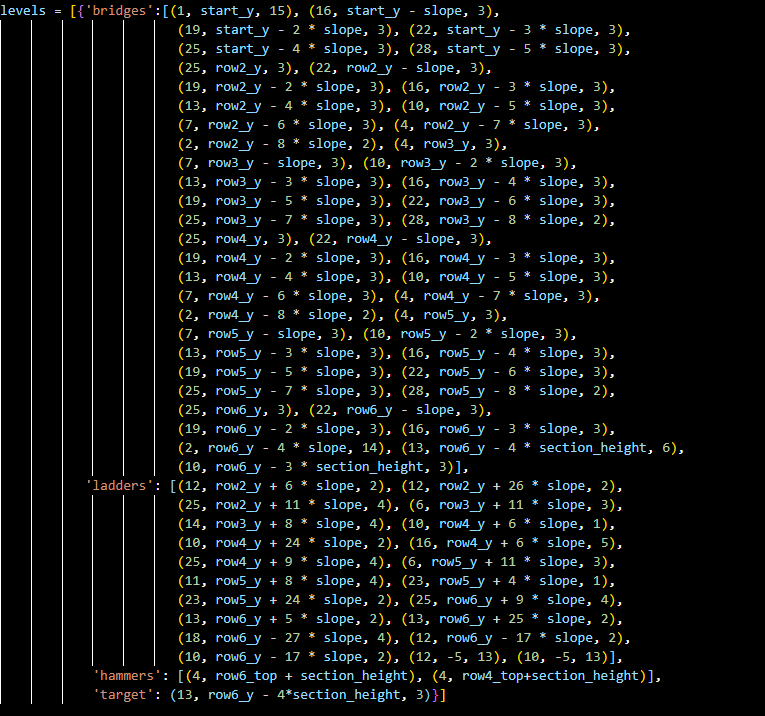
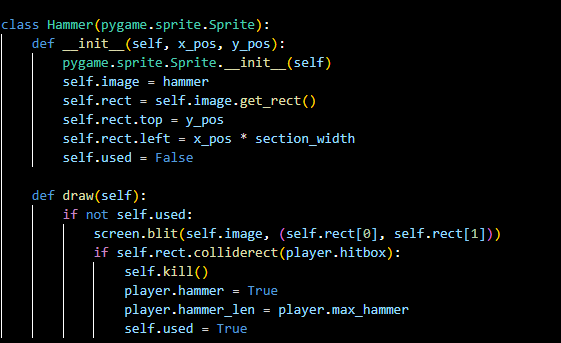
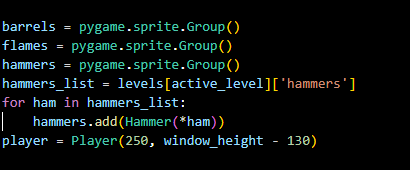
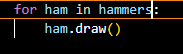
The hammer is drawn on the screen and Mario can take the hammer.
![]() | ![]() |
Smashing Barrels With Hammers,Drawing Scores, etc
Now we make an if elif method to check if the player holds the hammer and touches the barrel, it will make the barrel disappear. And when one barrel disappears we will add 500 points to the score.

![]() | ![]() |
Next we can draw scores, bonuses, and live into the screen game by using the blit() method in the draw_extras() function.
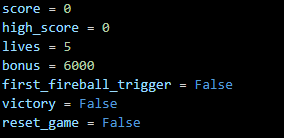
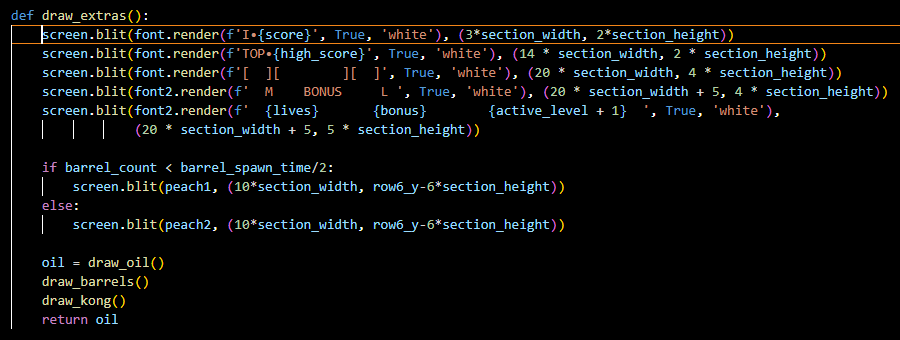
Top left is your score when you make a barrel disappear (add 500 for each barrel), M is your lives (5 lives), Bonus is the bonus point (6000), and the L is your active level.
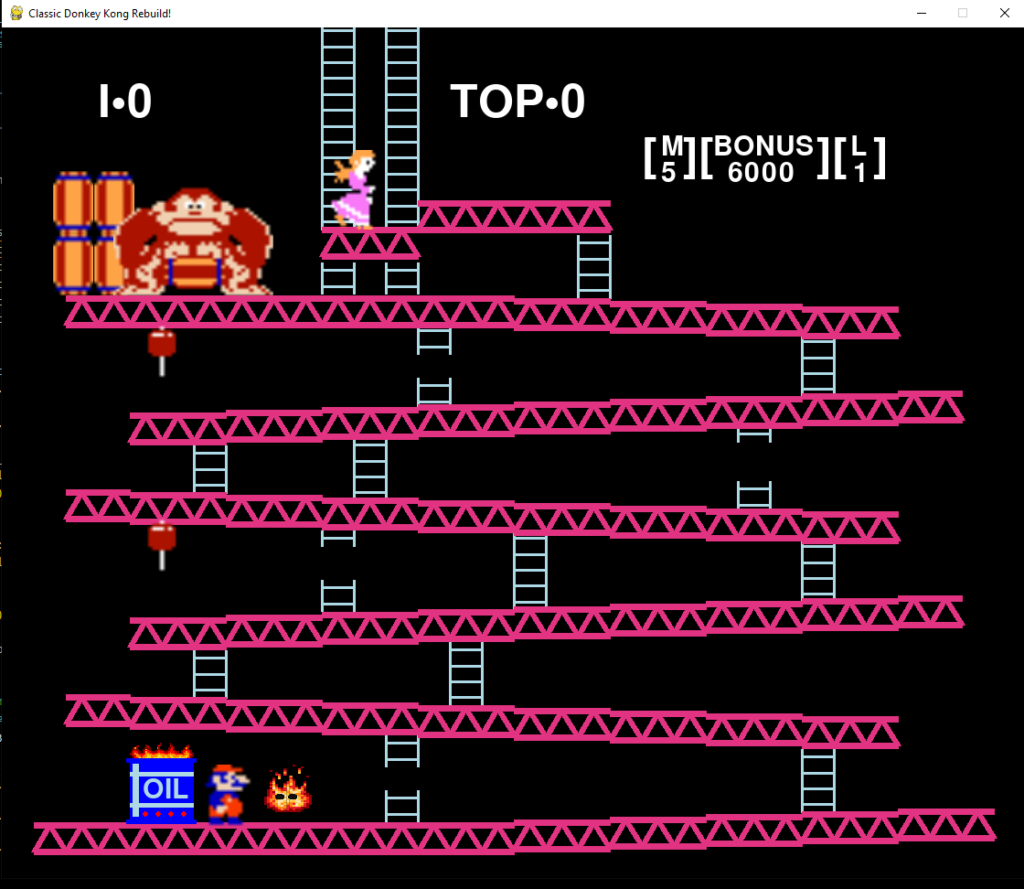
Check Player Collision With Barrels And Flames
Now we check if the player collides with the barrel, flames(fireball), it will return reset = true, and we will make the game reset in reset() function in the next section.
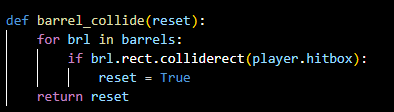
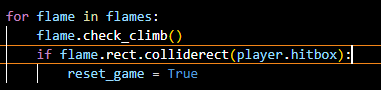
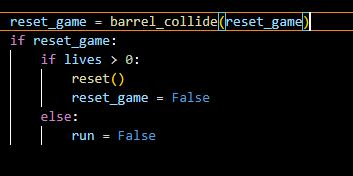
Resetting, Scoring, Winning!!!
We create a reset() function to reset the game when the player collides with a barrel. In game, we have 5 lives and minus 1 when the player collides with the barrel. And make a program check if the bonus points are larger than 0 it will be minus 100. And if the player go to the princess when the bonus points are not 0, we are winning the game !!!!
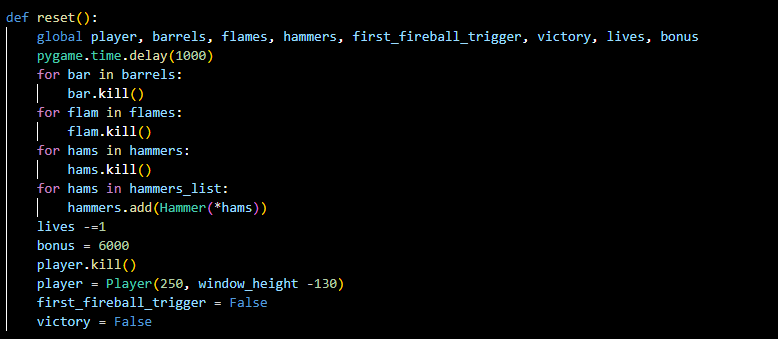

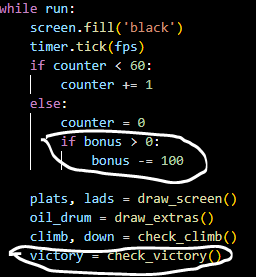
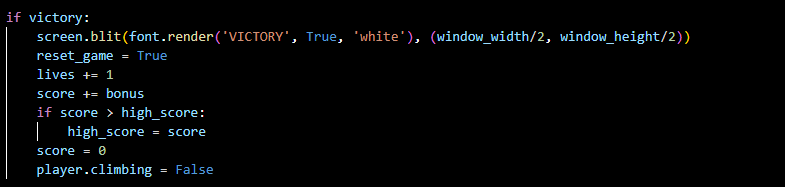
When you run code, the score, bonus points, and lives are working right now. And now you can play the game and try to save the princess to win the game.
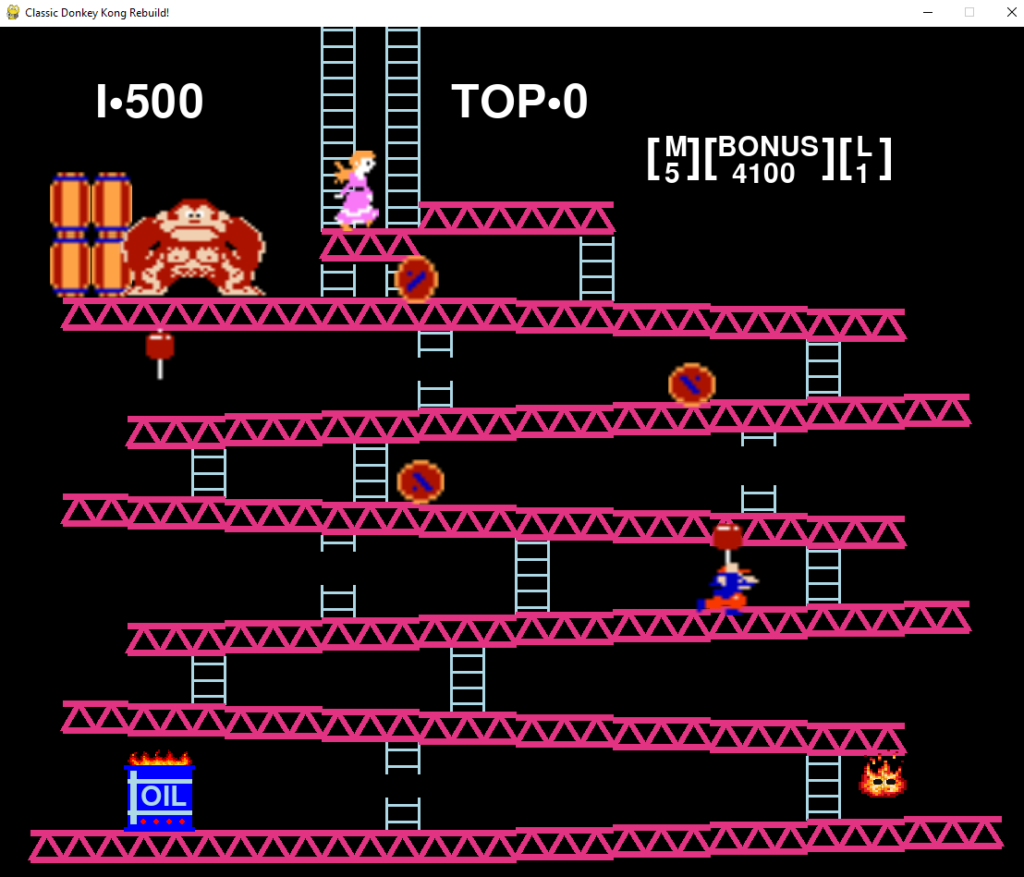
This is the tutorial from LeMaster Tech that I was followed to rebuild the Mario vs. Donkey Kong game.
No Responses